How to Use Python Functions for Cryptography
In today’s digital age, ensuring the security and confidentiality of data is of paramount importance. Whether you’re working with sensitive user information, financial records, or any other form of private data, cryptography is a crucial tool to protect your information from unauthorized access. Python, a versatile and widely-used programming language, offers an array of built-in and third-party functions that make cryptography implementation easier than ever. In this guide, we’ll delve into the world of cryptography using Python functions, covering encryption, decryption, hashing, and more, accompanied by practical code samples.
Table of Contents
1. Introduction to Cryptography with Python
Cryptography involves transforming data into a secure format to prevent unauthorized access. Python, with its extensive libraries and user-friendly syntax, provides a solid foundation for implementing various cryptographic techniques. Whether you’re a beginner or an experienced developer, understanding cryptography with Python can greatly enhance your ability to protect sensitive information.
2. Symmetric Encryption and Decryption
Symmetric cryptography, also known as secret-key cryptography, employs a single secret key for both encryption and decryption processes. The cryptography library in Python offers robust support for symmetric encryption algorithms such as AES (Advanced Encryption Standard).
2.1. Utilizing the cryptography Library
To get started with symmetric encryption, you’ll need to install the cryptography library if you haven’t already:
bash pip install cryptography
Next, let’s explore a code example demonstrating symmetric encryption and decryption using AES:
2.2. Code Example: Implementing Symmetric Encryption and Decryption
python from cryptography.fernet import Fernet # Generate a random symmetric key key = Fernet.generate_key() cipher_suite = Fernet(key) # Message to be encrypted message = b"Confidential information!" # Encrypt the message cipher_text = cipher_suite.encrypt(message) print("Cipher Text:", cipher_text) # Decrypt the message plain_text = cipher_suite.decrypt(cipher_text) print("Decrypted Text:", plain_text.decode())
In this example, the Fernet class from the cryptography library is used to generate a random symmetric key and perform encryption and decryption operations. The encrypted message, referred to as cipher text, is produced in bytes and can be decrypted using the same key to retrieve the original message.
3. Asymmetric Encryption and Decryption
Asymmetric cryptography involves a pair of keys: a public key for encryption and a private key for decryption. The cryptography library supports various asymmetric encryption algorithms like RSA (Rivest-Shamir-Adleman).
3.1. Generating Key Pairs
Creating key pairs is a fundamental step in asymmetric encryption. Let’s see how to generate key pairs and perform encryption and decryption using RSA:
3.2. Code Example: Applying Asymmetric Encryption and Decryption
python from cryptography.hazmat.primitives import serialization from cryptography.hazmat.primitives.asymmetric import rsa, padding # Generate RSA key pair private_key = rsa.generate_private_key( public_exponent=65537, key_size=2048 ) public_key = private_key.public_key() # Message to be encrypted message = b"Confidential information!" # Encrypt the message cipher_text = public_key.encrypt( message, padding.OAEP( mgf=padding.MGF1(algorithm=hashes.SHA256()), algorithm=hashes.SHA256(), label=None ) ) print("Cipher Text:", cipher_text) # Decrypt the message plain_text = private_key.decrypt( cipher_text, padding.OAEP( mgf=padding.MGF1(algorithm=hashes.SHA256()), algorithm=hashes.SHA256(), label=None ) ) print("Decrypted Text:", plain_text.decode())
In this code example, the RSA key pair is generated using the rsa.generate_private_key method. The OAEP (Optimal Asymmetric Encryption Padding) scheme is employed for secure encryption and decryption.
4. Hashing Techniques for Data Integrity
Hashing is a one-way transformation that converts input data into a fixed-size hash value. It’s commonly used to verify data integrity and create digital signatures. Python’s built-in hashlib library provides access to various hash functions.
4.1. The hashlib Library for Hash Functions
To begin using hash functions in Python, no additional installation is required. The hashlib library is readily available. Here’s how you can hash data using SHA-256:
4.2. Code Example: Hashing Data with Python
python import hashlib # Data to be hashed data = b"Hello, world!" # Create a SHA-256 hash object hash_object = hashlib.sha256() # Update hash object with data hash_object.update(data) # Get the hash value hash_value = hash_object.hexdigest() print("SHA-256 Hash:", hash_value)
In this example, the data “Hello, world!” is hashed using the SHA-256 algorithm. The resulting hash value is a fixed-length hexadecimal representation of the input data.
5. Digital Signatures for Authentication
Digital signatures provide a means to ensure the authenticity and integrity of messages. They involve the private key of the sender to sign the message and the recipient’s public key to verify the signature.
5.1. Generating Digital Signatures
Here’s how you can generate and verify digital signatures using Python’s cryptography tools:
5.2. Code Example: Creating and Verifying Digital Signatures
python from cryptography.hazmat.primitives import hashes from cryptography.hazmat.primitives.asymmetric import padding # Generate RSA key pair private_key = rsa.generate_private_key( public_exponent=65537, key_size=2048 ) public_key = private_key.public_key() # Message to be signed message = b"Important message to be signed!" # Create a signature signature = private_key.sign( message, padding.PSS( mgf=padding.MGF1(hashes.SHA256()), salt_length=padding.PSS.MAX_LENGTH ), hashes.SHA256() ) print("Signature:", signature) # Verify the signature try: public_key.verify( signature, message, padding.PSS( mgf=padding.MGF1(hashes.SHA256()), salt_length=padding.PSS.MAX_LENGTH ), hashes.SHA256() ) print("Signature is valid.") except InvalidSignature: print("Signature is not valid.")
In this code example, the sender signs the message using their private key and the recipient verifies the signature using the sender’s public key. This process ensures the authenticity and integrity of the message.
6. Practical Tips for Secure Cryptography Implementation
- Key Management: Ensure secure storage and management of cryptographic keys to prevent unauthorized access.
- Randomness: Use proper random number generators for generating keys and initialization vectors to enhance security.
- Algorithm Selection: Choose well-established and secure encryption algorithms. Avoid using custom encryption methods.
- Secure Communication: When transmitting keys or encrypted data, use secure channels like HTTPS to prevent interception.
- Regular Updates: Keep your cryptography libraries and tools updated to benefit from security patches and improvements.
Conclusion
Implementing cryptography in your Python projects is essential for safeguarding sensitive data and communications. With the built-in and third-party libraries available, you can easily integrate various cryptographic techniques such as symmetric and asymmetric encryption, hashing, and digital signatures. By following best practices and staying updated on security trends, you’ll be well-equipped to create robust and secure applications that protect your data from potential threats in the digital landscape.
Table of Contents
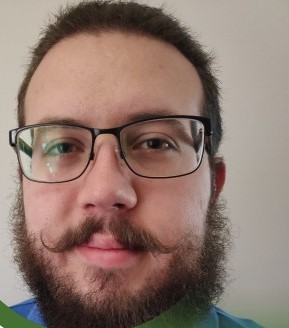
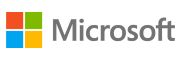