How to connect Python with a database?
Connecting Python to a database is a fundamental operation for applications that require persistent data storage. Python, given its extensive library ecosystem, provides multiple ways to interface with databases, both relational and non-relational.
Relational Databases (e.g., MySQL, PostgreSQL, SQLite):
- SQLite: Python’s standard library includes the `sqlite3` module, allowing for connections to SQLite databases without any additional installations.
```python import sqlite3 conn = sqlite3.connect('example.db') ```
- MySQL and PostgreSQL: For databases like MySQL or PostgreSQL, the `PyMySQL` and `psycopg2` libraries, respectively, are popular choices. You can install them via `pip` and use them to establish connections:
```python # For MySQL import pymysql conn = pymysql.connect(host='localhost', user='username', password='password', database='dbname') # For PostgreSQL import psycopg2 conn = psycopg2.connect(host='localhost', user='username', password='password', dbname='dbname') ```
ORM (Object Relational Mapping):
Tools like SQLAlchemy provide a higher-level, more Pythonic interface to databases. They allow developers to interact with databases using Python classes instead of writing SQL queries directly. ORM tools can simplify complex database operations and are especially useful for applications that target multiple database systems.
Non-Relational Databases (e.g., MongoDB):
For NoSQL databases like MongoDB, Python has libraries like `pymongo`. After installing the library, you can connect to MongoDB:
```python from pymongo import MongoClient client = MongoClient('localhost', 27017) db = client['database_name'] ```
Connection Management:
Regardless of the database or library, always remember to manage your database connections properly. This typically involves:
– Opening the connection as late as possible.
– Closing the connection as soon as possible, typically using context managers (`with` statement) or ensuring closure in a `finally` block.
Python’s vast ecosystem offers various tools and libraries for connecting to databases, whether they are relational or non-relational. It’s essential to choose the appropriate tool based on your application’s requirements and ensure proper connection management for optimal performance and reliability.
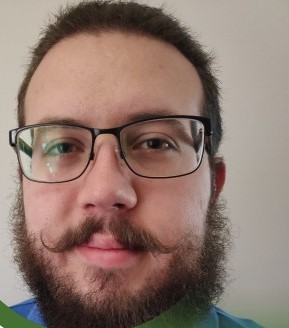
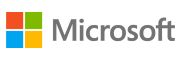