Boost Your App’s Efficiency: The Kotlin, GraphQL, and Apollo Trio You Need
In the ever-evolving landscape of app development, keeping up with the latest technologies is crucial to stay competitive. Two powerful tools in the developer’s toolkit are Kotlin and GraphQL, and when combined with Apollo, they become a dynamic trio that simplifies API consumption and enhances the development process.
Table of Contents
Kotlin, a statically typed programming language developed by JetBrains, has gained immense popularity in recent years. It’s known for its concise syntax, strong type inference, and seamless interoperability with Java, making it a top choice for Android app development and beyond. On the other hand, GraphQL, a query language for APIs, offers a flexible and efficient approach to fetching data. When you add Apollo, a comprehensive GraphQL client, to the mix, you unlock a world of possibilities for your app development.
1. Why Kotlin?
Kotlin’s rise in the tech world is not without reason. It offers several advantages that make it a compelling choice for developers:
- Concise Syntax: Kotlin’s syntax is concise and expressive, reducing boilerplate code and making codebase maintenance easier.
- Safety and Nullability: Kotlin’s type system helps catch null-pointer exceptions at compile time, enhancing the reliability of your code.
- Interoperability: Kotlin seamlessly interoperates with Java, allowing developers to leverage existing Java libraries and tools.
- Modern Language Features: Kotlin incorporates modern language features like lambdas, extension functions, and smart casts, improving code readability and maintainability.
2. Why GraphQL?
GraphQL revolutionizes API consumption by addressing some of the limitations of REST APIs:
- Precise Data Retrieval: GraphQL enables clients to request only the data they need, reducing over-fetching and under-fetching of data.
- Multiple Queries in One Request: Clients can send multiple queries or mutations in a single request, reducing network overhead and improving performance.
- Real-time Data: GraphQL supports real-time updates through subscriptions, making it ideal for building interactive applications.
3. Introducing Apollo for Kotlin
Apollo is a GraphQL client library available for various platforms, including Kotlin. It simplifies the integration of GraphQL into your Kotlin-based projects. Here’s how it works:
- Schema Generation: Apollo generates type-safe Kotlin classes from your GraphQL schema, ensuring compile-time safety.
- Query Builder: You can use Apollo’s fluent query builder to construct queries and mutations in a type-safe manner.
- Caching and Optimizations: Apollo offers caching capabilities, reducing redundant network requests and improving app performance.
4. Examples of Kotlin and GraphQL with Apollo
Let’s explore a few examples of how Kotlin and GraphQL with Apollo can simplify API consumption:
- Fetching User Data:
```kotlin val query = GetUserQuery.builder() .id(userId) .build() val response = apolloClient.query(query).execute() if (!response.hasErrors()) { val user = response.data?.user // Handle user data here } else { val errors = response.errors // Handle errors } ```
In this example, we use Apollo to fetch user data using a type-safe query.
- Updating User Information:
```kotlin val mutation = UpdateUserMutation.builder() .id(userId) .name(newName) .build() val response = apolloClient.mutate(mutation).execute() if (!response.hasErrors()) { // User information updated successfully } else { val errors = response.errors // Handle errors } ```
Here, we use Apollo to execute a mutation to update user information.
- Real-time Notifications:
```kotlin val subscription = NewMessageSubscription.builder() .channelId(channelId) .build() val subscriptionCallback = object : ApolloSubscriptionCall.Callback<NewMessageSubscription.Data>() { override fun onResponse(response: Response<NewMessageSubscription.Data>) { val message = response.data?.newMessage // Handle new message in real-time } override fun onFailure(e: ApolloException) { // Handle subscription failure } } apolloClient.subscribe(subscription).enqueue(subscriptionCallback) ```
In this example, we use Apollo to subscribe to real-time notifications, ensuring users receive new messages as they happen.
Conclusion
Kotlin and GraphQL with Apollo provide a potent combination for simplifying API consumption and enhancing the development experience. With Kotlin’s concise syntax, type safety, and interoperability, and GraphQL’s precise data retrieval and real-time capabilities, developers can build modern, efficient, and scalable applications.
By leveraging Apollo’s features tailored for Kotlin, you can unlock the full potential of GraphQL in your projects. So, if you’re an early-stage startup founder, VC investor, or tech leader looking to outperform the competition, consider incorporating Kotlin and GraphQL with Apollo into your tech stack.
Stay ahead in the tech race and empower your team to create outstanding products with these cutting-edge technologies.
References:
- Kotlin Programming Language – https://kotlinlang.org/
- GraphQL: A Query Language for APIs – https://graphql.org/
- Apollo GraphQL Client – https://www.apollographql.com/docs/android/
Table of Contents
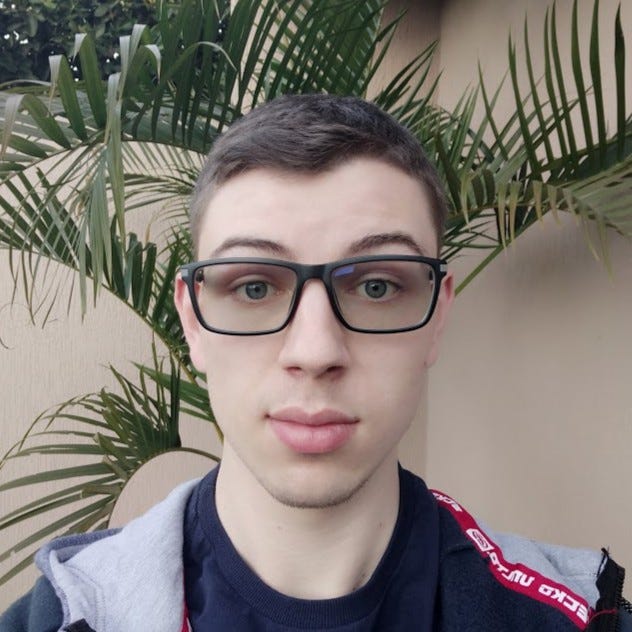
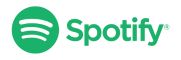