WordPress Plugin Security: Best Practices for Programmers
WordPress plugins are essential for adding functionality and features to your website. With over 50,000 plugins available in the WordPress repository, there’s no shortage of options to enhance your site. However, while plugins can greatly enhance your website’s capabilities, they can also introduce security vulnerabilities if not developed and maintained properly.
Table of Contents
In this guide, we will explore best practices for ensuring the security of your WordPress plugins. Whether you are a seasoned developer or just getting started with plugin development, these tips and techniques will help you create secure and reliable plugins that won’t compromise the security of your website or your users’ data.
1. Understanding the Importance of Plugin Security
Before we dive into the best practices, it’s crucial to understand why plugin security is paramount. WordPress powers over 40% of websites on the internet, making it an attractive target for hackers. They are constantly looking for vulnerabilities in plugins to gain unauthorized access, inject malicious code, or steal sensitive information.
When a security vulnerability is discovered in a plugin, it can lead to:
- Compromised Websites: Hackers can exploit vulnerabilities to take control of websites, deface them, or distribute malware.
- Data Breaches: Sensitive user data, such as personal information and login credentials, can be stolen and misused.
- Reputation Damage: A compromised website can lead to a loss of trust among visitors, customers, and search engines.
- Legal Consequences: Depending on your region and the data you handle, data breaches may have legal ramifications.
Now that you understand the significance of plugin security, let’s explore the best practices to ensure your plugins are safe and trustworthy.
2. Keep Your Plugins Updated
2.1. Regular Updates Matter
One of the most critical aspects of plugin security is keeping your plugins up to date. Developers often release updates to address security vulnerabilities and improve overall functionality. Failing to update your plugins can leave your website exposed to known security risks.
Here’s how you can ensure your plugins are always up to date:
php // Code to check for plugin updates add_action('admin_init', 'check_plugin_updates'); function check_plugin_updates() { $plugins = get_plugins(); foreach ($plugins as $plugin) { $plugin_data = get_plugin_data(WP_PLUGIN_DIR . '/' . $plugin); $current_version = $plugin_data['Version']; $latest_version = get_latest_plugin_version($plugin_data['Name']); if (version_compare($current_version, $latest_version, '<')) { // Notify the admin that an update is available add_action('admin_notices', 'show_update_notice'); break; } } } function show_update_notice() { echo '<div class="notice notice-warning"><p>A new plugin update is available. Please update now.</p></div>'; } function get_latest_plugin_version($plugin_name) { // Use an API or custom logic to fetch the latest version // Example: Fetch the latest version from the WordPress.org repository $api_url = 'https://api.wordpress.org/plugins/info/1.0/' . $plugin_name . '.json'; $response = wp_remote_get($api_url); if (is_wp_error($response)) { return false; } $data = json_decode(wp_remote_retrieve_body($response)); return $data->version; }
The code above checks for updates of all installed plugins and notifies the admin if updates are available. It also provides a function to fetch the latest version of a plugin from the WordPress.org repository.
2.2. Use a Version Control System
To maintain code integrity and track changes, it’s essential to use a version control system (VCS) like Git. This allows you to manage your plugin’s codebase efficiently, collaborate with other developers, and roll back changes if necessary.
Here’s a simple Git workflow for managing your WordPress plugin:
shell # Initialize a Git repository in your plugin directory git init # Add your plugin files to the repository git add . # Commit your changes git commit -m "Initial commit" # Connect your local repository to a remote repository (e.g., GitHub) git remote add origin https://github.com/your-username/your-plugin.git # Push your code to the remote repository git push -u origin master
Using a VCS also helps you track security-related updates and apply them promptly.
3. Follow WordPress Coding Standards
3.1. Coding Standards Ensure Consistency
Adhering to WordPress coding standards is crucial for maintaining plugin security and compatibility with the WordPress core. These standards include guidelines on naming conventions, code structure, and security practices.
Here are some essential coding standards to follow:
3.2. Naming Conventions
Function Names: Use clear and descriptive function names to improve code readability. Avoid using generic names like “function1” or “myFunction.”
php // Good function calculate_total_price() { // Function logic here } // Bad function function1() { // Unclear function purpose } Variable Names: Use meaningful variable names that indicate their purpose. php Copy code // Good $user_id = get_current_user_id(); // Bad $x = get_current_user_id(); // Unclear variable name
3.3. Sanitize and Validate Data
Always sanitize and validate user input to prevent security vulnerabilities like SQL injection and cross-site scripting (XSS) attacks. WordPress provides functions like sanitize_text_field() and is_email() for this purpose.
php // Sanitize and validate user input $user_input = $_POST['user_input']; $clean_input = sanitize_text_field($user_input); if (is_email($clean_input)) { // Valid email address } else { // Invalid input }
3.4. Escaping Output
When displaying user-generated content on your website, use appropriate escaping functions to prevent XSS attacks.
php // Escaping output $user_generated_content = '<script>alert("XSS attack");</script>'; echo esc_html($user_generated_content); // This will display the content as text, not execute the script
3.5. Security Functions
WordPress provides security functions like wp_nonce_field() and check_admin_referer() to help protect against cross-site request forgery (CSRF) attacks.
php // Add a nonce field to a form wp_nonce_field('my_action', 'my_nonce'); // Verify the nonce when processing the form submission if (isset($_POST['my_nonce']) && wp_verify_nonce($_POST['my_nonce'], 'my_action')) { // Nonce is valid, process the form data } else { // Nonce is invalid, reject the request }
By following these coding standards, you reduce the risk of introducing security vulnerabilities into your plugin’s codebase.
4. Implement Proper User Access Controls
4.1. Limit User Access
User access control is crucial for ensuring that only authorized users can perform specific actions within your plugin. WordPress provides a robust role and capability system that allows you to define what each user role can and cannot do.
Here are some guidelines for implementing proper user access controls:
4.2. Use WordPress Roles and Capabilities
- Administrator: This role should be limited to trusted individuals who can manage the entire site and plugins.
- Editor: Editors can edit and publish posts but should not have access to plugin settings.
- Author: Authors can create and publish their posts, but they shouldn’t have access to sensitive plugin functionality.
- Subscriber: Subscribers have the least privileges and should not have access to any plugin settings.
php // Check if the current user is an administrator if (current_user_can('administrator')) { // Allow access to sensitive functionality } else { // Restrict access }
4.2. Implement Custom User Roles
In some cases, you may need to create custom user roles with specific capabilities tailored to your plugin’s functionality. You can achieve this using plugins like “Members” or by writing custom code.
php // Define a custom user role function add_custom_user_role() { add_role('custom_role', 'Custom Role', [ 'read' => true, 'edit_posts' => true, 'upload_files' => true, ]); } add_action('init', 'add_custom_user_role');
4.3. Protect Sensitive Operations
If your plugin includes actions that can have significant consequences, such as deleting data or changing settings, always verify the user’s capabilities before allowing them to perform those actions.
php // Check if the user has permission to delete data if (current_user_can('delete_data')) { // Perform the delete operation } else { // Display an error message or deny access }
By implementing proper user access controls, you can prevent unauthorized users from making critical changes to your plugin or website.
5. Sanitize and Validate User Input
5.1. Input Validation Prevents Vulnerabilities
Sanitizing and validating user input is a fundamental security practice that can protect your plugin from various attacks, including SQL injection and XSS attacks. WordPress provides built-in functions for these tasks.
5.2. Sanitize User Input
When receiving user input, especially from forms, always sanitize it to remove any potentially harmful content. WordPress offers functions like sanitize_text_field(), sanitize_email(), and sanitize_url() for this purpose.
php // Sanitize user input $user_input = $_POST['user_input']; $clean_input = sanitize_text_field($user_input);
5.3. Validate User Input
In addition to sanitization, validate user input to ensure it matches the expected format or criteria. For example, you can use is_email() to validate email addresses and is_numeric() to ensure numeric input.
php // Validate email input $user_email = $_POST['user_email']; if (is_email($user_email)) { // Valid email address } else { // Invalid email address }
5.4. Use Prepared Statements for Database Queries
When interacting with the database, use prepared statements to prevent SQL injection attacks. WordPress provides the $wpdb class, which includes methods for secure database queries.
php global $wpdb; // Using a prepared statement $sql = $wpdb->prepare("SELECT * FROM {$wpdb->prefix}my_table WHERE id = %d", $user_id); $results = $wpdb->get_results($sql);
By sanitizing and validating user input and using prepared statements, you can significantly reduce the risk of common security vulnerabilities in your plugin.
6. Secure File Uploads
6.1. Safely Handle File Uploads
If your plugin allows users to upload files, such as images or documents, it’s essential to handle file uploads securely. Failing to do so can lead to security vulnerabilities, including arbitrary code execution.
Here are some best practices for secure file uploads:
6.2. Limit Allowed File Types
Specify which file types users can upload and block potentially dangerous file types. You can use the wp_check_filetype() function to verify file extensions and MIME types.
php // Check if the file type is allowed $allowed_types = ['jpg', 'jpeg', 'png', 'gif']; $file_info = wp_check_filetype($_FILES['user_file']['name']); if (in_array($file_info['ext'], $allowed_types)) { // File type is allowed, proceed with the upload } else { // File type not allowed, reject the upload }
6.3. Use a Secure Upload Directory
Store uploaded files in a secure directory outside the web root to prevent direct access. You can use the wp_upload_dir() function to get the path to the upload directory.
php $upload_dir = wp_upload_dir(); $upload_path = $upload_dir['basedir'] . '/my_plugin_uploads/'; // Ensure the directory exists wp_mkdir_p($upload_path);
6.4. Rename Uploaded Files
To prevent overwriting existing files and to add an extra layer of security, consider renaming uploaded files. You can generate unique file names using functions like wp_unique_filename().
php // Generate a unique file name $unique_filename = wp_unique_filename($upload_path, $file_info['name']); $target_path = $upload_path . $unique_filename; // Move the uploaded file to the secure directory move_uploaded_file($_FILES['user_file']['tmp_name'], $target_path);
6.5. Validate Uploaded Files
Before using uploaded files, validate them to ensure they are not malicious. You can use libraries like PHP’s getimagesize() to check the file’s content type.
php // Validate an uploaded image file $valid_types = ['image/jpeg', 'image/png', 'image/gif']; $image_info = getimagesize($target_path); if (in_array($image_info['mime'], $valid_types)) { // Valid image file } else { // Invalid file type, reject the upload }
By following these best practices, you can secure file uploads in your plugin and prevent potential security risks.
7. Regularly Perform Security Audits
7.1. Continuous Monitoring for Vulnerabilities
Security is an ongoing process, and it’s essential to regularly audit your plugin’s codebase for vulnerabilities. Here are some steps to perform security audits:
7.2. Code Review
Regularly review your plugin’s code to identify security issues, such as missing sanitization, validation, or access controls. Encourage peer reviews to gain different perspectives and identify potential vulnerabilities.
7.3. Vulnerability Scanning
Use automated tools and services to scan your plugin for known vulnerabilities. Plugins like “Wordfence Security” and online tools like “VirusTotal” can help identify potential threats.
7.4. Security Headers
Implement security headers in your plugin, such as Content Security Policy (CSP), to mitigate XSS and other security risks. WordPress plugins like “HTTP Security Headers” can assist in setting up these headers.
7.5. Penetration Testing
Consider conducting penetration testing on your plugin to simulate real-world attack scenarios. This can help you discover vulnerabilities that automated tools may miss.
7.6. Keep Abreast of Security Updates
Stay informed about security updates for WordPress and any third-party libraries or frameworks your plugin relies on. Promptly apply security patches to address newly discovered vulnerabilities.
8. Utilize Security Plugins
8.1. Leverage Third-party Security Plugins
In addition to following best practices during development, consider using reputable security plugins to enhance your website’s security. These plugins provide an extra layer of protection and can help detect and prevent security threats.
Here are some popular security plugins for WordPress:
8.2. Wordfence Security
Wordfence Security is a comprehensive security plugin that offers firewall protection, malware scanning, and login attempt monitoring. It also provides real-time threat defense feeds to keep your site secure.
8.3. Sucuri Security
Sucuri Security offers website security monitoring, malware scanning, and a web application firewall (WAF). It can help you identify and mitigate security threats quickly.
8.4. iThemes Security
iThemes Security (formerly known as Better WP Security) provides various security features, including brute force protection, two-factor authentication, and file change detection.
8.5. All In One WP Security & Firewall
This plugin offers a range of security features, including user account monitoring, login lockdown, and a firewall to protect your website from malicious requests.
Security plugins are not a replacement for good development practices, but they can complement your security efforts and provide additional layers of protection.
Conclusion
Ensuring the security of your WordPress plugins is crucial to protect your website and your users. By following best practices such as keeping your plugins updated, adhering to coding standards, implementing proper user access controls, sanitizing and validating user input, securing file uploads, performing security audits, and utilizing security plugins, you can develop and maintain secure and reliable WordPress plugins.
Remember that security is an ongoing process, and staying vigilant is key to safeguarding your website from evolving threats. By prioritizing plugin security, you can build trust with your users and maintain the integrity of your WordPress site.
Table of Contents
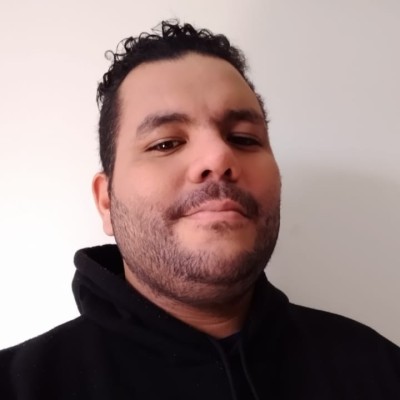
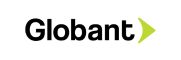