Creating Android User Interfaces with Kotlin: An Introduction to XML Layouts
When it comes to developing Android apps, the user interface (UI) plays a crucial role in delivering an exceptional user experience. With Kotlin and XML layouts, developers have powerful tools at their disposal to create visually appealing and interactive interfaces. In this blog post, we will dive into the world of Android UI design and explore the fundamentals of XML layouts in conjunction with Kotlin. By the end, you’ll have a solid foundation for building engaging user interfaces that captivate your app’s users.
1. The Importance of User Interfaces in Android Apps
The success of an Android app greatly depends on its user interface. A well-designed UI enhances user engagement, improves usability, and creates a positive impression of your app. Android UIs are primarily built using XML layouts in conjunction with Kotlin. XML layouts provide a declarative way to define the structure, appearance, and behavior of UI elements.
2. Introduction to XML Layouts
2.1. XML Layout Basics
XML stands for eXtensible Markup Language, and it is a markup language that defines hierarchical data structures. In Android, XML layouts are used to define the structure and appearance of UI elements. XML provides a clear separation between the UI and the app’s logic, making it easier to maintain and modify the UI independently.
2.2. Declaring UI Elements
In an XML layout file, UI elements are declared as tags. Each UI element has attributes that define its properties such as size, position, text, and appearance. For example, the following XML snippet declares a TextView element:
xml <TextView android:id="@+id/myTextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello, World!" />
2.3. Positioning UI Elements
XML layouts utilize various layout containers to position UI elements on the screen. The most commonly used containers are LinearLayout, RelativeLayout, and ConstraintLayout. These containers allow you to define rules and constraints to position UI elements relative to each other or the parent container.
2.4. Styling and Theming
Android provides a powerful styling and theming system that allows you to customize the appearance of your UI elements. Styles define a set of attributes that can be applied to multiple UI elements, while themes specify the overall visual style of the app. By utilizing styles and themes, you can achieve consistency and a polished look across your app’s UI.
3. Building an XML Layout
3.1. Creating a New XML Layout File
To create a new XML layout file in Android Studio, right-click on the “res” directory, select “New” > “Android Resource File,” and choose “Layout” as the resource type. Give the layout file a meaningful name and click “OK.” Android Studio will generate an XML file with the specified name and open it for editing.
3.2. Designing the UI Structure
Before adding UI elements, it’s essential to plan the structure of your UI. Identify the different components and their hierarchy, keeping in mind the user flow and interaction patterns. Once you have a clear understanding of the UI structure, you can proceed to add the necessary layout containers and UI elements in your XML file.
3.3. Adding UI Elements
To add UI elements, simply declare the desired elements as XML tags within the appropriate layout container. You can set various attributes to configure the appearance and behavior of each UI element. For example, to add a Button element, use the following XML snippet:
xml <Button android:id="@+id/myButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Click Me" />
3.4. Configuring UI Element Attributes
UI element attributes determine how the element appears and behaves. Common attributes include layout_width, layout_height, text, textColor, background, and padding. By assigning appropriate values to these attributes, you can customize the appearance of your UI elements to match your app’s design.
4. Connecting XML Layouts with Kotlin
4.1. Accessing UI Elements in Kotlin
To interact with UI elements declared in an XML layout, you need to obtain references to them in your Kotlin code. This can be done using the findViewById method or the more modern approach of data binding. The findViewById method retrieves a UI element by its unique identifier, while data binding generates binding classes that allow direct access to UI elements without the need for explicit view lookups.
Here’s an example of accessing a TextView and Button using findViewById:
kotlin val textView = findViewById<TextView>(R.id.myTextView) val button = findViewById<Button>(R.id.myButton)
4.2. Event Handling
UI elements often require event handling to respond to user interactions such as button clicks or text input. In Kotlin, you can attach event listeners to UI elements using lambda expressions or anonymous inner classes. For example, to handle a button click event, you can write the following code:
kotlin button.setOnClickListener { // Handle button click here }
4.3. Data Binding
Data binding is a powerful feature in Android that allows you to bind UI elements directly to data sources in your Kotlin code. It eliminates the need for manual view lookups and simplifies the codebase by providing a seamless connection between the UI and the underlying data. Data binding can significantly enhance the maintainability and scalability of your app’s UI code.
5. Best Practices for Android UI Design
5.1. Keep it Simple and Intuitive
Simplicity and intuitiveness are key aspects of effective UI design. Avoid cluttering the interface with unnecessary elements and strive for a clean and minimalistic design. Use meaningful icons, labels, and visual cues to guide users and ensure that the app’s functionality is easy to understand and navigate.
5.2. Consistency and Alignment
Maintaining consistency across your app’s UI is crucial for creating a cohesive and professional look. Use consistent typography, colors, and spacing throughout your app to establish a visual identity. Additionally, ensure proper alignment of UI elements to create a visually pleasing and balanced interface.
5.3. Responsiveness and Accessibility
Design your UI to be responsive across different screen sizes and orientations. Test your layouts on various devices and use responsive layout containers to adapt to different screen densities. Moreover, consider accessibility guidelines and provide options for users with disabilities, such as text-to-speech support and adjustable font sizes.
Conclusion
In this blog post, we explored the basics of creating Android user interfaces using XML layouts and Kotlin. We learned how to define UI elements, position them on the screen, and customize their appearance. We also discussed connecting XML layouts with Kotlin code through view lookups and data binding. By following best practices and guidelines, you can create visually appealing and user-friendly UIs that enhance the overall experience of your Android apps. With Kotlin and XML layouts, the possibilities for crafting engaging user interfaces are endless. Happy coding!
Table of Contents
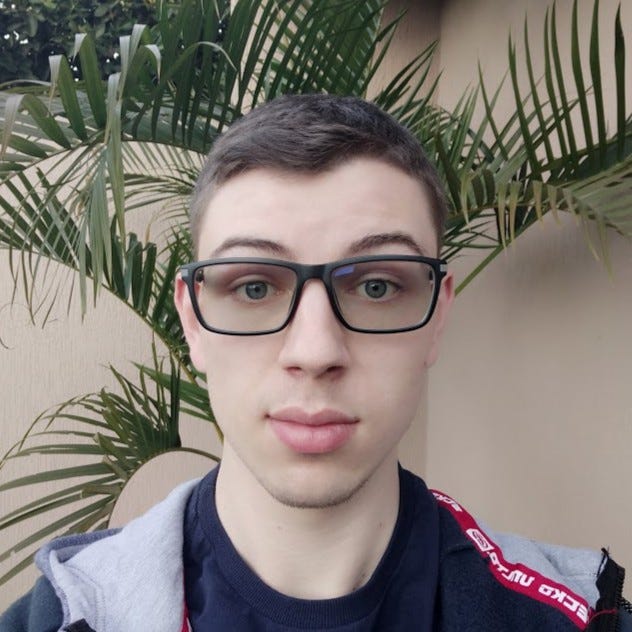
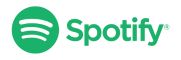