How to create a REST API in Python?
Creating a REST API is a common task in modern web development, and Python offers several frameworks to facilitate this. Here’s a concise overview:
- Selecting a Framework:
Two popular choices in the Python ecosystem for creating RESTful APIs are Flask and Django. While Django is a comprehensive web framework with its built-in support for creating APIs, Flask’s lightweight and modular nature allows for more flexibility and is often paired with extensions like Flask-RESTful.
- Using Flask with Flask-RESTful:
– Begin by installing Flask and Flask-RESTful: `pip install Flask Flask-RESTful`.
– Flask-RESTful simplifies API creation by providing a base `Resource` class from which you can derive custom resource classes. Each class defines methods (`get`, `post`, `put`, `delete`) that correspond to HTTP methods.
– Create an instance of the Flask app and an API object. Add resources to the API by associating endpoint URLs with resource classes.
- Using Django with Django Rest Framework (DRF):
– DRF is a powerful, flexible toolkit that integrates seamlessly with Django, making it easier to build Web APIs.
– Begin by installing Django and DRF: `pip install django djangorestframework`.
– DRF uses serializers to transform data for rendering into JSON, XML, or other content types. It also provides generic class-based views to handle typical actions like list, create, retrieve, update, and delete.
– Set up DRF by adding it to the `INSTALLED_APPS` list in your Django project’s settings. Then define your models, serializers, and views, and finally wire them up in the `urls.py` file.
- Securing Your API:
It’s crucial to implement authentication and permissions if your API exposes sensitive data or functionality. Both Flask-RESTful and DRF provide mechanisms to ensure only authorized clients can access and manipulate data. For instance, DRF integrates with various authentication methods, from simple token-based authentication to more complex OAuth2 implementations.
- Documentation:
Tools like Swagger and DRF’s built-in browsable API make it easier for developers to understand how to interact with your API.
Python provides excellent tools to create RESTful APIs, catering to both those who want out-of-the-box solutions and those seeking more control and flexibility. The choice of framework and tools will depend on the specific needs of the project, but both Flask and Django offer robust and efficient paths to a functional REST API.
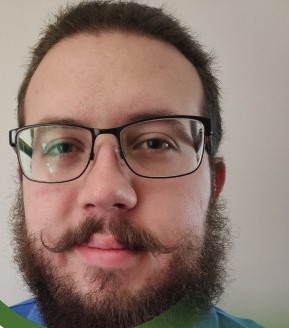
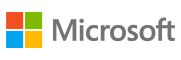