How to Use Python Functions for Stock Market Analysis
The stock market is a dynamic and intricate ecosystem where investors seek opportunities to maximize their returns. With the abundance of data available today, using Python for stock market analysis has become an indispensable tool for both beginners and seasoned traders. Python’s simplicity, versatility, and powerful libraries make it an ideal choice for analyzing stock market data and making informed investment decisions. In this blog post, we’ll delve into the world of Python functions and how they can be harnessed for effective stock market analysis.
Table of Contents
1. Understanding Stock Market Analysis
1.1. Why Python for Stock Market Analysis?
Python has gained tremendous popularity among analysts and traders due to its versatility and robust ecosystem of libraries. Libraries like Pandas, NumPy, Matplotlib, and more provide the tools necessary for data manipulation, analysis, and visualization. The simplicity of Python syntax also makes it accessible to those with varying levels of programming experience.
2. Getting Started with Python Functions
2.1. What Are Functions in Python?
Functions are blocks of code that perform a specific task and can be reused throughout your program. They allow you to modularize your code, making it more organized and easier to maintain. In the context of stock market analysis, functions can encapsulate various calculations and analyses, making your codebase more efficient.
2.2. Defining and Calling Functions
python def calculate_average(data): total = sum(data) num_elements = len(data) average = total / num_elements return average stock_prices = [50.2, 51.7, 49.5, 48.9, 50.6] avg_price = calculate_average(stock_prices) print("Average stock price:", avg_price)
2.3. Passing Parameters to Functions
Functions can accept parameters, allowing you to pass data or values to them for processing. This is particularly useful for applying the same analysis to different stocks or time periods.
python def calculate_rsi(prices): # RSI calculation logic here return rsi_value stock_data = [...] # Stock price data rsi = calculate_rsi(stock_data) print("RSI value:", rsi)
2.4. Returning Values from Functions
Functions can also return values, which can then be used for further analysis or decision-making.
python def analyze_bollinger_bands(data): # Bollinger Bands analysis logic here return signal price_data = [...] # Stock price data trade_signal = analyze_bollinger_bands(price_data) if trade_signal == "Buy": print("Consider buying the stock.")
3. Collecting and Preparing Stock Market Data
3.1. Using APIs to Fetch Stock Data
Many financial data providers offer APIs that allow you to fetch real-time or historical stock price data. Python’s requests library can be used to interact with these APIs and retrieve the data.
python import requests def fetch_stock_data(symbol, start_date, end_date): base_url = "https://api.example.com" params = {"symbol": symbol, "start_date": start_date, "end_date": end_date} response = requests.get(base_url, params=params) data = response.json() return data stock_symbol = "AAPL" start_date = "2023-01-01" end_date = "2023-07-31" stock_data = fetch_stock_data(stock_symbol, start_date, end_date)
3.2. Data Cleaning and Preprocessing
Before analysis, it’s essential to clean and preprocess the data. Pandas, a powerful library for data manipulation, comes to the rescue here.
python import pandas as pd df = pd.DataFrame(stock_data) df["Date"] = pd.to_datetime(df["Date"]) df.set_index("Date", inplace=True) # Perform data cleaning and preprocessing here
4. Performing Technical Analysis
4.1. Calculating Moving Averages
Moving averages are commonly used technical indicators. A simple moving average (SMA) can be calculated using the rolling function in Pandas.
python def calculate_sma(data, window): sma = data["Close"].rolling(window=window).mean() return sma window_size = 20 sma_20 = calculate_sma(df, window_size)
4.2. Relative Strength Index (RSI) Calculation
RSI is another important indicator. Here’s a simplified example of calculating RSI using Pandas.
python def calculate_rsi(data, window): # RSI calculation logic here return rsi_series rsi_window = 14 rsi_values = calculate_rsi(df, rsi_window)
4.3. Bollinger Bands Analysis
Bollinger Bands help identify potential overbought or oversold conditions.
python def analyze_bollinger_bands(data, window, num_std): # Bollinger Bands analysis logic here return signals bollinger_window = 20 num_std_dev = 2 bollinger_signals = analyze_bollinger_bands(df, bollinger_window, num_std_dev)
5. Sentiment Analysis with Natural Language Processing
5.1. Fetching Financial News Using APIs
To gauge market sentiment, you can retrieve financial news articles using APIs.
python def fetch_news_articles(query, num_articles): # News API integration code here return news_data search_query = "stock market" num_articles = 5 news_data = fetch_news_articles(search_query, num_articles)
5.2. Text Preprocessing for Sentiment Analysis
Clean and preprocess the text data before sentiment analysis.
python def preprocess_text(text): # Text preprocessing steps here return preprocessed_text preprocessed_news = [preprocess_text(article) for article in news_data]
5.3. Calculating Sentiment Scores
Use NLP libraries like NLTK or TextBlob to calculate sentiment scores.
python from textblob import TextBlob def calculate_sentiment(text): blob = TextBlob(text) sentiment_score = blob.sentiment.polarity return sentiment_score sentiment_scores = [calculate_sentiment(article) for article in preprocessed_news]
6. Visualizing Insights with Matplotlib
6.1. Creating Candlestick Charts
Candlestick charts are great for visualizing stock price movements.
python import mplfinance as mpf def plot_candlestick(data): # Candlestick chart plotting logic here mpf.plot(data, type="candle") plot_candlestick(df)
6.2. Plotting Technical Indicators
Visualize technical indicators alongside price data.
python import matplotlib.pyplot as plt def plot_technical_indicators(data, indicator1, indicator2): plt.figure(figsize=(10, 6)) plt.plot(data.index, data["Close"], label="Price") plt.plot(data.index, indicator1, label="SMA") plt.plot(data.index, indicator2, label="RSI") plt.legend() plt.xlabel("Date") plt.ylabel("Value") plt.title("Technical Indicators") plt.show() plot_technical_indicators(df, sma_20, rsi_values)
6.3. Displaying Sentiment Analysis Results
Visualize sentiment scores over time.
python def plot_sentiment_analysis(scores, dates): plt.figure(figsize=(10, 4)) plt.plot(dates, scores, marker="o") plt.xlabel("Date") plt.ylabel("Sentiment Score") plt.title("Sentiment Analysis") plt.xticks(rotation=45) plt.tight_layout() plt.show() plot_sentiment_analysis(sentiment_scores, df.index)
7. Building a Decision Support System
7.1. Combining Technical and Sentiment Analysis
Combine technical and sentiment analysis to make more informed decisions.
python def make_trading_decision(price_signal, sentiment_score): # Decision logic here if price_signal == "Buy" and sentiment_score > 0: return "Strong Buy" elif price_signal == "Sell" and sentiment_score < 0: return "Avoid Selling" else: return "Hold" decision = make_trading_decision(bollinger_signals, sentiment_scores[-1]) print("Trading decision:", decision)
7.2. Implementing Buy/Sell Signals
Incorporate the decision logic into your trading strategy.
python def generate_trade_signal(price_signal, sentiment_score): decision = make_trading_decision(price_signal, sentiment_score) if decision == "Strong Buy": return "Buy" elif decision == "Avoid Selling": return "Hold" else: return "No Trade" trade_signal = generate_trade_signal(bollinger_signals[-1], sentiment_scores[-1]) print("Trade signal:", trade_signal)
7.3. Backtesting Strategies
Backtest your trading strategy using historical data.
python def backtest_strategy(data, trade_signals): # Backtesting logic here return backtest_results backtest_results = backtest_strategy(df, bollinger_signals) print("Backtesting results:", backtest_results)
8. Risk Management and Portfolio Optimization
8.1. Calculating Risk-Adjusted Returns
Evaluate risk-adjusted returns using metrics like Sharpe ratio.
python def calculate_sharpe_ratio(data): # Sharpe ratio calculation logic here return sharpe_ratio sharpe_ratio = calculate_sharpe_ratio(df) print("Sharpe ratio:", sharpe_ratio)
8.2. Efficient Frontier and Portfolio Allocation
Use techniques like the efficient frontier to optimize portfolio allocation.
python def optimize_portfolio(data, num_portfolios): # Portfolio optimization logic here return optimized_portfolio num_portfolios = 1000 optimized_allocation = optimize_portfolio(df, num_portfolios) print("Optimized portfolio allocation:", optimized_allocation)
8.3. Automating Portfolio Rebalancing
Implement a rebalancing strategy to maintain your portfolio’s desired allocation.
python def rebalance_portfolio(data, current_allocation, target_allocation): # Portfolio rebalancing logic here return new_allocation new_allocation = rebalance_portfolio(df, current_allocation, target_allocation) print("New portfolio allocation:", new_allocation)
Conclusion
Using Python functions for stock market analysis can significantly enhance your ability to make informed investment decisions. From collecting and preprocessing data to performing technical analysis and sentiment analysis, Python’s rich ecosystem of libraries and functions provides the tools needed to navigate the complexities of the stock market. By visualizing insights, building decision support systems, and optimizing portfolio allocation, you can approach stock market analysis with confidence and increase your chances of success in the dynamic world of finance. Whether you’re a novice investor or an experienced trader, leveraging Python functions can empower you to stay ahead in the stock market game.
Table of Contents
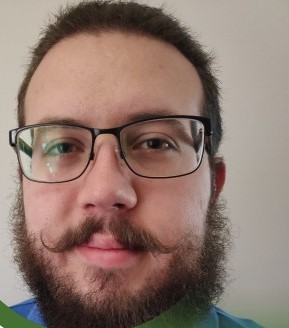
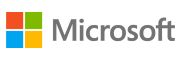