From Novice to Pro: A Complete Beginner’s Guide to Mastering Kotlin
Kotlin, a statically typed programming language designed for the Java Virtual Machine (JVM), has been making waves in the world of programming since its introduction by JetBrains in 2011. Given that Google endorsed Kotlin as an official language for Android application development in 2017, there has been an exponential growth in its popularity, leading to an increasing demand to hire Kotlin developers. Whether you are a beginner looking to learn your first programming language, an experienced developer wanting to expand your skills, or a company seeking to hire Kotlin developers, Kotlin is an excellent choice. In this comprehensive guide, we will delve into the fundamentals of Kotlin, making your journey to mastering Kotlin, or your journey to hire Kotlin developers, much smoother.
What is Kotlin?
Kotlin is a modern programming language that combines object-oriented and functional programming features. Itβs interoperable with Java, meaning it can use all existing Java frameworks and libraries, bringing a blend of simplicity, clarity, and flexibility to developers. Let’s go through some of the crucial aspects of Kotlin one should master as a beginner.
Variables and Data Types
Variables in Kotlin are defined using either `var` (mutable variable) or `val` (immutable variable). Here’s an example:
```kotlin var mutableVariable: Int = 10 val immutableVariable: String = "Hello, Kotlin!" ```
In the above example, `mutableVariable` is an integer type variable that can be changed, whereas `immutableVariable` is a string type variable that cannot be changed once assigned.
Kotlin also supports a variety of other data types like `Long`, `Double`, `Boolean`, `Char` and `Float`.
Conditional Statements
Kotlin supports standard conditional statements such as `if`, `else if`, and `else`. Additionally, `when` is a powerful control flow statement, similar to the switch statement in other languages. Here’s an example:
```kotlin var number = 3 when (number) { 1 -> print("One") 2 -> print("Two") 3 -> print("Three") else -> { print("Number not found") } } ```
In the above code, `when` checks the value of the `number` variable and executes the corresponding branch. If none of the conditions are met, it executes the `else` branch.
Functions
In Kotlin, functions are declared using the `fun` keyword. Here’s an example of a simple function that adds two numbers:
```kotlin fun addNumbers(num1: Int, num2: Int): Int { return num1 + num2 } ```
Here, `addNumbers` is a function that takes two integers as arguments and returns their sum.
Classes and Objects
Like other object-oriented programming languages, Kotlin uses classes as blueprints for objects. Here’s a simple class definition:
```kotlin class Person(name: String, age: Int) { var name: String = name var age: Int = age } ```
In this code, `Person` is a class with two properties, `name` and `age`. An instance of this class can be created as follows:
```kotlin val person1 = Person("John Doe", 25) ```
Null Safety
One of Kotlin’s most notable features is its inherent null safety. In Kotlin, variables cannot hold a null value by default. You need to explicitly specify if a variable can be null using the `?` symbol. This feature greatly reduces the likelihood of null pointer exceptions in your code.
```kotlin var nullableVar: String? = null var nonNullableVar: String = "Hello, Kotlin" ```
In the above code, `nullableVar` can hold a null value, but `nonNullableVar` cannot.
Lambda Functions and Higher-Order Functions
Kotlin supports both lambda functions and higher-order functions, enhancing the language’s capabilities to handle functional programming paradigms. This robustness is one of the reasons why organizations are choosing to hire Kotlin developers. A lambda function is a short anonymous function that isn’t declared but is passed immediately as an expression. A higher-order function, on the other hand, is a function that takes functions as parameters or returns a function.
Understanding these concepts not only increases your proficiency in Kotlin but also puts you in a better position if you’re looking to hire Kotlin developers, as you’ll have a clear understanding of the skill set you’re seeking.
Here’s an example of a lambda function:
```kotlin val multiplyByTwo: (Int) -> Int = { number -> number * 2 } println(multiplyByTwo(4)) // Prints 8 ```
And here’s an example of a higher-order function:
```kotlin fun calculate(a: Int, b: Int, operation: (Int, Int) -> Int): Int { return operation(a, b) } val sum = calculate(5, 3, { a, b -> a + b }) println(sum) // Prints 8 ```
In the higher-order function example, `calculate` is a function that takes two integers and a function as parameters, applies the function to the integers, and returns the result.
Conclusion
Kotlin is a powerful language that brings together the best features of object-oriented and functional programming languages. Its simplicity and safety features, such as null safety, make it an excellent choice for beginners and seasoned developers alike, thus leading to an increasing demand to hire Kotlin developers.
With the basics covered in this guide, you’re well on your way to mastering Kotlin, whether you’re looking to add it to your own skill set or aiming to hire Kotlin developers for your team. But remember, the best way to learn is by doing. Therefore, take what you’ve learned here and start building your own Kotlin applications, or use this knowledge to better inform your search when you hire Kotlin developers.
Table of Contents
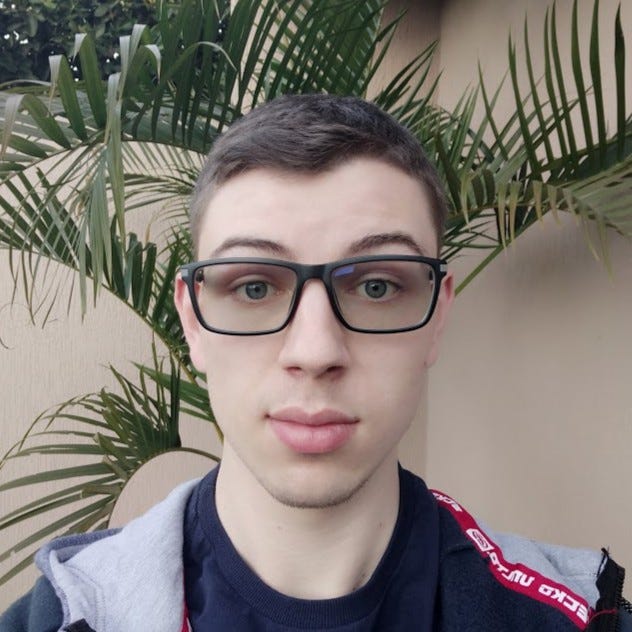
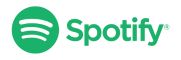