How to handle sessions and cookies in Python web apps?
Handling sessions and cookies is crucial for maintaining state and personalizing user experiences in web applications. In Python, various web frameworks provide utilities for working with them. Here’s a general overview focusing on Flask, a popular Python web framework:
- Cookies: Cookies are small pieces of data stored on the client side, sent to the server with each HTTP request.
– Setting Cookies: In Flask, after creating a response object, use the `set_cookie()` method to set a cookie.
```python response = make_response("Hello, World!") response.set_cookie("username", "JohnDoe") ```
– Reading Cookies: Use the `request.cookies` dictionary to access cookies.
```python username = request.cookies.get("username") ```
- Sessions: Sessions are more secure than cookies for storing sensitive data since they reside server-side. In Flask, the session object lets you store and retrieve data across requests.
– Configuring Sessions: First, set a secret key for your application. This ensures the client-side session cookie’s integrity.
```python app.secret_key = "your_secret_key" ```
– Using Sessions: The `session` object in Flask acts like a Python dictionary.
```python # Set session data session["username"] = "JohnDoe" # Retrieve session data username = session.get("username") ```
- Session Expiry and Persistence: You can set the duration for which a session remains valid. In Flask, this can be done using the `PERMANENT_SESSION_LIFETIME` configuration. To make sessions persistent across browser restarts, set the session’s `permanent` attribute to `True`.
- Security: It’s essential to ensure the confidentiality and integrity of sessions and cookies.
– Use HTTPS: Always serve your web applications over HTTPS to protect cookie data during transit.
– HttpOnly and Secure Flags: In Flask, the session cookie is set with both the `HttpOnly` and `Secure` flags by default, preventing client-side scripts from accessing the cookie and ensuring it’s sent only over HTTPS connections, respectively.
While handling sessions and cookies in Python web applications, prioritize security. Always validate and sanitize data stored in sessions or cookies and use them judiciously based on the sensitivity of the data.
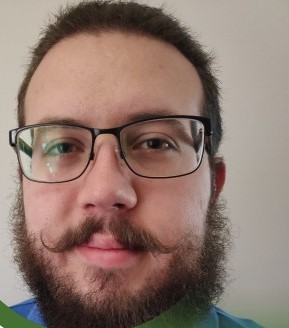
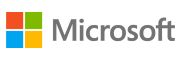