Mastering the Art of Hiring Kotlin Developers: A Comprehensive Guide
Kotlin, the versatile programming language, has transformed the landscape of Android app development and beyond. To build exceptional projects, hiring skilled Kotlin developers is paramount. This guide is your compass to navigate the interview process, probing candidates’ technical prowess, problem-solving acumen, and expertise in the Kotlin ecosystem. Let’s delve into the essential interview questions and strategies that will empower you to pinpoint the perfect Kotlin developer for your team.
Table of Contents
1. How to Hire Kotlin Developers
When embarking on your journey to hire Kotlin developers, these steps will guide you toward success:
- Job Requirements: Define the specific job prerequisites, outlining the skills and expertise you desire.
- Search Avenues: Harness job postings, online platforms, and tech communities to unearth potential candidates.
- Screening: Scrutinize candidates’ mastery of Kotlin, relevant experience, and additional proficiencies.
- Technical Assessment: Forge a robust technical assessment to gauge coding prowess and problem-solving capabilities.
2. Core Skills of Kotlin Developers to Look For
When assessing potential Kotlin developers, keep an eye out for these pivotal skills:
- Kotlin Proficiency: A firm grasp of Kotlin syntax, features, and best practices.
- Android Development: For roles involving mobile app creation, experience in Kotlin-driven Android development is indispensable.
- Java Compatibility: Proficiency in the seamless interaction between Kotlin and Java.
- Asynchronous Programming: Aptitude in managing asynchronous operations using Coroutines or RxJava.
- Unit Testing: Experience in crafting effective unit tests for Kotlin code.
- Code Hygiene: A commitment to writing clean, maintainable, and comprehensible code.
- Problem-Solving Acumen: The ability to dissect intricate problems and devise effective solutions.
3. An Overview of the Kotlin Developer Hiring Process
Here’s a panoramic view of the Kotlin developer hiring process:
3.1 Defining Job Requirements and Skillsets
Lay the groundwork by crystallizing the job prerequisites and the specific skills and knowledge you’re seeking.
3.2 Crafting Compelling Job Descriptions
Create magnetic job descriptions that accurately mirror the role, magnetizing the right candidates.
3.3 Crafting Kotlin Developer Interview Questions
Craft a well-rounded set of interview questions encompassing Kotlin language intricacies, problem-solving adeptness, and relevant technologies.
4. Sample Kotlin Developer Interview Questions and Answers
Dive into these sample questions with comprehensive answers to assess candidates’ Kotlin skills:
Q1. What are the key advantages of using Kotlin over Java for Android development?
A: Kotlin offers enhanced expressiveness, null safety, concise code, and seamless interoperability with Java. Its modern features streamline development and lead to more robust apps.
Q2. Explain the concept of nullable types in Kotlin and how you handle null safety.
A: In Kotlin, nullable types allow variables to hold null values. To ensure null safety, you can use safe calls (?.), the Elvis operator (?:), or non-null assertions (!!).
Q3. Write a Kotlin function that takes a list of integers and returns the sum of all even numbers.
fun sumEvenNumbers(numbers: List<Int>): Int { return numbers.filter { it % 2 == 0 }.sum() }
Q4. What are sealed classes in Kotlin, and how are they useful in modeling hierarchies?
A: Sealed classes restrict inheritance to a set of predefined subclasses. They are useful for modeling finite hierarchies where all possible subclasses are known.
Q5. How does Kotlin handle extension functions, and what are their benefits?
A: Extension functions allow you to augment existing classes with new methods without altering their source code. This promotes cleaner, more organized code and improved reusability.
Q6. Write a Kotlin data class for representing a user profile with properties like name, age, and email.
data class UserProfile(val name: String, val age: Int, val email: String)
Q7. Explain the usage of the when
expression in Kotlin and provide an example.
A: The when
expression is akin to a versatile switch statement. It evaluates an expression and matches it against various cases.
fun evaluateGrade(score: Int): String { return when (score) { in 90..100 -> "A" in 80 until 90 -> "B" in 70 until 80 -> "C" else -> "F" } }
Q8. How do you perform network requests asynchronously using Kotlin Coroutines?
A: Kotlin Coroutines facilitate asynchronous programming by allowing you to write sequential code while handling asynchronous tasks concurrently. Here’s a basic example of network request using Coroutines:
suspend fun fetchUserData(): User { return withContext(Dispatchers.IO) { // Perform network request // Return user data } }
Q9. Implement a custom Kotlin property delegate for lazy initialization.
class LazyInit<T>(private val initializer: () -> T) { private var value: T? = null operator fun getValue(thisRef: Any?, property: KProperty<*>): T { return value ?: initializer().also { value = it } } }
Q10. Describe the Kotlin let
, apply
, run
, and with
scope functions and provide examples of their usage.
A: These scope functions facilitate concise and expressive code.
let
: Use it to perform operations on a non-null object.
val nameLength = name?.let { it.length } ?: 0
apply
: Employ it for initializing properties of an object.
val person = Person().apply { name = "John" age = 30 }
run
: Leverage it to execute operations on an object and return the result.
val total = shoppingCart.run { calculateTotal() }
with
: Use it to execute operations on an object without the need for the object name.
val average = with(scores) { sum() / size }
Q11. Write a Kotlin lambda expression that filters a list of strings to return only those with more than five characters.
val filteredList = strings.filter { it.length > 5 }
Q12. What is dependency injection, and how can it be achieved in a Kotlin project?
A: Dependency injection is a design pattern that enables loose coupling and enhances code maintainability. In Kotlin, dependency injection can be accomplished through frameworks like Koin or Dagger.
Q13. How does Kotlin handle type inference, and what are its benefits?
A: Kotlin employs type inference to deduce the types of variables from their context. This minimizes verbosity and enhances code readability without sacrificing type safety.
Q14. Implement a basic RecyclerView adapter in Kotlin for displaying a list of items.
class ItemAdapter(private val items: List<Item>) : RecyclerView.Adapter<ItemViewHolder>() { override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ItemViewHolder { // Inflate the layout and create a view holder } override fun onBindViewHolder(holder: ItemViewHolder, position: Int) { val item = items[position] holder.bind(item) } override fun getItemCount(): Int = items.size }
Q15. Describe the concept of Kotlin’s data binding and how it’s used in Android development.
A: Kotlin’s data binding is a technique that connects UI components to data sources. It enhances code maintainability by eliminating boilerplate code.
Q16. Write a Kotlin extension function that checks if a given string is a palindrome.
fun String.isPalindrome(): Boolean { val cleanString = this.replace("[^A-Za-z0-9]", "").toLowerCase() return cleanString == cleanString.reversed() }
Q17. Explain the concept of higher-order functions in Kotlin and provide an example.
A: Higher-order functions are functions that accept other functions as arguments or return them.
fun <T> List<T>.customFilter(predicate: (T) -> Boolean): List<T> { val result = mutableListOf<T>() for (item in this) { if (predicate(item)) { result.add(item) } } return result }
Q18. How do you work with lambdas and higher-order functions in Kotlin’s standard library?
A: Kotlin’s standard library is replete with higher-order functions that enhance code modularity and readability. For instance, the map
, filter
, and reduce
functions are invaluable tools.
Q19. What are the benefits of using Kotlin Coroutines for concurrency, compared to traditional thread-based approaches?
A: Kotlin Coroutines offer more lightweight concurrency by efficiently managing threads and reducing context switches. They streamline asynchronous programming and mitigate issues such as callback hell.
Q20. Write a Kotlin class that implements a basic binary search algorithm for a sorted list of integers.
class BinarySearch { fun search(list: List<Int>, target: Int): Int { var left = 0 var right = list.size - 1 while (left <= right) { val mid = left + (right - left) / 2 when { list[mid] == target -> return mid list[mid] < target -> left = mid + 1 else -> right = mid - 1 } } return -1 } }
5. How to Hire Kotlin Developers through CloudDevs
CloudDevs is your ultimate ally in streamlining the process of hiring skilled Kotlin developers:
Step 1: Connect with CloudDevs: Initiate a conversation with a CloudDevs consultant to discuss your project’s specifics, preferred skills, and expected experience.
Step 2: Discover the Perfect Fit: Within a mere 24 hours, CloudDevs will furnish you with a curated selection of pre-screened Kotlin developers. Peruse their profiles and select the candidate who resonates with your project’s vision.
Step 3: Embark on a No-Risk Trial: Engage in a discussion with your chosen Kotlin developer to ensure seamless onboarding. Once content, formalize the collaboration and embark on a week-long free trial.
Leveraging the power of CloudDevs, you can effortlessly identify and engage top-tier Kotlin developers, ensuring your team is fortified with the indispensable skills required to craft stellar applications.
Conclusion
By assimilating the insights offered within this all-encompassing guide and employing the detailed interview questions, you’re poised to expertly evaluate the capabilities of Kotlin developers. Whether you’re crafting innovative Android apps or pioneering backend systems, securing the right Kotlin developers for your team holds the key to your project’s triumph.
Table of Contents
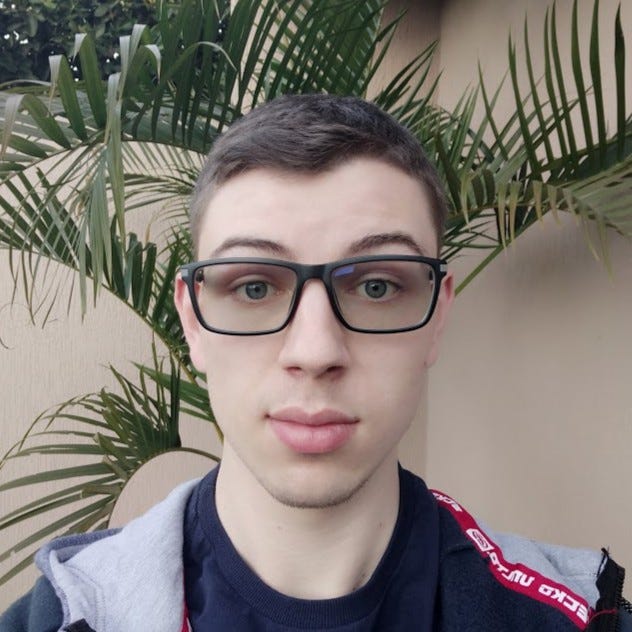
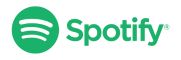