From Static to Dynamic: Harnessing Real-Time Data Sync with Kotlin & Firestore
In the fast-paced world of mobile app development, staying up-to-date with the latest technologies is essential. One such powerful combination for building real-time apps is Kotlin and Firebase Cloud Firestore. In this blog post, we’ll delve into the intricacies of using Kotlin with Firebase Cloud Firestore to create applications that synchronize data in real-time. Let’s dive in!
Table of Contents
1. What is Kotlin?
Kotlin is a modern, statically-typed programming language developed by JetBrains. It’s known for its conciseness, safety features, and seamless interoperability with Java. Many developers have embraced Kotlin for Android app development due to its simplicity and robustness.
2. Introducing Firebase Cloud Firestore
Firebase Cloud Firestore is a NoSQL, cloud-hosted database provided by Google as part of the Firebase suite of services. It’s designed to store and sync data across multiple clients in real-time, making it an ideal choice for building responsive and collaborative applications.
3. Getting Started
Before we dive into coding, make sure you’ve set up a Firebase project and added your Android app to it. You’ll also need to add the Firebase SDK to your project. Firebase provides comprehensive documentation on these steps.
4. Creating a Firestore Database
Once your Firebase project is set up, create a Firestore database. Firestore uses a document-oriented data model, where data is stored in collections, and each collection contains documents. Documents, in turn, can contain fields with various data types.
5. Real-Time Data Sync with Firestore
One of the standout features of Firestore is its real-time data synchronization. When you make changes to data in the database, the updates are immediately reflected on all connected clients. Let’s look at some code examples in Kotlin to demonstrate this feature.
```kotlin // Create a reference to the Firestore database val db = FirebaseFirestore.getInstance() // Reference to a specific document val docRef = db.collection("cities").document("SF") // Listen for changes in real-time docRef.addSnapshotListener { documentSnapshot, e -> if (e != null) { // Handle errors here return@addSnapshotListener } if (documentSnapshot != null && documentSnapshot.exists()) { // Data is available here val data = documentSnapshot.data // Handle the data } } ```
In this example, we create a reference to the Firestore database and then a reference to a specific document within a collection. The `addSnapshotListener` method allows us to listen for changes to that document in real-time. Any updates to the data will trigger the callback, giving us the latest information.
6. Examples of Real-Time Use Cases
Now that you understand how real-time data sync works, let’s explore some practical use cases for this powerful feature:
- Chat Applications: Build chat applications where messages are instantly delivered to all participants. Users can see new messages as soon as they are sent.
- Collaborative Editing: Create collaborative document editing apps, similar to Google Docs. Multiple users can work on the same document simultaneously, with changes reflected instantly.
- Live Feeds: Develop social media platforms or news apps where new posts or updates are immediately visible to users as they are published.
- Online Gaming: Design multiplayer games where game states are synchronized in real-time across all players, ensuring a seamless and responsive gaming experience.
Further Reading:
To further enhance your understanding of Kotlin and Firebase Cloud Firestore, here are some external resources you can explore:
- Kotlin Official Documentation – https://kotlinlang.org/docs/home.html : Dive deep into Kotlin with the official documentation, offering in-depth explanations and code examples.
- Firebase Documentation – https://firebase.google.com/docs : Explore Firebase’s official documentation to learn more about Firestore and other Firebase services.
- GitHub Repository with Kotlin Firestore Examples – https://github.com/firebase/snippets-kotlin/tree/master/firestore : Find Kotlin code snippets for Firestore on Firebase’s official GitHub repository.
Conclusion
Kotlin and Firebase Cloud Firestore provide a dynamic duo for creating real-time data synchronization in your mobile applications. With Kotlin’s expressive syntax and Firestore’s real-time capabilities, you can build interactive and responsive apps that captivate your users.
Table of Contents
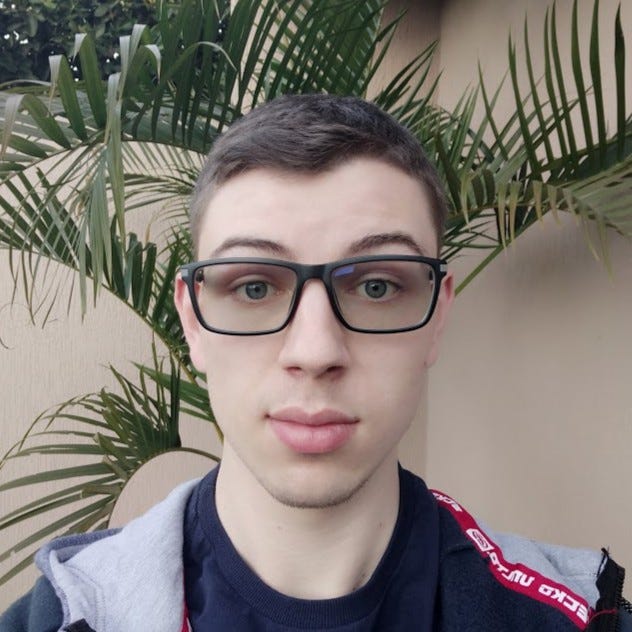
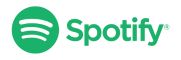