The Power of Decorators in Python: Enhancing Code Through Advanced Techniques
In Python, a decorator is a design pattern that allows the behavior of a function or class to be modified without changing its source code. They are a form of metaprogramming since they modify the behavior of code during runtime. As the term “decorator” suggests, they are used to add features (decorate) functions or classes. Understanding and effectively using decorators is an important skill, whether you’re developing your own Python proficiency or planning to hire Python developers for complex tasks. This knowledge aids in enhancing your Python code and sets the bar when you’re looking to hire Python developers.
How Do Decorators Work?
Before diving into decorators, it’s essential to understand that in Python, functions are first-class objects. This means they can be assigned to variables, passed to other functions as arguments, returned from other functions, and even defined inside other functions.
A decorator in Python is a callable Python object that is used to modify a function, method, or class definition. The original object, the one whose behavior is going to be modified, is passed to a decorator as an argument. The decorator returns a modified object, e.g., a modified function, which is then used in place of the original object.
Basic Decorator Syntax
Let’s illustrate with a simple decorator example:
```python def my_decorator(func): def wrapper(): print("Something is happening before the function is called.") func() print("Something is happening after the function is called.") return wrapper def say_hello(): print("Hello!") say_hello = my_decorator(say_hello) ```
In the example above, `my_decorator` is a decorator. It’s a function that takes another function as its argument (`say_hello` in this case), and returns a new function that alters the behavior of the original function.
However, Python provides a convenient syntax for applying decorators using the @ symbol:
```python @my_decorator def say_hello(): print("Hello!") ```
This code does exactly the same thing as the previous example. The `@my_decorator` line is equivalent to `say_hello = my_decorator(say_hello)`.
Common Applications of Decorators
- Logging and Timing: Decorators can add quick logging or timing code to your functions:
```python import time def timer_decorator(func): def wrapper(*args, **kwargs): start_time = time.time() result = func(*args, **kwargs) end_time = time.time() print(f"Function {func.__name__} took {end_time - start_time} seconds to run.") return result return wrapper @timer_decorator def long_running_function(): # Some time-consuming operations here time.sleep(2) long_running_function() ```
In this example, the `timer_decorator` adds timing functionality to the `long_running_function`. It calculates and prints the time taken for the function to execute.
- Authorization and Authentication: Decorators can control access to functions based on certain conditions, typically used for user roles and permissions:
```python def admin_required(func): def wrapper(user, *args, **kwargs): if user.is_admin: return func(user, *args, **kwargs) else: raise PermissionError("Admin privileges are required to run this function.") return wrapper @admin_required def admin_function(user): print("Admin function executed!") # Where 'user' is an object with a property 'is_admin' ```
In this example, the `admin_required` decorator checks if a user is an admin before executing the function. If the user is not an admin, it raises a PermissionError.
- Caching/Memoization: Decorators can be used to store the results of expensive function calls and reuse them when the same inputs occur:
```python def memoize_decorator(func): cache = dict() def wrapper(*args, **kwargs): key = (args, fro zenset(kwargs.items())) if key in cache: return cache[key] result = func(*args, **kwargs) cache[key] = result return result return wrapper @memoize_decorator def expensive_function(): # Some expensive computation here pass ```
In this example, the `memoize_decorator` stores the result of `expensive_function` in a dictionary. If the function is called again with the same arguments, the decorator returns the cached result instead of calling the function again.
Conclusion
Decorators offer a powerful way to modify or enhance the behavior of a function, method, or class, without altering its source code. They are commonly used for logging, authorization, and caching, among other things. Understanding and utilizing decorators is not only crucial for efficient coding, but it’s also a skill you should look for when you hire Python developers. While decorators can be a bit challenging to understand at first, they become an invaluable tool once you’re comfortable with them. They provide a clean, elegant way to augment functionality, helping to make your Python code more readable, maintainable, and DRY (Don’t Repeat Yourself). This understanding can significantly enhance your ability to hire Python developers who can deliver efficient, maintainable code.
Table of Contents
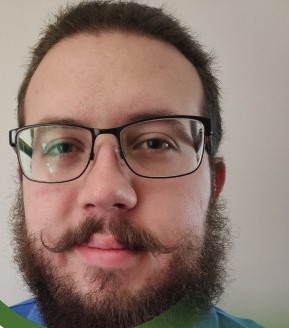
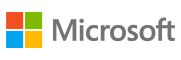