Python Tutorial: Understanding the Inheritance and Polymorphism
In the world of object-oriented programming, two crucial concepts that facilitate code organization, reusability, and flexibility are inheritance and polymorphism. These concepts are the cornerstones of building robust and maintainable software systems. In this Python tutorial, we’ll dive deep into the realm of inheritance and polymorphism, uncovering their significance and demonstrating how to implement them effectively in your Python code.
Table of Contents
1. Understanding Inheritance
1.1. Introduction to Inheritance
Inheritance is a fundamental concept in object-oriented programming that allows a new class to inherit properties and behaviors from an existing class. The existing class is referred to as the base class or parent class, while the new class is known as the derived class or child class. This relationship establishes an “is-a” connection between the two classes, where the derived class inherits attributes and methods from the base class.
1.2. Base and Derived Classes
Let’s illustrate this with an example. Consider a scenario where you’re building a software application for a zoo. You might have a base class called Animal, which contains common attributes and methods shared by all animals. The derived classes, such as Lion, Elephant, and Giraffe, would inherit from the Animal class, gaining its characteristics while also having the flexibility to define their specific attributes and methods.
python class Animal: def __init__(self, name): self.name = name def speak(self): pass # Abstract method class Lion(Animal): def speak(self): return "Roar!" class Elephant(Animal): def speak(self): return "Trumpet!" class Giraffe(Animal): def speak(self): return "Neck stretch!"
In this example, the Lion, Elephant, and Giraffe classes inherit the name attribute and the speak() method from the Animal class.
1.3. Inheriting Attributes and Methods
Inheritance enables you to extend and customize existing classes without modifying their original implementation. The derived class can override methods from the base class to provide specialized behavior. Additionally, it can define new attributes and methods that are unique to the derived class.
2. Polymorphism in Python
2.1. Exploring Polymorphism
Polymorphism is another critical concept in object-oriented programming that allows objects of different classes to be treated as objects of a common superclass. This concept enhances flexibility and code reusability by enabling you to write code that can work with objects of different types in a seamless manner.
In Python, polymorphism is often achieved through duck typing and method overriding.
2.2. Duck Typing
Duck typing is a concept that focuses on the behavior of an object rather than its type. The phrase “If it looks like a duck, swims like a duck, and quacks like a duck, then it probably is a duck” captures the essence of duck typing. In other words, as long as an object supports the required methods and attributes, it can be treated as if it belongs to a specific type.
python def animal_sound(animal): return animal.speak() lion = Lion("Simba") elephant = Elephant("Dumbo") giraffe = Giraffe("Geoffrey") print(animal_sound(lion)) # Output: "Roar!" print(animal_sound(elephant)) # Output: "Trumpet!" print(animal_sound(giraffe)) # Output: "Neck stretch!"
In this example, the animal_sound() function works with different types of animals, demonstrating the essence of duck typing.
2.3. Method Overriding
Method overriding is the practice of providing a new implementation for a method that is already defined in the base class. This allows the derived class to customize or extend the behavior of the inherited method. When a method is called on an object of the derived class, the overridden method in the derived class is executed instead of the method in the base class.
python class Shape: def area(self): pass # Abstract method class Circle(Shape): def __init__(self, radius): self.radius = radius def area(self): return 3.14 * self.radius ** 2 class Rectangle(Shape): def __init__(self, width, height): self.width = width self.height = height def area(self): return self.width * self.height circle = Circle(5) rectangle = Rectangle(4, 6) print(circle.area()) # Output: 78.5 print(rectangle.area()) # Output: 24
In this example, the Circle and Rectangle classes override the area() method from the base class Shape to provide their own area calculation logic.
3. Benefits of Inheritance and Polymorphism
3.1. Code Reusability
Inheritance and polymorphism promote code reusability by allowing you to create a base class with common attributes and methods, which can be inherited by multiple derived classes. This reduces redundant code and ensures consistent behavior across related classes.
3.2. Flexibility and Extensibility
With inheritance, you can extend existing classes to create new ones without modifying the original code. This flexibility is particularly useful when you want to add new features or modify behavior without affecting the entire codebase.
3.3. Organized Code Structure
Using inheritance and polymorphism leads to a more organized code structure. Grouping related classes under a common hierarchy makes the codebase easier to understand and maintain.
4. Implementing Inheritance and Polymorphism
4.1. Creating Base and Derived Classes
To create a derived class, simply define it using the class keyword and provide the base class as an argument in the class definition.
python class Parent: # Parent class attributes and methods class Child(Parent): # Child class attributes and methods
4.2. Overriding Methods
To override a method from the base class, define a method with the same name in the derived class. Make sure the method signature (including parameters) matches that of the base class method.
python class Parent: def greet(self): print("Hello from Parent") class Child(Parent): def greet(self): print("Hello from Child")
4.3. Achieving Polymorphism
To achieve polymorphism, create functions or methods that can accept objects of different classes as parameters. As long as the objects share a common base class or interface, they can be treated interchangeably.
python def introduce(entity): entity.introduce() class Person: def introduce(self): print("Hello, I am a person.") class Dog: def introduce(self): print("Woof! I am a dog.") alice = Person() fido = Dog() introduce(alice) # Output: "Hello, I am a person." introduce(fido) # Output: "Woof! I am a dog."
Conclusion
Understanding inheritance and polymorphism is essential for mastering object-oriented programming in Python. These concepts empower you to build modular, extensible, and efficient software systems. By leveraging the “is-a” relationship between classes, you can create organized code structures and achieve seamless interaction between diverse objects. With the ability to extend classes and override methods, you’re equipped to adapt and tailor your code to meet evolving requirements. So go ahead, apply these principles to your projects, and unlock the true potential of object-oriented Python programming. Happy coding!
Table of Contents
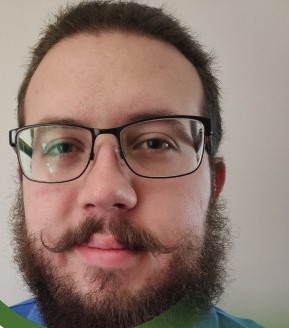
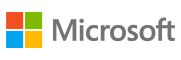