Discover, Connect, Communicate: Bluetooth Made Effortless with Kotlin
With the ever-increasing trend of IoT (Internet of Things) and wearable devices, communication between gadgets has become paramount. One of the most prevalent means of short-range communication is Bluetooth. Kotlin, with its concise syntax and Android support, makes it easier for developers to manage Bluetooth communications. In this post, we will explore how to leverage Kotlin’s power for Bluetooth connectivity.
1. Bluetooth Basics
Before diving into code, it’s essential to understand a few Bluetooth basics:
- Bluetooth Classic vs. Bluetooth Low Energy (BLE):
– Bluetooth Classic is primarily used for connecting phones to headphones, car stereos, and other audio equipment.
– BLE is optimized for devices that run on coin-cell batteries, such as fitness trackers or smart home gadgets.
- Pairing: The process where two Bluetooth devices establish a connection by sharing a common secret (PIN).
- Profile: Defines how two devices communicate over Bluetooth. For example, the Advanced Audio Distribution Profile (A2DP) describes how audio can be streamed from one device to another.
2. Setting Up Kotlin for Bluetooth
Before implementing Bluetooth functionalities, ensure you’ve requested the necessary permissions in the `AndroidManifest.xml`:
```xml <uses-permission android:name="android.permission.BLUETOOTH"/> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/> <!-- For BLE devices --> <uses-permission android:name="android.permission.BLUETOOTH_PRIVILEGED"/> <uses-feature android:name="android.hardware.bluetooth_le" android:required="true"/> ```
3. Discovering Devices
Before you can connect to a device, you need to discover it. Here’s a simple way to discover nearby Bluetooth devices using Kotlin:
```kotlin val bluetoothAdapter: BluetoothAdapter? = BluetoothAdapter.getDefaultAdapter() bluetoothAdapter?.startDiscovery() val receiver = object : BroadcastReceiver() { override fun onReceive(context: Context?, intent: Intent?) { val action: String = intent!!.action!! when(action) { BluetoothDevice.ACTION_FOUND -> { val device: BluetoothDevice = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE)!! val deviceName = device.name val deviceAddress = device.address // Add the device to your list } } } } val filter = IntentFilter(BluetoothDevice.ACTION_FOUND) registerReceiver(receiver, filter) ```
4. Connecting to a Device
Once discovered, you can establish a connection using a `BluetoothSocket`:
```kotlin val device: BluetoothDevice = bluetoothAdapter!!.getRemoteDevice(deviceAddress) val uuid: UUID = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB") // Standard SerialPortService ID val socket: BluetoothSocket = device.createRfcommSocketToServiceRecord(uuid) socket.connect() ```
With an established connection, you can send and receive data through the input and output streams.
5. BLE in Kotlin
For devices that use BLE:
- Discovering Services:
After connecting to a BLE device, you can discover its services:
```kotlin val gattCallback = object : BluetoothGattCallback() { override fun onServicesDiscovered(gatt: BluetoothGatt?, status: Int) { if (status == BluetoothGatt.GATT_SUCCESS) { gatt?.services?.forEach { service -> // Handle discovered services } } } } val bluetoothGatt = device.connectGatt(context, false, gattCallback) bluetoothGatt.discoverServices() ```
- Reading and Writing to a Characteristic:
If you wish to read from a characteristic:
```kotlin val characteristic: BluetoothGattCharacteristic = ... // Obtain the characteristic bluetoothGatt.readCharacteristic(characteristic) ```
For writing:
```kotlin characteristic.value = ... // Your data bluetoothGatt.writeCharacteristic(characteristic) ```
Conclusion
Kotlin simplifies the process of integrating Bluetooth functionalities in your Android apps. Whether it’s connecting to traditional Bluetooth devices or the new BLE gadgets, Kotlin and its standard libraries provide all the tools you need for a smooth experience.
With the examples provided, you can initiate connections, communicate, and even traverse the world of BLE with ease. Remember, while Bluetooth provides an excellent medium for device communication, always ensure you follow best practices, like managing connections properly and ensuring user privacy.
Here’s to seamless connectivity in the Kotlin world!
Table of Contents
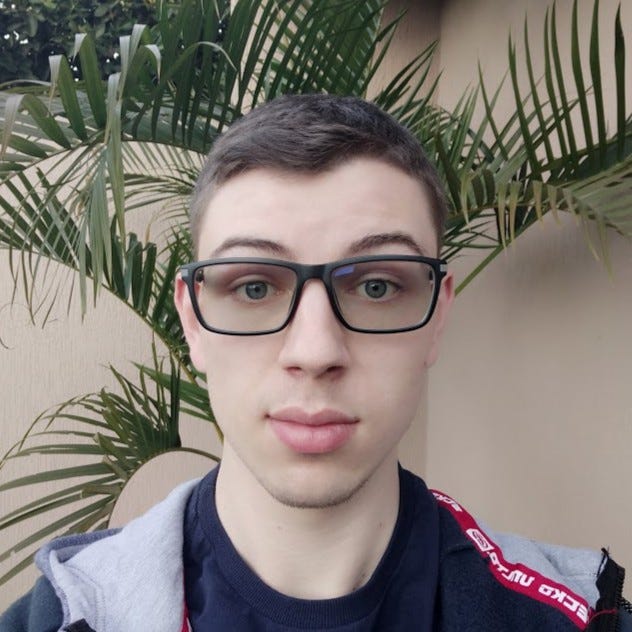
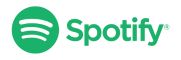