Understanding List Comprehension with Python
In the realm of Python programming, efficiency and readability go hand in hand. One technique that beautifully marries these two aspects is list comprehension. If you’re looking to streamline your code while maintaining its clarity, list comprehension is a tool you should master. In this blog, we’ll dive deep into the world of list comprehension, uncovering its nuances and showcasing its practical application through code examples.
Table of Contents
1. Introduction to List Comprehension
1.1. What is List Comprehension?
List comprehension is a concise and elegant way to create lists in Python. It allows you to generate a new list by applying an expression to each item in an existing iterable, such as a list, tuple, or string, and optionally filtering the results based on certain conditions. This powerful technique not only reduces the lines of code you need to write but also enhances the readability of your programs.
1.2. Advantages of List Comprehension
List comprehension offers several advantages that make it a popular choice among Python developers:
- Conciseness: With list comprehension, you can achieve in a single line what might take multiple lines using traditional loops.
- Readability: The compact syntax of list comprehension makes it easier to understand the intent of the code, especially for simple operations.
- Performance: In many cases, list comprehension can be faster than traditional loops since it’s optimized internally by Python’s interpreter.
- Functional Programming: List comprehension aligns with the functional programming paradigm, allowing you to think more abstractly about data transformation.
2. Syntax and Basic Structure
The basic syntax of a list comprehension consists of an expression followed by a for loop that iterates over an iterable. The general structure is as follows:
python new_list = [expression for item in iterable]
Let’s break down the components:
- expression: This is the operation you want to perform on each item in the iterable to generate a new value for the new list.
- item: This variable takes on each value from the iterable as the loop iterates through it.
- iterable: This is the collection of items that you’re iterating over, such as a list or a range.
Here’s a simple example to illustrate the syntax:
python numbers = [1, 2, 3, 4, 5] squared_numbers = [num ** 2 for num in numbers] print(squared_numbers) # Output: [1, 4, 9, 16, 25]
In this example, the expression num ** 2 calculates the square of each number in the numbers list.
3. Conditionals in List Comprehension
3.1. Using if Statements
List comprehensions can also incorporate conditional statements to filter elements based on certain criteria. The syntax for adding an if condition is as follows:
python new_list = [expression for item in iterable if condition]
The condition should be a Boolean expression that determines whether the item should be included in the new list. Here’s an example that filters out even numbers:
python numbers = [1, 2, 3, 4, 5] odd_numbers = [num for num in numbers if num % 2 != 0] print(odd_numbers) # Output: [1, 3, 5]
3.2. Using if-else Statements
You can even use if-else statements within list comprehensions to apply different expressions based on the condition. The syntax is slightly modified:
python new_list = [expression_if_true if condition else expression_if_false for item in iterable]
Let’s see an example that categorizes numbers as “Even” or “Odd”:
python numbers = [1, 2, 3, 4, 5] category = ["Even" if num % 2 == 0 else "Odd" for num in numbers] print(category) # Output: ["Odd", "Even", "Odd", "Even", "Odd"]
4. Nested List Comprehension
List comprehensions can also be nested within each other, allowing you to create more complex structures. This is particularly useful when dealing with nested iterables like lists of lists. The syntax for a nested list comprehension is:
python new_list = [[expression for item in inner_iterable] for outer_item in outer_iterable]
Consider an example where you want to flatten a matrix:
python matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] flattened = [num for row in matrix for num in row] print(flattened) # Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
5. Applications and Use Cases
List comprehension is a versatile tool that finds applications in various scenarios. Let’s explore a few use cases:
5.1. Creating Lists of Squares and Cubes
python numbers = [1, 2, 3, 4, 5] squares = [num ** 2 for num in numbers] cubes = [num ** 3 for num in numbers]
5.2. Filtering Even and Odd Numbers
python numbers = [1, 2, 3, 4, 5] even_numbers = [num for num in numbers if num % 2 == 0] odd_numbers = [num for num in numbers if num % 2 != 0]
5.3. Manipulating Strings
python words = ["hello", "world", "python", "list", "comprehension"] capitalized_words = [word.upper() for word in words] word_lengths = [len(word) for word in words]
6. Performance Comparison: List Comprehension vs. Traditional Loops
While list comprehension offers concise and readable code, it’s worth comparing its performance against traditional loop constructs like for and while loops. In many cases, list comprehension can be more efficient due to Python’s internal optimizations.
7. Best Practices for Using List Comprehension
To make the most of list comprehension, keep these best practices in mind:
- Keep It Simple: List comprehensions are best suited for simple operations. For complex tasks, prefer traditional loops or other techniques.
- Prioritize Readability: While brevity is great, code readability is essential. Avoid cramming too much logic into a single line.
- Use Descriptive Names: Meaningful variable names enhance code clarity. Choose names that reflect the purpose of the comprehension.
- Avoid Nested Comprehensions: Complex nested comprehensions can quickly become difficult to understand. Use them sparingly.
Conclusion
List comprehension is a potent weapon in the arsenal of any Python programmer. Its ability to generate new lists with concise syntax while applying filtering conditions is a testament to its elegance and versatility. By mastering list comprehension, you can make your code more efficient, readable, and in line with Python’s ethos of simplicity and beauty. As you continue your coding journey, remember to leverage this tool whenever it’s appropriate, and enjoy the benefits of streamlined code that’s a joy to work with.
Table of Contents
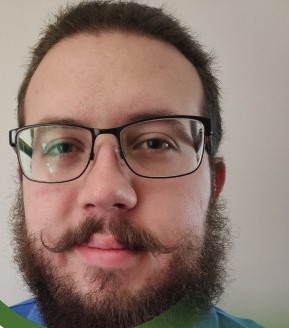
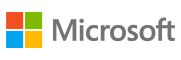