Python Q & A
How to optimize memory usage in Python?
Optimizing memory usage in Python is essential for performance-intensive applications, especially when dealing with large data sets or running on resource-constrained environments. Here are some strategies and best practices to consider:
- Use Built-in Data Types: Utilize Python’s built-in types like sets and tuples wherever appropriate. For instance, a set is more memory-efficient for membership tests than a list.
- Lazy Evaluation with Generators: Instead of using lists, which load all data into memory, you can use generators. Generators yield items one by one using the `yield` keyword and are memory-efficient as they only produce values when needed.
- Strings Optimization: Use interning (`sys.intern()`) for repetitive long strings. It ensures that strings with the same content share the same memory. However, use it judiciously, as it’s not always beneficial for short or unique strings.
- Use `array` for Homogeneous Data: If you have a large list of numbers, consider using the `array` module, which provides a memory-efficient, type-specific container.
- Profiling Memory: Tools like `objgraph` and `memory-profiler` can help you inspect objects’ memory consumption and track memory leaks.
- Optimize Object Structures: If you’re using custom classes, consider using `__slots__` to limit attributes to a predefined set, which can reduce overhead.
- Use Third-Party Libraries: Libraries like `NumPy` and `Pandas` are optimized for memory and performance and can handle large datasets more efficiently than built-in data structures.
- Garbage Collection: Python uses reference counting and a cyclic garbage collector to manage memory. While it’s usually efficient, in specific scenarios, you might benefit from controlling it manually using the `gc` module. For instance, you can disable it temporarily during bulk data processing and enable it afterward.
- Avoid Global Variables: Large global variables can persist throughout the lifetime of your application. Use local variables within functions wherever possible, ensuring they’re garbage collected once out of scope.
Optimizing memory in Python requires a combination of efficient coding practices, judicious use of built-in and third-party tools, and occasionally diving deep into profiling to uncover and resolve bottlenecks.
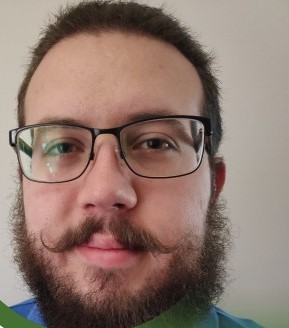
Previously at
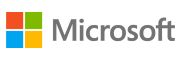
Senior Software Engineer with 7+ yrs Python experience. Improved Kafka-S3 ingestion, GCP Pub/Sub metrics. Proficient in Flask, FastAPI, AWS, GCP, Kafka, Git