Building Better Apps: The Kotlin and Architecture Components Blueprint
In the dynamic world of Android app development, keeping up with the latest tools and best practices is essential. Kotlin has emerged as the preferred language for Android development, and Android Architecture Components provide a robust framework for building scalable apps. In this blog post, we will explore the best practices for leveraging Kotlin with Android Architecture Components to create high-quality, efficient, and maintainable Android applications.
Table of Contents
1. Why Choose Kotlin for Android Development
Kotlin, an expressive and concise programming language, has gained significant traction in the Android development community for several compelling reasons:
- Concise Syntax: Kotlin’s syntax is concise and expressive, reducing boilerplate code and making your codebase more readable and maintainable.
- Null Safety: Kotlin’s type system enforces null safety, reducing the chances of null pointer exceptions, a common source of crashes in Android apps.
- Interoperability: Kotlin seamlessly interoperates with Java, allowing developers to gradually migrate existing Java codebases to Kotlin.
2. Understanding Android Architecture Components
Android Architecture Components provide a set of libraries and guidelines to build robust and maintainable Android apps. Key components include:
- LiveData: LiveData is an observable data holder class that allows you to easily propagate changes in data to UI components. In Kotlin, you can create LiveData objects like this:
```kotlin val liveData = MutableLiveData<String>() ```
- ViewModel: ViewModel is responsible for managing UI-related data. It survives configuration changes and ensures that data is available to the UI components. Here’s how you can use it:
```kotlin class MyViewModel : ViewModel() { val data = MutableLiveData<String>() } ```
- Room Database: Room is a persistence library that provides an abstraction layer over SQLite. It simplifies database operations and allows you to define your database using Kotlin data classes and DAOs.
- Navigation Component: Navigation Component simplifies navigation in your app, providing a visual representation of navigation paths. It helps manage app navigation efficiently.
3. Best Practices for Using Kotlin with Android Architecture Components
- LiveData and ViewModel
– When using LiveData, observe data changes in your UI components using the `observe` extension function. This ensures that your UI stays up-to-date with the data changes.
```kotlin viewModel.data.observe(viewLifecycleOwner, Observer { newData -> // Update UI with newData }) ```
– Store UI-related data in ViewModel to ensure data survives configuration changes and is available to different UI components.
- Room Database
– Define your entities as Kotlin data classes, which are concise and easy to work with.
```kotlin @Entity data class User( @PrimaryKey val id: Int, val name: String ) ```
– Use Kotlin’s coroutines for asynchronous database operations, making your code more concise and readable.
```kotlin @Query("SELECT * FROM user WHERE id = :userId") suspend fun getUserById(userId: Int): User ```
- Navigation Component
– Create a single, centralized navigation graph that represents the entire app’s navigation flow. This simplifies navigation management and enhances the overall user experience.
– Define destination fragments and actions in XML to visualize and configure navigation paths.
4. Sample Code Snippets
Here are some sample code snippets illustrating the best practices discussed:
- LiveData and ViewModel:
```kotlin val viewModel = ViewModelProvider(this).get(MyViewModel::class.java) viewModel.data.observe(viewLifecycleOwner, Observer { newData -> // Update UI with newData }) ```
- Room Database:
```kotlin @Entity data class User( @PrimaryKey val id: Int, val name: String ) @Dao interface UserDao { @Query("SELECT * FROM user WHERE id = :userId") suspend fun getUserById(userId: Int): User } ```
- Navigation Component (XML):
```xml <fragment android:id="@+id/firstFragment" android:name="com.example.FirstFragment" android:label="First Fragment" > <action android:id="@+id/action_first_to_second" app:destination="@id/secondFragment" /> </fragment> ```
5. Testing Kotlin Code in Android
To ensure the reliability and quality of your Kotlin code, consider these testing strategies:
- Unit Testing: Use JUnit for writing unit tests for your Kotlin code. Isolate components and test their functionality in isolation.
- UI Testing: Utilize Espresso or other UI testing frameworks to create automated tests that simulate user interactions and validate your app’s behavior.
6. External Resources
For further learning and reference, here are some external resources:
- Official Kotlin Documentation – https://kotlinlang.org/docs/home.html: Kotlin’s official documentation provides comprehensive insights into the language.
- Android Developer Documentation – https://developer.android.com/: The official Android developer documentation is a valuable resource for all things Android development.
- Android Architecture Components Guide – https://developer.android.com/jetpack/guide: Explore the official guide to Android Architecture Components for in-depth information.
Conclusion
Incorporating Kotlin with Android Architecture Components empowers developers to create high-quality Android applications efficiently. By following the best practices outlined in this blog post, you can leverage the strengths of Kotlin and the stability of Architecture Components to build robust, maintainable, and user-friendly Android apps.
With Kotlin’s concise syntax and Android Architecture Components’ structure, you’ll be well-equipped to outperform the competition in the ever-evolving world of Android app development.
Table of Contents
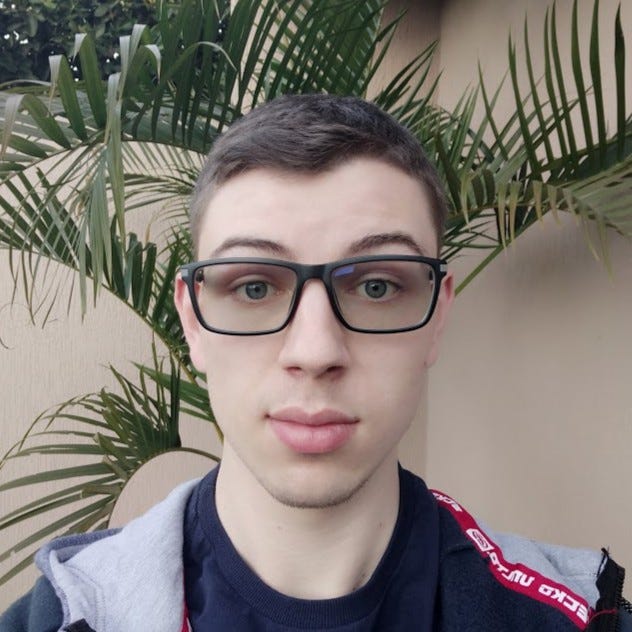
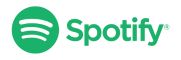