10 Python Libraries for Natural Language Processing
Natural Language Processing (NLP) is a subfield of artificial intelligence that deals with the interaction between computers and human language. It enables machines to understand, interpret, and generate human language, making it a crucial component in many modern applications such as chatbots, sentiment analysis, language translation, and more.
Python, with its simplicity and extensive library support, has become the language of choice for many NLP practitioners and researchers. In this blog, we will explore the top 10 Python libraries for Natural Language Processing, each offering unique functionalities to process and analyze textual data effectively.
1. NLTK (Natural Language Toolkit)
The Natural Language Toolkit (NLTK) is one of the most popular libraries for NLP in Python. It provides tools and resources for tasks such as tokenization, stemming, part-of-speech tagging, parsing, and more. NLTK also includes a vast collection of corpora, lexical resources, and pre-trained models, making it an excellent choice for beginners and researchers alike.
Example: Tokenization
python import nltk nltk.download('punkt') from nltk.tokenize import word_tokenize text = "Natural Language Processing is fascinating!" tokens = word_tokenize(text) print(tokens)
2. spaCy
spaCy is a fast and efficient NLP library designed for production use. It excels in tasks like named entity recognition, dependency parsing, and sentence segmentation. Its focus on performance and ease of use has made it a popular choice in both academia and industry.
Example: Named Entity Recognition (NER)
python import spacy nlp = spacy.load('en_core_web_sm') text = "Apple Inc. was founded by Steve Jobs." doc = nlp(text) for ent in doc.ents: print(ent.text, ent.label_)
3. Gensim
Gensim is a powerful library for topic modeling and document similarity analysis. It’s designed to handle large text collections efficiently and provides implementations of popular algorithms like Word2Vec and Doc2Vec, which are widely used in word embeddings and document representations.
Example: Word2Vec
python from gensim.models import Word2Vec sentences = [["machine", "learning", "is", "awesome"], ["natural", "language", "processing"]] model = Word2Vec(sentences, min_count=1) vector = model.wv['natural'] print(vector)
4. TextBlob
TextBlob is a user-friendly NLP library built on top of NLTK and Pattern. It offers a simple API for common NLP tasks and provides sentiment analysis, part-of-speech tagging, noun phrase extraction, translation, and more.
Example: Sentiment Analysis
python from textblob import TextBlob text = "TextBlob is a fantastic library for NLP!" blob = TextBlob(text) sentiment = blob.sentiment.polarity print(sentiment)
5. Transformers
Transformers, developed by Hugging Face, is a cutting-edge library for state-of-the-art NLP models like BERT, GPT-3, and more. It offers pre-trained models for various tasks, including text classification, question-answering, language translation, and text generation.
Example: Text Classification with BERT
python from transformers import BertTokenizer, BertForSequenceClassification import torch tokenizer = BertTokenizer.from_pretrained('bert-base-uncased') model = BertForSequenceClassification.from_pretrained('bert-base-uncased') text = "This is a positive example." inputs = tokenizer(text, return_tensors="pt") outputs = model(**inputs) predictions = torch.softmax(outputs.logits, dim=1) print(predictions)
6. Pattern
Pattern is a comprehensive library that provides tools for web mining, NLP, machine learning, and network analysis. It offers functionalities for part-of-speech tagging, sentiment analysis, word inflection, and more.
Example: Part-of-Speech Tagging
python from pattern.en import parse sentence = "Pattern library is useful for NLP tasks." parsed_sentence = parse(sentence, lemmata=True) for word, pos in parsed_sentence.split(): print(f"{word}: {pos}")
7. spaCy-stanza
spaCy-stanza is an extension for spaCy that integrates the Stanza library. Stanza is known for its multilingual support and provides pre-trained models for more than 60 languages. This combination makes spaCy-stanza a great choice for cross-lingual NLP tasks.
Example: Multilingual Named Entity Recognition
python import spacy_stanza nlp = spacy_stanza.load_pipeline('en') text = "Le Louvre is located in Paris." doc = nlp(text) for ent in doc.ents: print(ent.text, ent.label_)
8. Polyglot
Polyglot is another multilingual library that supports over 100 languages. It offers functionalities for text processing, named entity recognition, and language detection.
Example: Language Detection
python from polyglot.detect import Detector text = "Hola, ¿cómo estás?" detector = Detector(text) print(detector.language.code)
9. Flair
Flair is a library that focuses on state-of-the-art contextual embeddings and allows easy integration with other NLP libraries. It supports various embedding models and provides functionalities for text classification, named entity recognition, and more.
Example: Named Entity Recognition with Flair
python from flair.models import SequenceTagger from flair.data import Sentence tagger = SequenceTagger.load('ner') sentence = Sentence("Flair is a powerful NLP library.") tagger.predict(sentence) for entity in sentence.get_spans('ner'): print(entity)
10. PyText
PyText, developed by Facebook, is a library specifically designed for natural language processing tasks in the domain of conversational AI and language understanding. It simplifies the process of building and deploying NLP models at scale.
Example: Text Classification with PyText
python from pytext import data from pytext.config import PyTextConfig from pytext.task import create_task # Load and preprocess data train_data, eval_data, test_data = data.make_data() # Create task task = create_task(config=PyTextConfig, task_name='classification') # Train the model task.train(train_data=train_data, eval_data=eval_data)
Conclusion
These ten Python libraries for Natural Language Processing cover a wide range of functionalities, from basic text processing to advanced language understanding tasks. Depending on your project requirements and specific use cases, you can choose the library that best fits your needs. Whether you’re a beginner or an experienced NLP practitioner, these libraries will undoubtedly enhance your text analysis and language processing capabilities. Happy coding and exploring the fascinating world of Natural Language Processing with Python!
Table of Contents
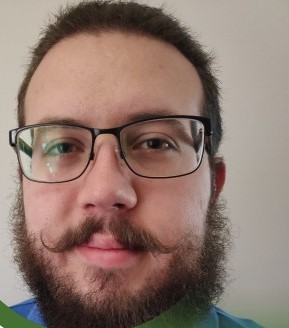
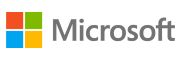