How can I serialize data in Python using JSON?
Serialization is the process of converting complex data structures, such as dictionaries or lists, into a format that can be easily stored or transmitted and later reconstructed. In Python, one of the most popular formats for serialization is JSON (JavaScript Object Notation), due to its lightweight nature and compatibility with many systems.
Serializing Data using JSON in Python:
- Using the `json` Module:
Python’s standard library comes with a module named `json`, which provides methods to handle JSON data.
- Encoding to JSON (`dump` and `dumps` methods):
– `json.dumps()`: This method takes a Python object and returns its JSON representation as a string.
```python import json data = {"name": "Alice", "age": 30} json_string = json.dumps(data) ```
– `json.dump()`: This is used to write JSON data directly to a file-like object.
```python with open("data.json", "w") as file: json.dump(data, file) ```
- Decoding from JSON (`load` and `loads` methods):
– `json.loads()`: This method parses a JSON string and returns the corresponding Python object.
```python decoded_data = json.loads(json_string) ```
– `json.load()`: This is used to read JSON data directly from a file-like object.
```python with open("data.json", "r") as file: data_from_file = json.load(file) ```
- Complex Data Types:
By default, the `json` module can handle basic Python data types like dictionaries, lists, strings, numbers, and boolean values. For more complex types, like custom objects, you might need to supply custom functions to the `default` parameter of `dumps` or the `object_hook` parameter of `loads`.
- Benefits of JSON in Python:
– Cross-Language Compatibility: JSON is supported by nearly all modern programming languages, making it an excellent choice for data interchange between applications written in different languages.
– Human-Readable: The format is easy for humans to read and write, facilitating debugging and manual data inspection.
JSON is a powerful and widely-used format for data serialization in Python. By understanding the core methods provided by the `json` module, developers can easily convert between Python objects and JSON strings, enabling efficient storage, transmission, and integration with other systems.
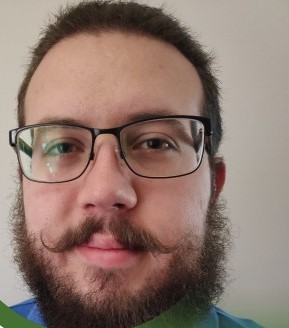
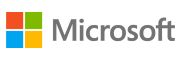