WordPress REST API: Authentication and Authorization with Programming
The WordPress REST API has revolutionized the way developers interact with WordPress sites, enabling them to create dynamic applications and services that communicate seamlessly with WordPress installations. However, with this increased connectivity comes the pressing need for ensuring data security. This blog post will delve into the essential concepts of authentication and authorization within the context of the WordPress REST API, and provide hands-on programming examples to demonstrate how to implement them effectively.
Table of Contents
1. Understanding Authentication and Authorization
1.1. Authentication: Who Are You?
Authentication is the process of verifying the identity of a user, application, or system attempting to access a resource. It ensures that only authorized individuals or entities can interact with your WordPress site via the REST API. In simpler terms, authentication answers the question, “Who are you?”
1.2. Token-Based Authentication
Token-based authentication is a prevalent method in modern web applications, including the WordPress REST API. The idea revolves around issuing a unique token (often a JSON Web Token or JWT) to authenticated users. This token is then included in the headers of subsequent API requests, allowing the server to identify the user without needing to store sensitive credentials.
Let’s take a look at a basic code example of token-based authentication using JavaScript:
javascript // Fetching data from the REST API with authentication const apiUrl = 'https://your-wordpress-site.com/wp-json/wp/v2/posts'; const authToken = 'your_jwt_token_here'; fetch(apiUrl, { method: 'GET', headers: { Authorization: `Bearer ${authToken}` } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
2. Authorization: What Are You Allowed to Do?
Authorization comes into play after authentication. Once a user’s identity is confirmed, authorization determines what actions they are allowed to perform. This could involve reading, creating, updating, or deleting specific resources. In a nutshell, authorization addresses the question, “What are you allowed to do?”
2.1. Role-Based Authorization
Role-based authorization is a widely used approach in WordPress, where each user is assigned a specific role (e.g., subscriber, author, editor, administrator). Each role carries a set of capabilities that dictate what actions the user can perform. The WordPress REST API leverages these roles to grant or deny access to different API endpoints.
Let’s see how to use role-based authorization in WordPress REST API:
php // Allowing access to a specific endpoint based on user role function customize_api_authorization($response, $handler, $request) { if (!is_user_logged_in()) { return new WP_Error('rest_forbidden', __('You are not authorized to access this endpoint.'), array('status' => 401)); } $user = wp_get_current_user(); if (!in_array('editor', $user->roles)) { return new WP_Error('rest_forbidden', __('You do not have permission to perform this action.'), array('status' => 403)); } return $response; } add_filter('rest_pre_dispatch', 'customize_api_authorization', 10, 3);
3. Implementing Authentication and Authorization in WordPress REST API
3.1. Securing the WordPress REST API with OAuth 2.0
OAuth 2.0 is a widely adopted framework for enabling secure authorization to APIs. By implementing OAuth 2.0 in your WordPress site, you can grant third-party applications limited access to your resources without exposing user credentials. This is particularly useful when building mobile apps or integrating external services.
To get started with OAuth 2.0 in WordPress, you can use plugins like “OAuth Server” or “WP OAuth Server,” which streamline the implementation process. Once set up, you can generate client IDs and secrets for authorized applications.
3.2. Adding Custom Endpoints with Advanced Authorization
Sometimes, the default roles and capabilities might not cover your specific use case. In such scenarios, you might need to create custom endpoints with advanced authorization logic. This allows you to finely control who can access certain resources and actions.
Let’s say you want to create a custom endpoint that allows authors to mark their posts as “featured.” Here’s how you can do it:
php // Creating a custom endpoint with advanced authorization function register_featured_endpoint() { register_rest_route('custom/v1', '/mark-featured/(?P<id>\d+)', array( 'methods' => 'POST', 'callback' => 'mark_post_featured', 'permission_callback' => 'custom_authorization_check' )); } function mark_post_featured($request) { // Logic to mark the post as featured } function custom_authorization_check() { if (!is_user_logged_in()) { return false; } $user = wp_get_current_user(); if (in_array('author', $user->roles)) { return true; } return false; } add_action('rest_api_init', 'register_featured_endpoint');
Conclusion
Securing your WordPress REST API is paramount to protecting your data, users, and resources. Understanding authentication and authorization is the first step towards building a robust and secure API. Token-based authentication and role-based authorization are powerful techniques to ensure that only authenticated users with appropriate privileges can interact with your WordPress site via the API.
By implementing OAuth 2.0, you can extend your API’s capabilities while maintaining a high level of security, making it an excellent choice for scenarios where third-party applications need controlled access.
Furthermore, creating custom endpoints with advanced authorization logic enables you to tailor access control to your specific requirements, going beyond the default roles and capabilities.
As the WordPress ecosystem continues to evolve, mastering these authentication and authorization techniques will empower you to build dynamic and secure applications that leverage the full potential of the WordPress REST API. So, go ahead and start implementing these practices to enhance the security of your WordPress-powered applications.
Remember, a secure API is the foundation of a trustworthy and resilient digital experience for your users, and staying informed about the latest security practices is an ongoing commitment that pays off in the long run.
Table of Contents
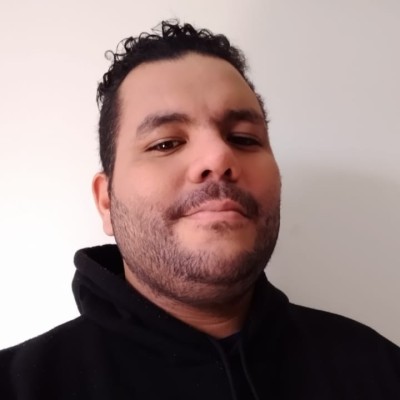
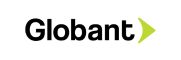