Introduction to Object-Oriented Programming in Python
In the realm of programming, different methodologies are used to structure and organize code. One such approach that has garnered significant recognition is Object-Oriented Programming (OOP). It’s a model that simplifies software development and maintenance by providing principles that guide how a program should be designed and written.
Python, a highly intuitive and flexible programming language, supports multiple programming paradigms, including OOP. In this tutorial, we will take a deep dive into the principles of OOP in Python and explore how you can leverage this powerful model to create more efficient and organized code.
The Core Principles of Object-Oriented Programming
At its heart, OOP is centered around four main principles: encapsulation, inheritance, polymorphism, and abstraction.
- Encapsulation: This principle entails grouping related variables and functions into single units, called objects. In Python, these units are typically created with classes.
- Inheritance: This principle allows one class to inherit the attributes and methods of another, leading to code reusability and efficiency.
- Polymorphism: This principle allows one interface to be used for a general class of actions. It means that the exact method being called is determined at run time based on the calling object.
- Abstraction: This principle is used to hide the complexity of the system and expose only the necessary details to the user.
Let’s learn more about these principles by creating a class in Python.
Understanding Classes and Objects
Classes are the backbone of OOP in Python. They’re essentially user-defined data structures that act as blueprints for creating objects.
To create a class in Python, you use the `class` keyword. Let’s create a simple class called `Car`.
class Car: pass
In this case, `pass` is a placeholder indicating that no action should take place. Now, let’s add some attributes to our class. Attributes are the variables that belong to a class.
class Car: def __init__(self, brand, model, year): self.brand = brand self.model = model self.year = year
The `__init__` function is a special method called a constructor that Python calls when a new instance of the class is created. The `self` keyword is used to access attributes and methods within the class.
Objects are instances of a class. To create an object of the `Car` class, you would do the following:
my_car = Car("Tesla", "Model S", 2020)
Encapsulation
In Python, you can restrict access to methods and variables. This prevents data from direct modification, a concept known as encapsulation. In Python, we denote private attributes using an underscore as a prefix, i.e., `_variable`.
class Car: def __init__(self, brand, model, year): self._brand = brand self._model = model self._year = year
You can provide getter and setter methods to handle these private variables.
class Car: def __init__(self, brand, model, year): self._brand = brand self._model = model self._year = year # Getter method def get_brand(self): return self._brand # Setter method def set_brand(self, brand): self._brand = brand
Inheritance
In Python, a class can inherit attributes and methods from another class. The class from which attributes and methods are inherited is called the parent class, and the class that inherits. Those attributes and methods are called the child class.
Let’s create a child class `ElectricCar` that inherits from the `Car` class.
class ElectricCar(Car): def __init__(self, brand, model, year, battery_size): super().__init__(brand, model, year) self.battery_size = battery_size def describe_battery(self): print(f"This car has a {self.battery_size}-kWh battery.")
Polymorphism
Polymorphism allows us to use a single interface with different underlying forms. For example, the `Car` and `ElectricCar` classes can have a method with the same name, but their tasks are different.
class Car: # rest of the code def start_engine(self): print("Engine starts with a roar!") class ElectricCar(Car): # rest of the code def start_engine(self): print("Engine starts silently!") ```
In the above example, the `start_engine` method acts differently for `Car` and `ElectricCar`.
Abstraction
Abstraction is about hiding complexity and providing a simple interface to the user. In Python, you can create abstract classes and methods using the `abc` module.
from abc import ABC, abstractmethod class Vehicle(ABC): @abstractmethod def mileage(self): pass class Car(Vehicle): def mileage(self): return "This car gives 15 kmpl."
The `Vehicle` class is an abstract class that cannot be instantiated, and the `mileage` method must be implemented in any child class.
Conclusion
In Python, understanding Object-Oriented Programming is key to structuring your code effectively and developing complex software systems. The OOP principles, encapsulation, inheritance, polymorphism, and abstraction, are crucial pillars that guide the structuring and flow of programs in Python.
By creating classes that represent real-world things and situations, and by creating objects based on these classes, you can write more relevant and logical code. The OOP approach to Python offers a clean, efficient, and replicable method of coding, suitable for small scripts and large projects alike.
Table of Contents
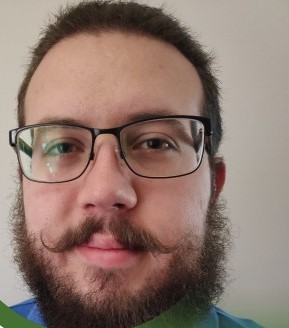
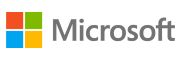