A Hands-On Approach to Python’s Regular Expressions
Regular expressions, also known as regex or regexp, are powerful tools for matching, finding, and replacing text in a string. They are ubiquitous in the realm of programming and offer extensive use in data processing, searching algorithms, data validation, and much more. This critical knowledge is something companies appreciate when they hire Python developers. Python offers the `re` module, which provides a set of functions that allow us to perform these actions using regex. This article is an endeavor to demystify regular expressions in Python with examples, not just to benefit Python developers but also to help those who aim to hire Python developers gain a better understanding of this essential tool.
What is a Regular Expression?
A regular expression is a special sequence of characters that helps you match or find other strings or sets of strings, using a specialized syntax held in a pattern. The Python module ‘re’ provides full support for Perl-like regular expressions.
Basic Metacharacters in Regular Expressions
Some of the basic metacharacters used in regular expressions are:
– `.`: Matches any character except a newline.
– `^`: Matches the start of the string.
– `$`: Matches the end of the string.
– `*`: Matches 0 or more repetitions.
– `+`: Matches 1 or more repetitions.
– `?`: Matches 0 or 1 repetitions.
– `{}`: Indicates a specific number of repetitions.
– `\\`: Escape special characters.
– `[]`: Indicates a set of characters.
– `|`: OR operator.
– `()`: Groups regular expressions and remembers matched text.
Using Regular Expressions in Python
Python’s `re` module allows you to use regular expressions. You can use it in your Python code by importing it using `import re`.
Common `re` Module Functions
– `re.match()`: Determines if the regular expression matches at the beginning of the string.
– `re.search()`: Searches the string for a match to the regular expression, returning a match object, or None if no match was found.
– `re.findall()`: Returns all non-overlapping matches of the regular expression as a list of strings.
– `re.sub()`: Replaces one or many matches with a string.
Examples
`re.match()`
Let’s begin with an example using `re.match()`:
```python import re result = re.match(r'AI', 'AI is fascinating') print(result) ```
In this example, we use `re.match()` to check if ‘AI’ is at the start of the string ‘AI is fascinating’. The `r` at the start of the pattern is a flag for a raw string, which directs Python to interpret the string literally.
`re.search()`
Next, we use `re.search()`:
```python import re result = re.search(r'fascinating', 'AI is fascinating') print(result) ```
In this example, we use `re.search()` to check if ‘fascinating’ is present anywhere in the string ‘AI is fascinating’.
`re.findall()`
Now, we’ll use `re.findall()`:
```python import re result = re.findall(r'\d', 'AI was founded in 2020') print(result) ```
Here, we use `re.findall()` to find all occurrences of any digit in the string ‘AI was founded in 2020’. `\d` is a special sequence that matches any digit.
`re.sub()`
Finally, let’s use `re.sub()`:
```python import re result = re.sub(r'2020', '2023', 'AI was founded in 2020') print(result) ```
In this example, we use `re.sub()` to replace ‘2020’ with ‘2023’ in the string ‘AI was founded in 2020’.
Advanced Usage
Now that we’ve covered the basics, let’s dive into some more advanced uses of regular expressions in Python.
Using Groups
Groups are used by placing the characters to be grouped inside a set of parentheses. Here is an example:
```python import re result = re.search(r'(AI)\s\w+', 'AI is fascinating') print(result) ```
In this example, `(AI)` is a group that matches the string ‘AI’, and `\s\w+` matches a space followed by one or more word characters. The entire regular expression matches ‘AI is’.
Using Quantifiers
Quantifiers specify how many instances of a character, group, or character class must be present in the input for a match to be found. Here’s how to use them:
```python import re result = re.search(r'9{1,3}
Here, `9{1,3}$` matches a string ending with one to three ‘9’s.
Using Special Sequences
`\A`, `\b`, `\B`, `\d`, `\D`, `\s`, `\S`, `\w`, `\W`, `\Z`, etc., are special sequences that can be used in a regex. They make your regular expressions more compact and understandable.
```python import re result = re.search(r'\bAI\b', 'AI is fascinating') print(result) ```
In this example, `\bAI\b` matches ‘AI’, but only when it’s a complete word, not part of another word.
Regular Expressions for Data Science
Regular expressions are a powerful tool for various kinds of string manipulation. They are very useful in ‘cleaning’ the data which is a vital step in the data preparation process in data science and machine learning.
```python import re data = "My phone number is 123-456-7890. Call me at 9 am." phone_num_regex = r'\d{3}-\d{3}-\d{4}' phone_numbers = re.findall(phone_num_regex, data) print(phone_numbers) ```
In the above example, we have used a simple regex pattern to extract phone numbers from a text. This can be particularly useful when dealing with large volumes of text data, where manual extraction would be impractical.
Conclusion
Regular expressions in Python provide a powerful and flexible method for manipulating text. With the right understanding and practice, even those looking to hire Python developers should recognize the immense value in this skill set. Harnessing the power of regular expressions can make data processing tasks more efficient and accurate. This guide is intended to help not just Python developers, but also those aiming to hire Python developers, to better understand the utility of regular expressions in Python. Happy coding!
Table of Contents
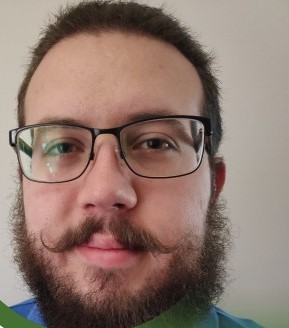
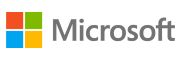