Working with Loops and Conditional Statements in Python
Welcome to this Python tutorial, where we’ll explore two of the most fundamental programming concepts: loops and conditional statements. Familiarizing yourself with these elements is crucial to developing your Python skills and understanding how different program flows work.
Conditional Statements
Conditional statements in Python are used to make decisions based on certain conditions. The program checks if a specific condition is met and decides the next course of action. The key statements used are `if`, `else`, and `elif`.
The If Statement
In Python, an `if` statement is written as follows:
if condition: # code to execute if the condition is true
For instance, consider a program that checks if a number is greater than 10:
num = 15 if num > 10: print("The number is greater than 10")
The If-Else Statement
The `else` statement is used alongside the `if` statement. It defines what the program should do if the condition in the `if` statement is not met.
if condition: # code to execute if the condition is true else: # code to execute if the condition is false
Using our previous example, we can add an `else` clause:
num = 7 if num > 10: print("The number is greater than 10") else: print("The number is not greater than 10")
The Elif Statement
The `elif` statement (short for “else if”) allows us to check multiple conditions. It follows the `if` or another `elif` statement:
if condition1: # code to execute if condition1 is true elif condition2: # code to execute if condition2 is true else: # code to execute if neither condition1 nor condition2 is true
For example:
num = 10 if num > 10: print("The number is greater than 10") elif num == 10: print("The number is exactly 10") else: print("The number is less than 10")
Loops
Loops in Python allow us to execute a block of code multiple times, depending on the condition set. Python has two types of loops: `for` and `while`.
The For Loop
A `for` loop in Python is used for iterating over a sequence (like a list, tuple, dictionary, string, or a set) or other iterable objects.
for value in sequence: # code to execute for each value
For example, here is a loop that iterates over a list of numbers:
numbers = [1, 2, 3, 4, 5] for num in numbers: print(num)
The While Loop
The `while` loop in Python is used to iterate over a block of code as long as the condition is true.
while condition: # code to execute while the condition is true
For example, here is a loop that prints numbers from 1 to 5:
num = 1 while num <= 5: print(num) num += 1
Break and Continue
Sometimes, you might need to change the course of your loop, depending on a certain condition. That’s where `break` and `continue` come in.
– `break`: Stops the loop entirely and executes the next block of code.
for num in range(1, 11): if num == 6: break print(num)
This loop will print numbers from 1 to 5, but it stops before printing 6 because of the `break` statement.
– `continue`: Skips the current iteration and moves to the next.
for num in range(1, 11): if num == 6: continue print(num)
This loop will print numbers from 1 to 10, but it skips 6 due to the `continue` statement.
Conclusion
Understanding loops and conditional statements is foundational to becoming proficient in Python. These concepts allow us to control how and when certain pieces of code are executed, making our programs more efficient and powerful. Happy coding!
Table of Contents
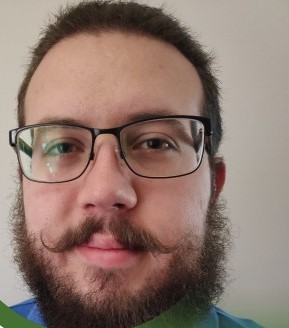
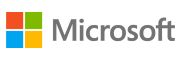