Keeping Tabs on Your Elements: The useInView Hook in ReactJS for Seamless Viewport Detection
Welcome to this guide on using `useInView`, a powerful hook in ReactJS that detects if an element is within the viewport. This tool is built on the Intersection Observer API and enhances web development efficiency by controlling element load and activation. `useInView` helps create dynamic experiences like lazy loading images or triggering animations. Regardless of your ReactJS experience, this guide will provide valuable insights into using `useInView` for viewport detection to optimize your applications.
The Basics of useInView in ReactJSThe Basics of useInView in ReactJS
`useInView` is a custom hook in ReactJS that utilizes the Intersection Observer API to observe changes in the intersection of a target element with an ancestor element or the viewport. It provides a simplified, React-compatible interface to identify when a component enters or leaves the viewport. When the `useInView` hook is used, it returns a ref to be assigned to the observed element and a Boolean `inView` value, indicating whether the element is in the viewport.
Here’s a simple usage example:
import { useInView } from 'react-intersection-observer'; function MyComponent() { const [ref, inView] = useInView({ triggerOnce: true, // Change this to false if you want to observe continuously }); return <div ref={ref}>{inView ? 'In viewport' : 'Not in viewport'}</div>; }
In this example, when the `div` element comes into the viewport, `inView` becomes `true`, and ‘In viewport’ is rendered. If the `div` is not in the viewport, `inView` is `false`, and ‘Not in viewport’ is rendered. The `triggerOnce` option ensures the observer is disconnected after the element has been in view once.
Remember, `useInView` does more than just reporting visibility. It can drive significant UI interactions, such as loading content when it’s needed or initiating animations. We’ll explore more of these advanced concepts later in the post.
Prerequisites for Using useInView
The blog post emphasizes that before using the `useInView` hook in ReactJS, certain prerequisites need to be met:
- JavaScript Knowledge: A strong understanding of JavaScript, especially ES6 features such as destructuring, arrow functions, and hooks, is essential.
- Understanding of ReactJS: As `useInView` is a React hook, familiarity with ReactJS concepts like creating components, understanding component lifecycle methods, and hooks is necessary.
- A working ReactJS Environment: A development environment with Node.js and npm installed is required. A React project setup, possibly created with `create-react-app`, is also necessary.
- Understanding of HTML & CSS: Basic HTML and CSS knowledge is required as the elements being observed with `useInView` are defined in these languages.
- Familiarity with npm: As `useInView` is distributed as an npm package, comfort with using npm to install packages is required.
- Understanding of Intersection Observer API: Although not essential, a basic understanding of the Intersection Observer API, which `useInView` uses to detect when elements are in the viewport, could be beneficial.
With these prerequisites met, one is prepared to effectively use `useInView` in their ReactJS projects.
Installing and Setting up useInView
Setting up `useInView` in your React project is straightforward. Follow the steps below to install and set it up:
1. Installation:
The first thing you need to do is to install the `react-intersection-observer` package. It’s the package that contains the `useInView` hook. You can install it using npm or yarn.
Using npm:
npm install react-intersection-observer
Or using yarn:
yarn add react-intersection-observer
2. Importing `useInView`:
After installation, you can import the `useInView` hook in your React component file where you want to use it. Here’s how you do it:
import { useInView } from 'react-intersection-observer';
3. Using `useInView` in a Component:
Now you can use `useInView` within your component. Remember, `useInView` returns an array with a ref and a Boolean value. You can assign this ref to the element you want to observe. The Boolean value indicates whether the observed element is in the viewport (`true`) or not (`false`).
Here’s an example of a simple React component that uses `useInView`:
import React from 'react'; import { useInView } from 'react-intersection-observer'; const MyComponent = () => { const [ref, inView] = useInView({ triggerOnce: true, }); return <div ref={ref}>{inView ? 'In viewport' : 'Not in viewport'}</div>; }; export default MyComponent;
That’s it! You’ve successfully installed and set up `useInView` in your React application. The next sections will go into detail on how to effectively utilize `useInView` with practical examples and advanced concepts.
Examples of useInView Usage
Let’s explore some practical examples to illustrate how `useInView` can be used in a real-world React application.
Example 1: Basic Usage of `useInView`
This is the simplest use case of `useInView` where you want to track when an element is visible in the viewport.
import React from 'react'; import { useInView } from 'react-intersection-observer'; const MyComponent = () => { const [ref, inView] = useInView({ triggerOnce: true, }); return <div ref={ref}>{inView ? 'In viewport' : 'Not in viewport'}</div>; }; export default MyComponent;
In this example, when the `div` enters the viewport, `inView` becomes `true`, and the message ‘In viewport’ is displayed. When it’s not in the viewport, ‘Not in viewport’ is displayed. The `triggerOnce` option ensures the observer disconnects after the element has been in view once.
Example 2: Lazy Loading Images
`useInView` is excellent for lazy loading images or content. Here’s an example of how to do it:
import React from 'react'; import { useInView } from 'react-intersection-observer'; const LazyImage = ({ src, alt }) => { const [ref, inView] = useInView({ triggerOnce: true, // The image loads once and won't unload }); return <img ref={ref} src={inView ? src : ''} alt={alt} />; }; export default LazyImage;
In this example, the image’s source (`src`) is only set when the image comes into the viewport. This approach can enhance performance, especially for pages with many large images.
These examples illustrate the versatility of `useInView`. By combining it with other features or hooks, you can create some powerful interactions and optimizations in your application. As always, ensure you understand what the code is doing and tweak it to suit your specific use case.
Advanced Concepts
Now that we have covered the basics and practical usage examples of `useInView`, let’s delve into some advanced concepts that will further enhance your understanding and usage of this hook.
1. Understanding `rootMargin` and `threshold` options:
`useInView` can be customized with an options object, which includes `rootMargin` and `threshold`:
– `rootMargin`: Similar to CSS margin, this adjusts the root element’s bounding box (usually the viewport), influencing when an element is reported as visible. For instance, a `rootMargin` of ‘100px’ will report an element as visible when it’s within 100px of the viewport.
– `threshold`: This can be a number or an array of numbers between 0.0 and 1.0, representing the percentage of the element’s visibility. The callback triggers each time the intersection crosses one of these thresholds. A threshold of 0.5, for instance, means the callback will run when 50% of the element is visible.
const [ref, inView] = useInView({ rootMargin: '-100px 0px', threshold: 0.5, });
2. Observing Multiple Elements:
You can use `useInView` to observe multiple elements. You’ll need to create separate refs for each element you want to observe.
import React from 'react'; import { useInView } from 'react-intersection-observer'; const MyComponent = () => { const [ref1, inView1] = useInView({ triggerOnce: true }); const [ref2, inView2] = useInView({ triggerOnce: true }); return ( <div> <div ref={ref1}>{inView1 ? 'First div in viewport' : 'First div not in viewport'}</div> <div ref={ref2}>{inView2 ? 'Second div in viewport' : 'Second div not in viewport'}</div> </div> ); }; export default MyComponent;
3. Using `useInView` with Animation Libraries:
You can combine `useInView` with animation libraries like `framer-motion` to trigger animations when an element comes into view.
import React from 'react'; import { useInView } from 'react-intersection-observer'; import { motion } from 'framer-motion'; const MyComponent = () => { const [ref, inView] = useInView({ triggerOnce: true, }); return ( <motion.div ref={ref} animate={{ opacity: inView ? 1 : 0 }} > Content </motion.div> ); }; export default MyComponent;
In this example, the `div`’s opacity animates to 1 when the `div` comes into the viewport, creating a fade-in effect.
These are a few examples of how you can leverage `useInView`’s versatility. Understanding these advanced concepts will help you to create more dynamic and interactive experiences in your applications.
Best Practices and Potential Pitfalls
When integrating `useInView` into your React applications, consider these best practices:
- Leverage Lazy Loading: Use `useInView` for lazy loading to improve application performance by loading content only when needed.
- Limit Use for Small Components: Optimize performance by observing larger, singular components instead of multiple smaller ones.
- Combine with Animation Libraries: Enhance user experience by initiating animations when a component comes into view, using animation libraries like `framer-motion` or `react-spring`.
However, be aware of potential pitfalls:
- Not Understanding Intersection Observer API: As `useInView` is a wrapper around the Intersection Observer API, it’s beneficial to understand it to effectively utilize `useInView`.
- Ignoring Browser Compatibility: The Intersection Observer API, and hence `useInView`, doesn’t have universal browser support. If your application needs to support a broad range of browsers, consider using a polyfill.
- Misusing the `triggerOnce` Option: Misusing this option could disconnect the observer after the first trigger, not ideal if continuous observation is required.
Adherence to these practices and awareness of potential pitfalls can enhance your effective use of `useInView` in your React applications.
Conclusion
This guide has provided a comprehensive exploration of the `useInView` hook in ReactJS, a powerful tool for identifying when an element is in the viewport. The guide covers basic usage, setup procedures, practical examples, and dives into advanced concepts. It also points out best practices and potential pitfalls.
The appropriate application of `useInView` can notably enhance a React application, offering dynamic, interactive user experiences such as triggering animations or implementing lazy loading for improved performance.
It’s crucial to remember that each application is unique, and the effective use of `useInView` depends on the project’s specific needs. Continue experimenting and learning to fully harness the potential of `useInView` in ReactJS.
Happy coding!
Table of Contents
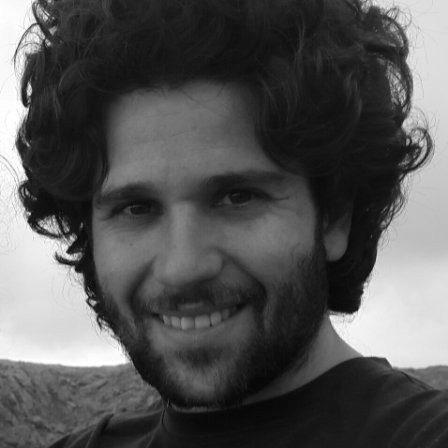
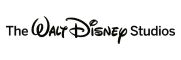