Revolutionize Your IoT Devices with Kotlin and BLE Integration
The age of the Internet of Things (IoT) is upon us, with smart devices, wearables, and even household appliances connecting with our smartphones, tablets, and computers. At the core of these connections, especially in battery-powered devices, is Bluetooth Low Energy (BLE) technology. Kotlin, with its expressive and concise syntax, has become a preferred language for many Android developers. Combining the simplicity and power of Kotlin with BLE, developers can build robust IoT applications efficiently.
In this article, we’ll delve into building a basic IoT application using Kotlin to interact with BLE devices.
1. Why BLE?
Bluetooth Low Energy, a subset of the Bluetooth 4.0 specification, was designed for low-power, short-range communications. Its main advantages include:
– Low Power Consumption: Perfect for battery-operated devices.
– Short Range: Ideally suited for personal area networks.
– Low Cost: Affordable to implement in many devices.
2. Setting up the Environment:
Ensure you have Android Studio installed with Kotlin support. The AndroidManifest.xml should have the necessary permissions:
```xml <uses-permission android:name="android.permission.BLUETOOTH"/> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/> ```
Remember: Location permission is necessary as BLE device discovery can be used for location tracking.
3. Kotlin & BLE: Getting Started:
Scanning for Devices:
To start discovering BLE devices, use the BluetoothAdapter.
```kotlin val bluetoothAdapter: BluetoothAdapter? = BluetoothAdapter.getDefaultAdapter() bluetoothAdapter?.startLeScan(leScanCallback) ```
Define the `leScanCallback`:
```kotlin private val leScanCallback = object : BluetoothAdapter.LeScanCallback { override fun onLeScan(device: BluetoothDevice?, rssi: Int, scanRecord: ByteArray?) { // Handle the discovered device here } } ```
Connecting to a Device:
Once you discover a device, you can connect to it:
```kotlin val bluetoothGatt = device?.connectGatt(context, false, gattCallback) ```
Define the `gattCallback`:
```kotlin private val gattCallback = object : BluetoothGattCallback() { override fun onConnectionStateChange(gatt: BluetoothGatt, status: Int, newState: Int) { when (newState) { BluetoothProfile.STATE_CONNECTED -> { // Device connected gatt.discoverServices() } BluetoothProfile.STATE_DISCONNECTED -> { // Device disconnected } } } } ```
4. Communicating with the Device:
Reading from a Device:
Once connected, you can read characteristics from the BLE device.
```kotlin val characteristic: BluetoothGattCharacteristic = ... // get the characteristic you want to read gatt.readCharacteristic(characteristic) ```
Then, handle the read operation in the `gattCallback`:
```kotlin override fun onCharacteristicRead(gatt: BluetoothGatt, characteristic: BluetoothGattCharacteristic, status: Int) { if (status == BluetoothGatt.GATT_SUCCESS) { val data: ByteArray = characteristic.value // Process the data } } ```
Writing to a Device:
Writing data to a BLE device is similar:
```kotlin characteristic.value = yourByteArray gatt.writeCharacteristic(characteristic) ```
5. Disconnecting
Remember to properly disconnect and close your GATT connection when done:
```kotlin bluetoothGatt?.disconnect() bluetoothGatt?.close() ```
6. Practical Applications
With the basics set, you can explore a myriad of applications:
– Smart Home: Control lights, thermostats, and door locks.
– Wearables: Fetch health data from smartwatches or fitness bands.
– Retail: BLE beacons for location-based promotions.
– Healthcare: Continuous patient monitoring with medical devices.
Conclusion
Kotlin simplifies BLE integration on Android by reducing boilerplate and improving readability. The combination of Kotlin and BLE offers powerful tools in the hands of developers to imagine and create the next generation of IoT applications.
When building with BLE, remember to handle edge cases (like BLE being turned off, permissions not granted, etc.) and always respect user privacy. Happy coding!
Table of Contents
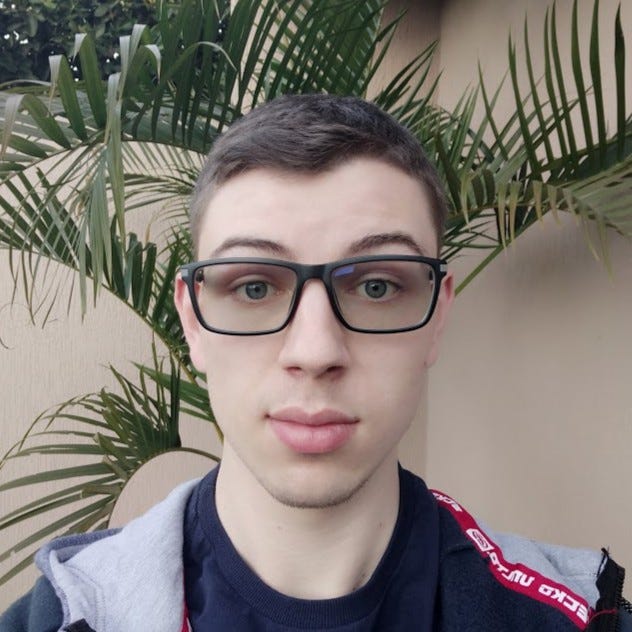
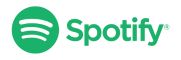