How to copy a list in Python?
Copying lists in Python is a task that appears simple at first but carries subtleties that can lead to unexpected behaviors, especially when dealing with nested lists or lists containing mutable objects. Here’s a comprehensive overview of copying lists in Python:
- Shallow Copy:
– Using the Slice Notation: You can create a copy of a list by slicing it without specifying start and end indices.
```python copied_list = original_list[:] ```
– Using the `list()` Constructor: Another way to achieve a shallow copy is to pass the original list to the `list()` constructor.
```python copied_list = list(original_list) ```
– Using the `copy()` Method**: Lists in Python have a built-in method called `copy()`, which returns a shallow copy of the list.
```python copied_list = original_list.copy() ```
In all these methods, only references to the objects in the original list are copied, not the objects themselves. This means that while the two lists are independent, the objects inside them are shared.
- Deep Copy:
For lists that contain other lists or mutable objects, shallow copying can lead to problems, as changes to the inner lists will reflect in both the original and copied lists. In such cases, you need a deep copy.
– Using the `copy` Module: The `copy` module provides the `deepcopy()` method, which creates a deep copy of the list.
```python import copy copied_list = copy.deepcopy(original_list) ```
With a deep copy, both the list and all the objects inside it are duplicated, ensuring full independence from the original list.
- Potential Pitfalls:
Simply assigning a list to another variable (`new_list = original_list`) doesn’t create a copy but rather a new reference to the original list. Any changes made using either variable will affect the other, as they both point to the same list.
When copying lists in Python, it’s essential to determine whether a shallow copy will suffice or if a deep copy is necessary, especially when working with nested structures or mutable objects. Using the appropriate copying method ensures that lists operate independently, preventing unexpected behaviors and bugs. Understanding the nuances of list copying is vital for robust and error-free Python programming.
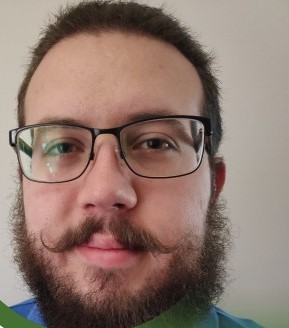
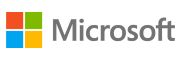