What are *args and **kwargs in Python?
In Python, `*args` and `**kwargs` are special syntaxes used in function signatures to allow a variable number of arguments. They provide flexibility, enabling functions to handle both an unspecified quantity and type of arguments.
- `args`:
– Purpose: It allows a function to accept any number of positional arguments.
– Usage: Within the function, `args` is treated as a tuple, where you can access the passed positional arguments.
For instance, if you have a function that you want to design to accept any number of numerical arguments and return their sum, you’d use `*args`.
– Syntax:
```python def sum_numbers(*args): return sum(args) ```
Calling `sum_numbers(1, 2, 3)` would return `6`.
- `kwargs`:
– Purpose: It permits a function to accept any number of keyword arguments (arguments preceded by a keyword).
– Usage: Within the function, `kwargs` is treated as a dictionary. You can access the passed keyword arguments as key-value pairs.
For example, if you’re creating a function that builds a dictionary from provided keyword arguments, you’d utilize `**kwargs`.
– Syntax:
```python def build_dict(**kwargs): return kwargs ```
Calling `build_dict(name=”Alice”, age=30)` would return `{‘name’: ‘Alice’, ‘age’: 30}`.
Points to Remember:
– When using both `*args` and `**kwargs` in a function signature, `*args` must come before `**kwargs`.
– While `args` and `kwargs` are the conventional names, the real magic lies in the `*` and `**` prefixes. You could technically use other names like `*varargs` or `**keyargs`, but sticking to the convention makes the code more universally understandable.
– These constructs are particularly handy for functions like decorators or wrappers, which might need to accept a wide variety of arguments.
`*args` and `kwargs` offer a powerful way to create flexible functions in Python. They cater to scenarios where the exact number and kind of arguments can’t be predetermined, enhancing the adaptability of your Python functions. Familiarity with these constructs is vital for any Python developer aiming for versatile and dynamic code.
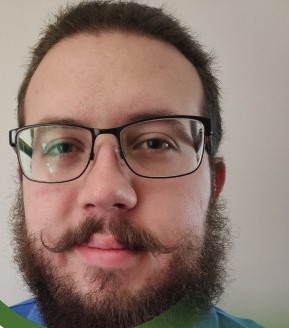
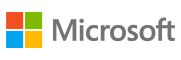