Cross-Platform Showdown: Kotlin Multi Platform Meets Flutter
Cross-platform development has been the holy grail for many developers. The idea of writing a single codebase to target multiple platforms is alluring, promising faster development cycles, reduced costs, and a consistent user experience. Two major players in this domain are Kotlin Multiplatform (KMP) and Flutter. This article will explore both, offering insights and examples.
1. Kotlin Multiplatform (KMP)
Kotlin, initially developed by JetBrains as an alternative to Java for Android, has since expanded its horizons. With KMP, Kotlin offers a way to share code between different platforms, including iOS, Android, Web, and Desktop.
Example: Sharing Business Logic between iOS and Android**
Let’s consider an app that fetches user profiles from an API.
Common Code (in Kotlin):
```kotlin data class UserProfile(val id: Int, val name: String, val email: String) expect class ProfileApi { fun fetchProfile(): UserProfile } fun getUserName(): String { val profile = ProfileApi().fetchProfile() return profile.name } ```
In the above, the `expect` keyword is used to declare an expected platform-specific implementation. Now, for each platform:
Android Implementation:
```kotlin actual class ProfileApi { fun fetchProfile(): UserProfile { // Android-specific API fetching return UserProfile(1, "Android User", "android@example.com") } } ```
iOS Implementation:
```kotlin actual class ProfileApi { fun fetchProfile(): UserProfile { // iOS-specific API fetching return UserProfile(1, "iOS User", "ios@example.com") } } ```
2. Flutter
Developed by Google, Flutter is a UI toolkit that allows developers to create natively compiled applications for mobile, web, and desktop from a single codebase. It uses the Dart language.
Example: A Simple Flutter App
This app will display a user profile fetched from an API.
```dart import 'package:flutter/material.dart'; void main() => runApp(ProfileApp()); class ProfileApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Profile Viewer', theme: ThemeData(primarySwatch: Colors.blue), home: ProfileScreen(), ); } }
class ProfileScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('User Profile')), body: Center( child: FutureBuilder<UserProfile>( future: fetchProfile(), builder: (context, snapshot) { if (snapshot.connectionState == ConnectionState.done) { return Text(snapshot.data!.name); } else if (snapshot.error != null) { return Text("Error: ${snapshot.error}"); } else { return CircularProgressIndicator(); } }, ), ), ); } }
class UserProfile { final int id; final String name; final String email; UserProfile({required this.id, required this.name, required this.email}); // Assume this function fetches the data from an API static Future<UserProfile> fetchProfile() async { await Future.delayed(Duration(seconds: 2)); return UserProfile(id: 1, name: 'Flutter User', email: 'flutter@example.com'); } } ```
3. Comparison
– Learning Curve: For Java or Kotlin developers, transitioning to KMP might be smoother. Flutter, on the other hand, demands learning Dart – a new language for most developers. However, Dart is intuitive and easy to pick up.
– Performance: Both KMP and Flutter offer near-native performance. However, Flutter controls the entire rendering process, making it slightly more predictable in terms of UI consistency across platforms.
– Library/Support: Flutter has a rich set of widgets and a growing community, providing numerous plugins/packages. Kotlin has the robustness and maturity of Kotlin/Java libraries for Android and can access native libraries on other platforms, giving it a distinct edge.
– UI/UX: Flutter’s widget-based approach ensures consistent UI across platforms. With KMP, you’ll use native UI components, meaning the UI may vary based on platform conventions.
Conclusion
Both Kotlin Multiplatform and Flutter have their strengths. KMP shines when you want to reuse business logic and maintain native UIs, while Flutter excels in delivering a consistent UI with a single codebase. Developers should weigh their project needs, existing expertise, and long-term goals before choosing a platform.
As cross-platform development continues to evolve, the choices will only get richer, driving the tech industry towards more efficient, cost-effective, and user-friendly solutions.
Table of Contents
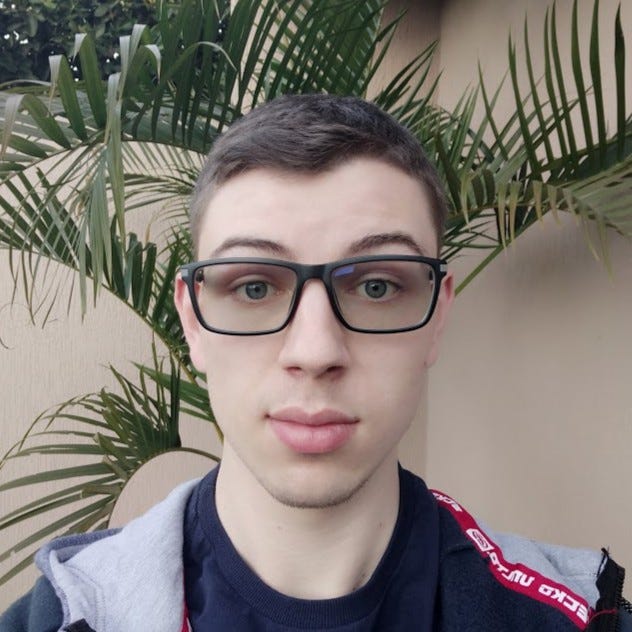
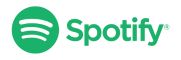