Mastering Kotlin Coroutines: Advanced Tips and Tricks
Kotlin coroutines have revolutionized asynchronous programming in Kotlin by providing a powerful and intuitive way to write asynchronous code. With their ability to simplify concurrency, coroutines have become an essential tool for building efficient and responsive applications.
In this blog post, we will dive deeper into Kotlin coroutines and explore advanced tips and tricks that will help you master this powerful concurrency framework. We will cover various techniques, patterns, and code samples to write efficient and robust asynchronous code using coroutines.
1. Error Handling in Coroutines
1.1 Handling Exceptions in Coroutine Builders
When working with coroutines, it’s crucial to handle exceptions appropriately. In coroutine builders such as launch or async, exceptions can be caught and handled using a try-catch block. Additionally, the CoroutineExceptionHandler can be used to handle uncaught exceptions globally.
kotlin val coroutineExceptionHandler = CoroutineExceptionHandler { _, throwable -> // Handle the exception here } GlobalScope.launch(coroutineExceptionHandler) { // Coroutine code here }
1.2 SupervisorScope for Fault-Tolerant Coroutines
The SupervisorScope is a coroutine scope that provides fault-tolerance for child coroutines. If a child coroutine fails, it won’t affect the other child coroutines or the parent coroutine.
kotlin GlobalScope.launch { supervisorScope { launch { // Child coroutine 1 } launch { // Child coroutine 2 } } }
2. Cancellation and Timeouts
2.1 Structured Concurrency and Cancellation
Structured concurrency ensures that all coroutines launched in a specific scope are canceled when the scope is canceled. By using structured concurrency, we can avoid leaking resources and ensure proper cancellation of coroutines.
kotlin val scope = CoroutineScope(Dispatchers.Main) val job = scope.launch { // Coroutine code here } job.cancel() // Cancels the coroutine and its children
2.2 Timeout and withTimeout Functions
The withTimeout function allows you to specify a timeout for a coroutine, after which it will be canceled automatically. This is useful when you want to set a maximum execution time for a coroutine.
kotlin withTimeout(5000) { // Coroutine code here }
3. Coroutine Context and Dispatchers
3.1 Understanding Coroutine Context
Coroutine context provides a set of elements that define the behavior and context of a coroutine. It includes a coroutine dispatcher, which determines the thread or thread pool on which the coroutine runs.
kotlin val context = Dispatchers.IO + CoroutineName("CoroutineName") val job = GlobalScope.launch(context) { // Coroutine code here }
3.2 Customizing Coroutine Dispatchers
You can create custom coroutine dispatchers to control the execution of coroutines. For example, you can create a dispatcher that limits the number of concurrent coroutines or dispatches them on a specific thread pool.
kotlin val customDispatcher = Executors.newFixedThreadPool(4).asCoroutineDispatcher() val job = GlobalScope.launch(customDispatcher) { // Coroutine code here }
4. Coroutine Channels
4.1 Basics of Channels
Channels are a way to communicate between coroutines through streams. They provide a flow of data that can be sent and received asynchronously. Channels can be used to build complex pipelines or communicate between different parts of an application.
kotlin val channel = Channel<Int>() // Sender coroutine GlobalScope.launch { for (i in 1..10) { channel.send(i) } channel.close() } // Receiver coroutine GlobalScope.launch { for (value in channel) { // Process value } }
4.2 Buffered Channels and Fan-out
Buffered channels allow sending multiple elements without suspending. This can be useful when the sender coroutine is faster than the receiver coroutine. Additionally, fan-out is a technique to distribute work among multiple coroutines using channels.
kotlin val channel = Channel<Int>(capacity = 10) // Sender coroutine GlobalScope.launch { repeat(100) { channel.send(it) } channel.close() } // Receiver coroutines repeat(5) { GlobalScope.launch { for (value in channel) { // Process value } } }
5. Flow and StateFlow
5.1 Asynchronous Streams with Flow
Flow is a reactive streams-like API for emitting asynchronous sequences of values. It provides a declarative and composable way to handle asynchronous data streams.
kotlin fun fetchUsers(): Flow<User> = flow { // Fetch users asynchronously emit(user) } // Collecting the flow fetchUsers().collect { user -> // Process user }
5.2 StateFlow for State Management
StateFlow is a type of flow that represents a state that can be observed by multiple components. It provides a way to handle state changes and notify observers when the state changes.
kotlin val counter = MutableStateFlow(0) // Observing the state flow counter.collect { value -> // Update UI with new value } // Updating the state flow counter.value = 1
6. Testing Coroutines
6.1 Testing Suspended Functions
The runBlocking function can be used to test suspended functions that require a coroutine context. It creates a new coroutine and blocks until its completion.
kotlin @Test fun testSuspendedFunction() = runBlocking { val result = mySuspendedFunction() // Assert result }
6.2 Testing Coroutines with TestCoroutineDispatcher
The TestCoroutineDispatcher is a dispatcher specifically designed for testing coroutines. It allows you to control the execution of coroutines and test time-dependent behavior easily.
kotlin @Test fun testCoroutines() = runBlockingTest { val dispatcher = TestCoroutineDispatcher() val scope = CoroutineScope(dispatcher) // Run test code with the test dispatcher scope.launch { // Coroutine code here } // Advance time and verify results dispatcher.advanceTimeBy(1000) // Assert results }
Conclusion
In this blog post, we have explored advanced tips and tricks to master Kotlin coroutines. We covered various topics, including error handling, cancellation and timeouts, coroutine context and dispatchers, coroutine channels, flow and StateFlow, and testing coroutines. By leveraging these advanced techniques, you can write efficient, robust, and highly concurrent code using Kotlin coroutines.
Kotlin coroutines provide a flexible and powerful approach to handle asynchronous programming, and with the knowledge gained from this blog post, you are well-equipped to take full advantage of their capabilities. Happy coding with Kotlin coroutines!
Table of Contents
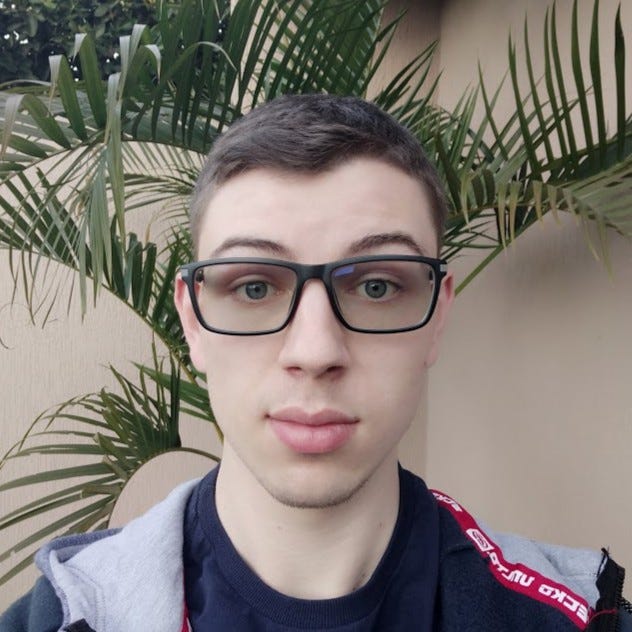
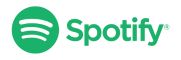