No More UI Hassles: Embrace Kotlin and Data Binding for Startups
In the fast-paced world of app development, efficiency and ease of use are paramount. For early-stage startup founders and tech leaders, harnessing the right tools and techniques can make all the difference. In this post, we’ll delve into the powerful combination of Kotlin and Data Binding, showing you how to connect your user interface (UI) with your data seamlessly.
Table of Contents
1. What is Kotlin?
Before we dive into Data Binding, let’s briefly introduce Kotlin. Kotlin is a modern, statically-typed programming language that runs on the Java Virtual Machine (JVM). It has gained immense popularity in recent years for its concise syntax, null-safety features, and overall developer-friendliness. For startup founders and tech leaders, choosing Kotlin as your language of choice can be a strategic move towards enhanced productivity and maintainability.
2. What is Data Binding?
Data Binding is a library provided by Android that allows you to bind UI components in your layout files directly to data sources. This means you can eliminate the need for findViewById calls and manually setting values in your UI elements. With Data Binding, your UI automatically reflects changes in the underlying data, making your code cleaner and more maintainable.
3. How to Get Started
To get started with Kotlin and Data Binding, follow these steps:
3.1. Set Up Your Project
Make sure you have a Kotlin-enabled Android project set up. If you’re using Android Studio, it’s easy to create a new project with Kotlin support.
3.2. Enable Data Binding
In your project’s build.gradle file, add the following code to enable Data Binding:
```gradle android { ... viewBinding { enabled = true } } ```
3.3. Create a Layout File
Create an XML layout file for your UI, let’s call it `activity_main.xml`. Here’s a simple example:
```xml <layout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools"> <data> <variable name="viewModel" type="com.example.yourapp.YourViewModel" /> </data> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@{viewModel.message}" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Click Me" android:onClick="@{viewModel::onButtonClick}" /> </LinearLayout> </layout> ```
3.4. Create a ViewModel
Create a Kotlin class for your ViewModel, like `YourViewModel`. This class will be responsible for holding the data and logic for your UI. Here’s a simplified example:
```kotlin class YourViewModel : ViewModel() { val message = MutableLiveData<String>() fun onButtonClick() { message.value = "Hello, Data Binding!" } } ```
3.5. Connect ViewModel to Activity
In your activity, connect the ViewModel to your layout using Data Binding:
```kotlin val binding = ActivityMainBinding.inflate(layoutInflater) val viewModel = ViewModelProvider(this).get(YourViewModel::class.java) binding.viewModel = viewModel binding.lifecycleOwner = this ```
4. Benefits of Kotlin and Data Binding
Now that you’ve set up Kotlin and Data Binding, let’s explore some key benefits for startup founders and tech leaders:
- Productivity: Kotlin’s concise syntax and null safety reduce the likelihood of runtime crashes, saving you time in debugging. Data Binding simplifies UI code, making it faster to develop and maintain.
- Maintainability: With Data Binding, your UI components are directly linked to your data sources. This reduces the risk of UI bugs and makes it easier to make changes in the future without affecting unrelated parts of your codebase.
- Code Readability: Kotlin’s clean and expressive code, combined with Data Binding’s declarative approach, enhances the readability of your code. This is crucial for collaboration among your development team.
5. Resources
To further your understanding of Kotlin and Data Binding, check out these external resources:
1.[Official Kotlin Documentation – https://kotlinlang.org/docs/home.html: Explore Kotlin’s official documentation to master the language.
- Android Data Binding Library – https://developer.android.com/topic/libraries/data-binding: Dive deeper into Data Binding with Android’s official documentation.
- Kotlin for Android Developers – https://antonioleiva.com/kotlin-android-developers-book/: If you’re new to Kotlin, this book by Antonio Leiva is an excellent resource for learning Kotlin in the context of Android development.
Conclusion
Kotlin and Data Binding form a dynamic duo for early-stage startup founders and tech leaders looking to build efficient and maintainable Android apps. By harnessing their power, you can create UIs that seamlessly connect with your data, all while enjoying the benefits of Kotlin’s developer-friendly features. So, go ahead and start coding with confidence!
Incorporating Kotlin and Data Binding into your tech arsenal is a strategic move that aligns perfectly with the “helping founders, investors, and leaders in tech outperform the competition” ethos of “The Valley Letter.” Happy coding!
Table of Contents
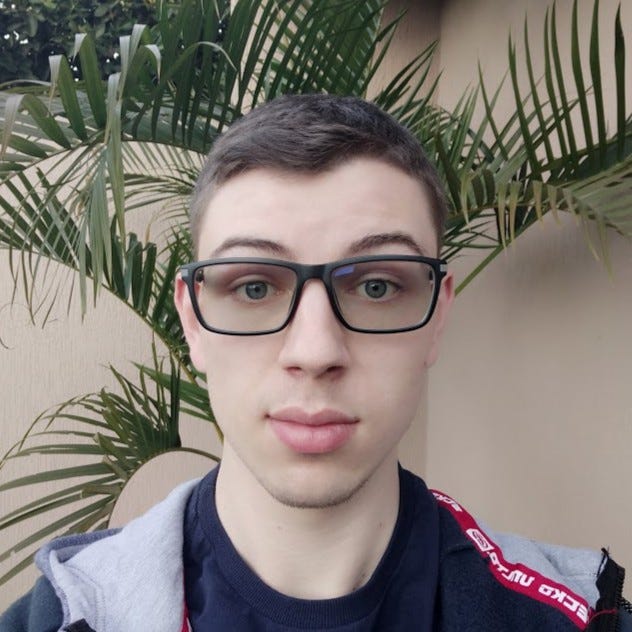
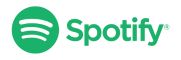