How to handle Unicode and encoding issues in Python?
Handling Unicode and encoding issues is paramount for developers, especially in today’s globalized world where applications often need to support a myriad of languages and scripts. Here’s a concise guide to addressing these challenges in Python:
- Understanding Unicode:
Unicode is a universal character encoding standard that aims to represent all the characters from all the world’s languages. Each character in Unicode has a unique code point, allowing consistent representation across different platforms and devices.
- String Types in Python:
– Python 2: There are two primary string types: `str` (a sequence of bytes) and `unicode` (a sequence of Unicode code points). This distinction has often led to confusion and encoding-related bugs.
– Python 3: Python made a significant leap in simplifying string handling. There’s `str` (a sequence of Unicode code points) and `bytes` (a sequence of bytes). This makes it explicit when you’re dealing with text (in Unicode) versus binary data.
- Encoding and Decoding:
– Encoding: Converting a `str` (Unicode) to `bytes`. For this, you use the `.encode()` method on a string, specifying the desired encoding (like ‘utf-8’).
– Decoding: Converting `bytes` back to a `str`. You use the `.decode()` method on a bytes object, providing the encoding it should be interpreted in.
- Common Pitfalls:
– Assuming Encoding: Never assume a particular encoding, especially when dealing with external data sources. Always verify the encoding used.
– Using Non-Unicode Aware Libraries: Some older Python libraries aren’t Unicode-aware, which can lead to issues when they encounter non-ASCII characters.
- Best Practices:
– Default to UTF-8: UTF-8 is the most widely used Unicode encoding. It’s compatible with ASCII, making it a good choice for many applications.
– Be Explicit: When opening files, always specify the encoding using the `encoding` parameter, e.g., `open(‘file.txt’, ‘r’, encoding=’utf-8′)`.
– Use Unicode Literals: In Python 2, prefix strings with `u` to make them Unicode literals (e.g., `u’hello’`). In Python 3, all string literals are Unicode by default.
– Handle Exceptions: Be prepared for `UnicodeEncodeError` and `UnicodeDecodeError`. These exceptions occur when converting between `str` and `bytes` fails due to character mismatches.
Understanding and handling Unicode in Python is vital for creating globally relevant applications. By embracing best practices, developers can ensure consistent and error-free text processing across diverse languages and scripts.
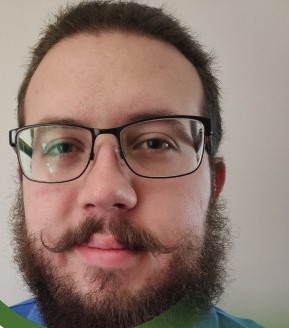
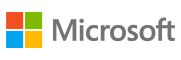