How to Use Python Functions to Create GUI Applications
Graphical User Interfaces (GUIs) play a pivotal role in modern software development. They provide an intuitive and user-friendly way for users to interact with applications. Python, being a versatile programming language, allows developers to create GUI applications effortlessly. In this tutorial, we’ll explore how to use Python functions to build interactive GUI applications using popular libraries like Tkinter and PyQt. Whether you’re a beginner or an experienced developer, understanding the process of creating GUIs with Python functions will open up new possibilities for your projects.
1. Understanding Python Functions:
Before diving into GUI development, let’s briefly review Python functions. Functions are blocks of reusable code that perform a specific task and can be called multiple times. They help modularize code, making it easier to read, maintain, and debug.
Defining Functions in Python:
To create a function in Python, use the def keyword followed by the function name and parentheses. You can pass parameters into functions and return values using the return statement. Here’s an example:
python def greet_user(name): return f"Hello, {name}!" user_name = "Alice" print(greet_user(user_name)) # Output: "Hello, Alice!"
2. Introduction to GUI Programming:
Graphical User Interface (GUI) programming focuses on creating visually interactive applications. GUIs use graphical elements like buttons, menus, and text boxes to enable user interaction with the software. They provide a more intuitive experience compared to text-based interfaces.
Advantages of GUI Applications:
- Improved User Experience: GUIs are more user-friendly, allowing users to perform actions through visual elements.
- Faster Learning Curve: GUIs simplify the navigation and interaction, reducing the learning curve for users.
- Enhanced Aesthetics: GUIs allow developers to design visually appealing interfaces, enhancing the application’s overall look.
Popular GUI Libraries in Python:
Python offers various GUI libraries to choose from, depending on the complexity and requirements of your project. Two widely used libraries are:
- Tkinter: Tkinter is Python’s standard GUI library. It provides a set of tools for creating simple and straightforward GUI applications.
- PyQt: PyQt is a powerful and flexible GUI library, offering more features and customization options than Tkinter.
3. Getting Started with Tkinter:
In this section, we’ll focus on Tkinter, the default choice for many Python GUI developers due to its simplicity and ease of use.
Installing Tkinter:
Tkinter comes pre-installed with Python, so there’s no additional installation needed. If you’re using Python 3, import it using:
python import tkinter as tk
Creating the Main Application Window:
To begin building a Tkinter-based GUI, you need a main application window. Here’s how to create one:
python import tkinter as tk app = tk.Tk() app.title("My GUI Application") app.geometry("400x300") app.mainloop()
Adding Widgets and Components:
Tkinter offers various widgets like labels, buttons, entry fields, and more. Let’s add a label and a button to the main window:
python import tkinter as tk def greet_user(): name = entry.get() greeting_label.config(text=f"Hello, {name}!") app = tk.Tk() app.title("My GUI Application") app.geometry("400x300") # Widgets entry = tk.Entry(app) entry.pack() greet_button = tk.Button(app, text="Greet", command=greet_user) greet_button.pack() greeting_label = tk.Label(app, text="") greeting_label.pack() app.mainloop()
In this example, the greet_user function is called when the “Greet” button is clicked. It reads the user’s input from the entry field and displays a personalized greeting on the label.
4. Building a Simple To-Do List Application:
Designing the User Interface:
To create the To-Do List application, we need a text entry field to input tasks, a “Add” button to add tasks to the list, and a listbox to display the tasks. Additionally, we can include “Mark as Completed” and “Delete” buttons to manage tasks in the list.
python import tkinter as tk def add_task(): task = entry.get() if task: tasks_listbox.insert(tk.END, task) entry.delete(0, tk.END) def mark_as_completed(): selected_task_index = tasks_listbox.curselection() if selected_task_index: tasks_listbox.itemconfig(selected_task_index, {'fg': 'gray'}) def delete_task(): selected_task_index = tasks_listbox.curselection() if selected_task_index: tasks_listbox.delete(selected_task_index) app = tk.Tk() app.title("To-Do List Application") app.geometry("400x300") # Widgets entry = tk.Entry(app) entry.pack() add_button = tk.Button(app, text="Add Task", command=add_task) add_button.pack() tasks_listbox = tk.Listbox(app, selectmode=tk.SINGLE) tasks_listbox.pack() mark_button = tk.Button(app, text="Mark as Completed", command=mark_as_completed) mark_button.pack() delete_button = tk.Button(app, text="Delete Task", command=delete_task) delete_button.pack() app.mainloop()
Implementing Functionality with Python Functions:
In this application, the add_task, mark_as_completed, and delete_task functions handle the corresponding actions. When the “Add Task” button is pressed, the add_task function reads the task from the entry field and adds it to the listbox. The “Mark as Completed” button executes the mark_as_completed function, which changes the text color of the selected task to gray. Finally, the “Delete Task” button runs the delete_task function to remove the selected task from the listbox.
5. Taking GUIs to the Next Level with PyQt:
While Tkinter is great for simple applications, PyQt offers more advanced features and customization options. Let’s explore how to use PyQt to build a calculator application.
Introduction to PyQt:
PyQt is a set of Python bindings for the Qt application framework. It provides a comprehensive set of tools for creating cross-platform GUI applications with an excellent range of widgets, graphics views, and multimedia features.
Installing PyQt:
To use PyQt, you need to install it first. Use the following command to install PyQt5:
bash pip install PyQt5
Building a Calculator Application:
Here’s a simple calculator application using PyQt:
python import sys from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QVBoxLayout, QLineEdit, QPushButton class CalculatorApp(QMainWindow): def __init__(self): super().__init__() self.setWindowTitle("Calculator") self.setGeometry(100, 100, 300, 300) self.central_widget = QWidget(self) self.setCentralWidget(self.central_widget) self.layout = QVBoxLayout(self.central_widget) self.result_display = QLineEdit(self) self.layout.addWidget(self.result_display) button_grid = [ ['7', '8', '9', '/'], ['4', '5', '6', '*'], ['1', '2', '3', '-'], ['0', '.', '=', '+'] ] for row in button_grid: button_row = QWidget(self) button_row_layout = QVBoxLayout(button_row) for btn_text in row: button = QPushButton(btn_text, self) button.clicked.connect(self.handle_button_click) button_row_layout.addWidget(button) self.layout.addWidget(button_row) self.current_input = '' self.current_operator = '' self.result_display.setText('0') def handle_button_click(self): button = self.sender() button_text = button.text() if button_text.isdigit() or button_text == '.': self.current_input += button_text self.result_display.setText(self.current_input) elif button_text in ['/', '*', '-', '+']: self.current_operator = button_text self.current_input += ' ' + button_text + ' ' self.result_display.setText(self.current_input) elif button_text == '=': try: result = eval(self.current_input) self.result_display.setText(str(result)) except Exception as e: self.result_display.setText("Error") print(e) finally: self.current_input = '' else: self.current_input = '' self.current_operator = '' self.result_display.setText('0') if __name__ == "__main__": app = QApplication(sys.argv) window = CalculatorApp() window.show() sys.exit(app.exec_())
6. Best Practices for Python GUI Applications:
As you venture into GUI application development with Python, keep in mind these best practices:
Organizing Code with Functions:
Use functions to modularize your code and keep it organized. Separating different functionalities into functions makes it easier to maintain and understand your codebase.
Designing Responsive and Intuitive Interfaces:
A good GUI design is essential for user satisfaction. Ensure that your interface is intuitive, responsive, and easy to navigate.
Error Handling in GUI Applications:
Implement proper error handling to catch and gracefully handle exceptions. This will prevent application crashes and provide a better user experience.
Conclusion:
In this tutorial, we explored the world of GUI application development with Python functions. We started by understanding Python functions, then delved into building interactive GUI applications using Tkinter and PyQt.
Remember, GUI applications bring immense value to software projects, whether you’re creating simple to-do lists or complex multimedia applications. The possibilities are vast, and Python’s versatility combined with its GUI libraries makes it an excellent choice for GUI development.
So, take your Python skills to the next level and start creating engaging and user-friendly GUI applications using functions and Python’s powerful GUI libraries. Happy coding!
Table of Contents
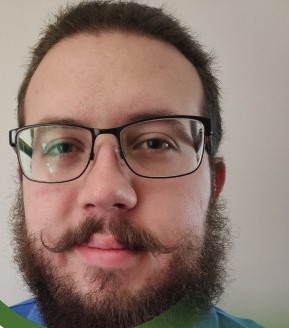
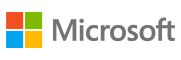