Python Techniques for PDF Text Extraction: A Comprehensive Guide
Python is one of the most versatile and popular programming languages out there. Thanks to its massive range of libraries and packages, Python can handle an extensive variety of tasks, including the extraction of text from PDFs. This capability is crucial in numerous contexts, including data analysis, information retrieval, and natural language processing. Owing to the complexity of these tasks, companies often hire Python developers to effectively implement such solutions. In this post, we will explore different methods to use Python functions for extracting text from PDFs, a task Python developers excel at, given the language’s flexibility and power.
Prerequisites
Before we start, make sure that you have Python installed on your computer. If you haven’t, you can download and install it from the [official Python website] (https://www.python.org/).
Additionally, we’ll need some specific Python libraries. You can install these using pip, which is a package manager for Python. Here are the libraries we’ll use:
- PyPDF2
- PDFMiner.six
You can install them with the following commands:
```bash pip install PyPDF2 pip install pdfminer.six ```
Extracting Text Using PyPDF2
PyPDF2 is a pure-python PDF library capable of splitting, merging, and transforming PDF files. It also has a basic text extraction feature.
Let’s see how we can use it:
```python import PyPDF2 def extract_text_pypdf2(filename): pdf_file_obj = open(filename, 'rb') # Open the PDF file in binary mode pdf_reader = PyPDF2.PdfFileReader(pdf_file_obj) # Create a PDF file reader object text = '' for page_num in range(pdf_reader.numPages): # Loop through all the pages page_obj = pdf_reader.getPage(page_num) # Get a specific page text += page_obj.extractText() # Extract the text from the page pdf_file_obj.close() # Close the PDF file return text ```
The function `extract_text_pypdf2` opens the PDF file, creates a reader object, and then loops through each page in the document, extracting the text and appending it to the `text` variable. It finally returns the entire text of the document as a single string.
Note that PyPDF2 may not always be able to correctly extract text, especially if the PDF contains images, tables, or non-standard fonts.
Extracting Text Using PDFMiner.six
PDFMiner.six is another Python library that can be used to extract text from PDFs. It’s a Python 2/3 compatible library for PDF parsing. Unlike PyPDF2, PDFMiner.six allows for detailed examination and extraction of text, including handling of font size, color, and other related properties.
Let’s see how to use it:
```python from pdfminer.high_level import extract_text def extract_text_pdfminer(filename): text = extract_text(filename) # Extract the text from the PDF file return text ```
This is a simpler and more straightforward way to extract text, thanks to PDFMiner’s `extract_text` high-level function.
If you want more control over the extraction process, you can use the lower-level functions in PDFMiner. Here is an example:
```python from pdfminer.converter import TextConverter from pdfminer.layout import LAParams from pdfminer.pdfdocument import PDFDocument from pdfminer.pdfinterp import PDFResourceManager, PDFPageInterpreter from pdfminer.pdfpage import PDFPage from pdfminer.pdfparser import PDFParser from io import StringIO def extract_text_pdfminer_detailed(filename): output_string = StringIO() with open(filename, 'rb') as in_file: parser = PDFParser(in_file) doc = PDFDocument(parser) rsrcmgr = PDFResourceManager() device = TextConverter(rsrcmgr, output_string, laparams=LAParams()) interpreter = PDFPageInterpreter(rsrcmgr, device) for page in PDFPage.create_pages(doc): interpreter.process_page(page) return output_string.getvalue() ```
In this function, `PDFParser` and `PDFDocument` are used to parse the PDF. `PDFResourceManager` is used to store shared resources such as fonts and images. `TextConverter` converts the PDF file’s text content into a string, and `PDFPageInterpreter` processes the page content. The `PDFPage.create_pages` method is used to get the pages, which are then processed by the interpreter.
Conclusion
This post provides a comprehensive yet concise guide to extracting text from PDFs using Python, specifically leveraging the PyPDF2 and PDFMiner.six libraries. While these libraries have unique strengths, they may not perfectly extract text from all PDFs. For handling such complex tasks, businesses can hire Python developers for professional assistance. These developers, with the expansive range of tools provided by Python, can efficiently unlock valuable data hidden inside PDFs for further analysis. So, if you’re grappling with a multitude of PDFs, remember to consider hiring Python developers to optimally harness the power of Python. Happy coding!
Table of Contents
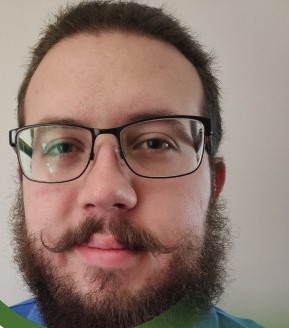
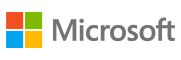