Python Q & A
What is functional programming in Python?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state or mutable data. Python, while primarily procedural and object-oriented, offers capabilities that facilitate functional programming. Here’s a brief overview of functional programming in Python:
- First-Class Functions: In Python, functions are first-class citizens, meaning they can be passed around as arguments, returned as values, and assigned to variables. This characteristic is fundamental to functional programming.
- Immutable Data: Functional programming promotes data immutability. While Python doesn’t enforce this strictly, data types like tuples and strings are immutable, encouraging a functional approach.
- Higher-Order Functions: Python provides built-in higher-order functions like `map()`, `filter()`, and `reduce()`. These functions take other functions as parameters, allowing you to process data in a functional style. For instance, `map()` applies a function to each item in an iterable.
- Lambda Functions: Lambda functions are small anonymous functions defined using the `lambda` keyword. They’re handy for short operations that can be described in a single statement, particularly as arguments to higher-order functions.
- List Comprehensions: List comprehensions offer a concise way to create lists based on existing lists. They align with functional programming by emphasizing expression evaluation over the loop’s procedural nature.
- Pure Functions: A hallmark of functional programming, pure functions, are those that have no side effects and return a value that depends only on their arguments. While Python doesn’t enforce this, you can write pure functions to align with functional principles.
- Recursion: Functional programming often uses recursion as a primary control structure instead of loops. Python supports recursion, but due to its recursion limit, it’s used judiciously.
- Functional Libraries: Libraries like `functools` enhance Python’s functional programming capabilities by offering tools like `partial` (for partial function application) and `lru_cache` (for memoization).
While Python isn’t a purely functional language, it offers features and tools that allow developers to employ functional programming paradigms when it benefits the problem at hand. Adopting a functional approach can lead to cleaner, more readable, and more parallelizable code.
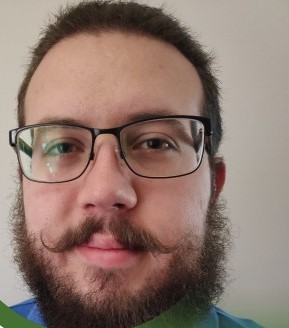
Previously at
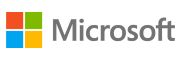
Senior Software Engineer with 7+ yrs Python experience. Improved Kafka-S3 ingestion, GCP Pub/Sub metrics. Proficient in Flask, FastAPI, AWS, GCP, Kafka, Git