How to Use Python Functions for Time Series Analysis
Time series data, a sequence of observations collected over time, plays a crucial role in various domains like finance, economics, and natural sciences. Analyzing time series data can provide insights into trends, patterns, and seasonality, aiding in informed decision-making. Python, with its rich ecosystem of libraries, offers a powerful toolkit for time series analysis. In this blog post, we’ll delve into how to leverage Python functions for effective time series analysis, covering techniques, examples, and best practices.
Table of Contents
1. Why Python for Time Series Analysis?
Python has gained immense popularity in data science and analytics due to its user-friendly syntax and an extensive collection of libraries tailored for various tasks. Some key libraries for time series analysis include:
- pandas: A versatile library for data manipulation and analysis. It provides a specialized DataFrame object that’s well-suited for handling time series data.
- NumPy: The fundamental package for scientific computing with Python. It offers support for large, multi-dimensional arrays and matrices, making it ideal for numerical operations.
- matplotlib and seaborn: These libraries help in creating visualizations to visualize trends and patterns in time series data.
- statsmodels: This library offers tools for exploring data, estimating statistical models, and performing hypothesis tests on time series data.
- scikit-learn: While primarily known for machine learning, scikit-learn also includes utilities for time series preprocessing and feature extraction.
2. Getting Started with Time Series Data
Before diving into analysis, let’s first understand how to load and preprocess time series data using Python functions.
2.1. Importing Libraries
python import pandas as pd import numpy as np import matplotlib.pyplot as plt import seaborn as sns
2.2. Loading Data
python # Load time series data into a DataFrame data = pd.read_csv('time_series_data.csv') # Display the first few rows of the DataFrame print(data.head())
2.3. Preprocessing Data
python # Convert the 'timestamp' column to datetime format data['timestamp'] = pd.to_datetime(data['timestamp']) # Set the 'timestamp' column as the index data.set_index('timestamp', inplace=True) # Handle missing values data = data.fillna(method='ffill') # Forward fill missing values
3. Exploratory Data Analysis (EDA) with Python
Exploring the data visually is essential to identify trends, seasonality, and potential anomalies.
3.1. Line Plot
python # Line plot of the time series data plt.figure(figsize=(10, 6)) plt.plot(data.index, data['value'], label='Value') plt.title('Time Series Data') plt.xlabel('Timestamp') plt.ylabel('Value') plt.legend() plt.show()
3.2. Seasonal Decomposition
python from statsmodels.tsa.seasonal import seasonal_decompose # Seasonal decomposition of the time series decomposition = seasonal_decompose(data['value'], model='additive') decomposition.plot() plt.show()
4. Time Series Analysis Techniques
Now that we have a grasp of the data, let’s explore some fundamental time series analysis techniques using Python functions.
4.1. Moving Average
The moving average smooths out fluctuations in data, making it easier to identify underlying trends.
python # Calculate and plot the 7-day moving average data['7-day MA'] = data['value'].rolling(window=7).mean() plt.figure(figsize=(10, 6)) plt.plot(data.index, data['value'], label='Value') plt.plot(data.index, data['7-day MA'], label='7-day Moving Avg') plt.title('Time Series with Moving Average') plt.xlabel('Timestamp') plt.ylabel('Value') plt.legend() plt.show()
4.2. Seasonal Adjustment
python # Seasonal adjustment using the seasonal component from decomposition data['seasonal_adj'] = data['value'] - decomposition.seasonal plt.figure(figsize=(10, 6)) plt.plot(data.index, data['seasonal_adj'], label='Seasonal Adjusted') plt.title('Seasonal Adjusted Time Series') plt.xlabel('Timestamp') plt.ylabel('Value') plt.legend() plt.show()
4.3. Autocorrelation and Partial Autocorrelation
python from statsmodels.graphics.tsaplots import plot_acf, plot_pacf # Autocorrelation plot plot_acf(data['value']) plt.title('Autocorrelation Plot') # Partial autocorrelation plot plot_pacf(data['value']) plt.title('Partial Autocorrelation Plot')
5. Predictive Modeling for Time Series
Python functions also facilitate predictive modeling for time series data. Let’s explore a simple example using an autoregressive integrated moving average (ARIMA) model.
5.1. ARIMA Modeling
python from statsmodels.tsa.arima_model import ARIMA # Fit an ARIMA model model = ARIMA(data['value'], order=(2,1,2)) model_fit = model.fit(disp=0) print(model_fit.summary())
5.2. Model Evaluation
python # Plot the actual vs. predicted values plt.figure(figsize=(10, 6)) plt.plot(data.index, data['value'], label='Actual') plt.plot(data.index, model_fit.fittedvalues, color='red', label='Predicted') plt.title('ARIMA Model: Actual vs. Predicted') plt.xlabel('Timestamp') plt.ylabel('Value') plt.legend() plt.show()
Conclusion
Python offers a comprehensive set of functions and libraries that empower data analysts and scientists to conduct robust time series analysis. This blog post covered just a glimpse of the capabilities Python provides for exploring, visualizing, and modeling time series data. By mastering these techniques and consistently practicing them, you can unlock valuable insights from your time series data and make informed decisions across various domains. Whether you’re studying financial markets, weather patterns, or any other time-dependent phenomena, Python’s functions have you covered. So, dive in, experiment, and elevate your time series analysis game with Python!
Table of Contents
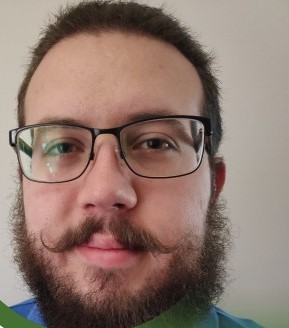
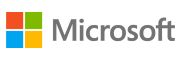