What is unit testing and how to do it in Python?
Unit testing is a software testing technique where individual units or components of a software are tested in isolation from the rest of the application. The main objective is to validate that each unit of the software performs as designed. In Python, the primary module for unit testing is the built-in `unittest` library.
Using the `unittest` Framework:
The `unittest` framework in Python is inspired by Java’s JUnit. It supports test automation, aggregation of tests into collections, and independence of tests from the reporting framework.
- Basic Test Structure:
In `unittest`, tests are structured as methods in a test class that inherits from `unittest.TestCase`. Within these methods, you utilize assert methods to validate outcomes:
```python import unittest def add(x, y): return x + y class TestAddFunction(unittest.TestCase): def test_add_positive_numbers(self): self.assertEqual(add(2, 3), 5) def test_add_negative_numbers(self): self.assertEqual(add(-1, -1), -2) ```
- Running the Tests:
To execute the tests, you can use the following if-statement at the bottom of your test file:
```python if __name__ == '__main__': unittest.main() ```
Or, you can run the tests from the command line using:
``` python -m unittest test_filename.py ```
- More Assertions:
The `unittest` framework provides a plethora of assertion methods such as `assertEqual`, `assertTrue`, `assertFalse`, `assertRaises`, and many others, aiding in a wide range of test scenarios.
Unit testing is essential for ensuring the correct functioning of individual components of a software application. Python’s `unittest` framework provides a robust and comprehensive environment for crafting and executing unit tests, thereby helping developers build reliable and maintainable software. As with all testing, the goal is not only to catch errors but to create a safety net for future changes, ensuring that modifications don’t introduce regressions.
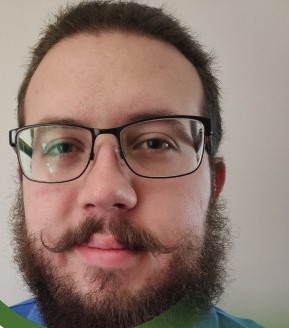
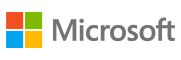