WordPress Theme Unit Testing: Ensuring Quality with Programming
In the world of WordPress theme development, ensuring quality and preventing bugs are paramount to delivering a seamless user experience. This is where unit testing comes into play. Unit testing involves breaking down your code into small, testable components and evaluating each component’s functionality independently. In this blog post, we’ll delve into the significance of unit testing in WordPress theme development, its benefits, and how to effectively implement it. Let’s explore how you can enhance your programming process and deliver top-notch themes to your users.
Table of Contents
1. The Importance of Unit Testing in WordPress Theme Development
1.1. Ensuring Functionality
WordPress themes are a complex amalgamation of templates, styles, scripts, and functionality. A small error in one part of the theme can lead to unexpected behavior or even site crashes. Unit testing allows you to identify issues early in the development process by testing individual components in isolation. By doing so, you can ensure that each piece of your theme works as intended before integrating it into the larger framework.
1.2. Preventing Regressions
As you make changes and enhancements to your theme over time, it’s not uncommon for new features to inadvertently break existing functionality. Unit tests act as safety nets, alerting you when changes lead to regressions—unintended negative impacts on previously functioning code. By running your unit tests after each change, you can quickly catch regressions and address them before they reach your users.
1.3. Enhancing Collaboration
In a collaborative development environment, where multiple developers might be working on the same theme, unit tests play a crucial role. They serve as a common benchmark for evaluating code quality and functionality. With well-written unit tests, developers can confidently make changes, knowing that their modifications won’t disrupt existing features. This collaborative harmony becomes even more vital as your theme grows and evolves.
2. The Benefits of Unit Testing
2.1. Reliable Codebase
Unit testing leads to a more reliable codebase. When each component is thoroughly tested and proven to work as expected, you can trust that your theme’s foundation is solid. This confidence is invaluable when you’re pushing updates and enhancements, ensuring that you’re not unknowingly introducing new issues.
2.2. Faster Debugging
Bug hunting can be a time-consuming and frustrating process. However, unit tests can significantly speed up the debugging process. When a test fails, it pinpoints the exact location and nature of the issue, making it easier to isolate and fix the problem. This efficiency becomes particularly apparent as your theme becomes more intricate.
2.3. Documentation Through Testing
Unit tests also serve as a form of documentation. A well-written test clearly outlines how a particular component is intended to function. This documentation can be invaluable when you or other developers revisit the codebase after some time. Unit tests provide insights into the expected behavior of your theme’s components, making it easier to understand their purpose and implementation.
3. Implementing Unit Testing in Your WordPress Theme Development
3.1. Choosing a Testing Framework
To get started with unit testing in WordPress theme development, you need a testing framework. One popular choice is PHPUnit, a widely used testing framework for PHP. PHPUnit offers a suite of tools for writing and executing tests, making it an ideal choice for unit testing in the WordPress ecosystem.
3.2. Writing Testable Code
Before you can write unit tests, your code needs to be structured in a way that’s conducive to testing. This involves adhering to best practices like separating concerns, using meaningful variable names, and avoiding tightly coupled code. By writing clean, modular code, you create components that can be easily isolated and tested.
3.3. Creating Test Suites
A test suite is a collection of individual tests that evaluate different aspects of your code. For a WordPress theme, you might have test suites that focus on template files, theme options, and custom functions. Each test within a suite should focus on a specific functionality, mimicking real-world scenarios to ensure thorough testing.
3.4. Writing Unit Tests
Let’s take a look at a simple example. Suppose you have a custom function that calculates the total price of items in a shopping cart. Your test might look something like this:
php class ShoppingCartTest extends WP_UnitTestCase { public function test_calculate_total_price() { $cart = new ShoppingCart(); $cart->add_item(100); // Adding an item with price $100 $cart->add_item(50); // Adding an item with price $50 $total_price = $cart->calculate_total_price(); $this->assertEquals(150, $total_price); } }
In this example, we’re testing the calculate_total_price method of the ShoppingCart class. We create an instance of the ShoppingCart class, add items with prices, and then assert that the calculated total price matches our expectation.
3.5. Running Tests
Once you’ve written your unit tests, you can run them using PHPUnit’s command-line interface. PHPUnit will execute your tests, providing feedback on which tests pass and which ones fail. This iterative process allows you to refine your code and tests until all components are functioning correctly.
Conclusion
In the realm of WordPress theme development, unit testing is more than just a best practice—it’s a necessity. Unit testing ensures that your theme’s components work as intended, prevents regressions, and enhances collaboration among developers. The benefits of unit testing, such as a reliable codebase, faster debugging, and inherent documentation, cannot be overstated.
By implementing unit testing in your WordPress theme development workflow, you can elevate the quality of your themes and provide users with a seamless and bug-free experience. Remember, unit testing is an investment that pays dividends in the form of higher code quality, reduced development time, and satisfied users.
So, the next time you embark on a WordPress theme development journey, make unit testing an integral part of your process. Your themes, your team, and your users will thank you for it.
Table of Contents
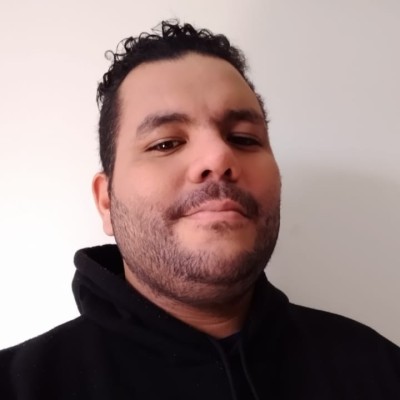
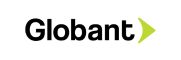