WordPress Multisite: User Management and Roles with Programming
WordPress Multisite is a powerful feature that allows you to manage multiple websites from a single WordPress installation. Whether you’re a site administrator, developer, or content creator, understanding user management and roles within the context of Multisite is essential. In this comprehensive guide, we’ll delve into the intricacies of user roles, permissions, and explore how programming can be leveraged to streamline the user management process across your Multisite network.
Table of Contents
1. Introduction to WordPress Multisite
1.1. What is WordPress Multisite?
WordPress Multisite is a feature that empowers you to create a network of interconnected websites, all managed from a single WordPress installation. This is particularly useful for organizations, businesses, or individuals who need to manage multiple websites while sharing resources such as themes, plugins, and user data.
1.2. Why is Efficient User Management Important?
In a Multisite network, managing users across various websites can quickly become a complex task. Efficient user management ensures that users have the right permissions on each site, preventing unauthorized access and data breaches. Additionally, understanding roles and capabilities becomes crucial when you want to grant users specific rights within the network.
2. User Roles in WordPress Multisite
WordPress Multisite introduces a hierarchical system of user roles, each with specific capabilities. Let’s take a look at the core user roles:
2.1. Super Admin
The Super Admin role has the highest level of control across the entire network. Super Admins can manage network settings, install themes and plugins, and even create new sites. This role is restricted to a few individuals who oversee the entire Multisite network.
2.2. Site Administrator
Each individual site within the network has its own administrators. Site Administrators have control over their specific site, including content management, user roles on that site, and theme/plugin management.
2.3. Editor
Editors have the authority to publish and manage posts and pages created by any user, including themselves. They can moderate comments, manage categories and tags, but they don’t have access to critical site settings.
2.4. Author
Authors can create and manage their own posts, but they can’t edit or publish posts by other users. This role is ideal for content creators who contribute to the site but don’t need broader management privileges.
2.5. Contributor
Contributors can write and manage their own posts, but they can’t publish them. Instead, their content goes into a pending review state until an Editor or Administrator approves and publishes it.
2.6. Subscriber
Subscribers have the least permissions. They can only manage their profile and subscribe to site updates. This role is often used for individuals who want to receive email notifications or access specific member-only content.
3. Permissions and Capabilities
WordPress assigns capabilities to each role, dictating what actions users in those roles can perform. Some capabilities include publishing posts, managing plugins, and moderating comments. If the default capabilities don’t align with your network’s requirements, you can customize them or create new ones.
3.1. Mapping User Capabilities
Understanding the capabilities associated with each role is key to effective user management. For instance, to check if a user has the capability to publish posts, you can use the current_user_can() function:
php if ( current_user_can( 'publish_posts' ) ) { // User can publish posts } else { // User doesn't have the capability to publish posts }
3.2. Adding Custom Capabilities
Adding custom capabilities can extend the default user roles. To add a new capability, use the add_cap() function:
php // Add a custom capability to the Author role $author_role = get_role( 'author' ); $author_role->add_cap( 'edit_others_posts' );
4. Default User Registration and Roles Setup
4.1. Enabling User Registration
WordPress Multisite allows you to control user registration for your network. You can enable user registration in the Network Admin settings under “Registration Settings.” This feature is especially useful when you want users to create accounts across different sites within the network.
4.2. Setting Default User Roles
You can specify the default role given to new users when they register on a site within the network. This can be set in the Network Admin settings under “Default Role” or via code:
php // Set the default role for new users add_filter( 'wpmu_signup_user_notification', function ( $user, $user_email, $key, $meta ) { return 'subscriber'; // Change to desired role }, 10, 4 );
5. Programmatic User Management
Managing users manually can be time-consuming, especially if you have a large network. Programming can help automate user management tasks.
5.1. Creating Users Programmatically
You can programmatically create users using the wp_insert_user() function:
php $new_user = array( 'user_login' => 'newuser', 'user_pass' => 'password', 'user_email' => 'newuser@example.com', ); $user_id = wp_insert_user( $new_user );
5.2. Assigning Roles Programmatically
You can assign roles to users using the add_role() function:
php // Define new role $role = 'custom_role'; $capabilities = array( // ... define capabilities ... ); // Add role with capabilities add_role( $role, 'Custom Role', $capabilities ); // Assign role to user $user = wp_get_current_user(); $user->add_role( $role );
5.3. Removing Users from Sites
To remove a user from a specific site, you can use the remove_user_from_blog() function:
php // Remove user from a site remove_user_from_blog( $user_id, $site_id );
6. Advanced Techniques with Code Samples
6.1. Network-wide Role Synchronization
Keeping user roles consistent across the network can be challenging. Automate this process using hooks, such as when a user role changes on one site, propagate that change across other sites:
php // Sync user role change across the network add_action( 'set_user_role', function ( $user_id, $role, $old_roles ) { // Loop through all sites in the network foreach ( get_sites() as $site ) { switch_to_blog( $site->blog_id ); $user = new WP_User( $user_id ); if ( in_array( $role, $old_roles ) ) { $user->remove_role( $role ); } else { $user->add_role( $role ); } restore_current_blog(); } }, 10, 3 );
6.2. Customizing User Invitation Process
When inviting users to join your network, customize the invitation email content and URL:
php // Customize user invitation email add_filter( 'invite_user_notification_message', function ( $message, $user, $user_email, $key, $meta ) { $url = network_site_url( "wp-activate.php?key=$key" ); $message .= "Click the following link to activate your account: $url"; return $message; }, 10, 5 );
6.3. Automating User Onboarding
Automate user onboarding by granting specific roles and capabilities when a user registers:
php // Automatically assign role and capabilities on registration add_action( 'user_register', function ( $user_id ) { $user = new WP_User( $user_id ); $user->set_role( 'contributor' ); $user->add_cap( 'edit_posts' ); }, 10, 1 );
7. Best Practices for User Management in WordPress Multisite
7.1. Regular Auditing of User Roles
Periodically review and adjust user roles to ensure that users have appropriate permissions. Remove users who no longer need access to maintain a clean and secure network.
7.2. Educating Site Administrators
Train site administrators on user management best practices to maintain consistency and security across the network. Educated administrators can handle day-to-day tasks more effectively.
7.3. Keeping Plugins and Themes Updated
Outdated plugins and themes can pose security risks. Regularly update them to prevent vulnerabilities that might be exploited to gain unauthorized access.
Conclusion
WordPress Multisite’s user management and roles system is an essential component of running a successful network of interconnected websites. Understanding user roles, permissions, and utilizing programming techniques can significantly streamline user management tasks and enhance the overall security and efficiency of your Multisite network. By following best practices and staying informed about the latest developments, you’ll be better equipped to create and manage a thriving WordPress Multisite network.
In conclusion, mastering user management and roles in WordPress Multisite is not only a technical endeavor but also a strategic one that ensures the seamless operation of your network. Whether you’re an administrator, developer, or content creator, a solid grasp of these concepts will empower you to create a robust and organized Multisite ecosystem.
Table of Contents
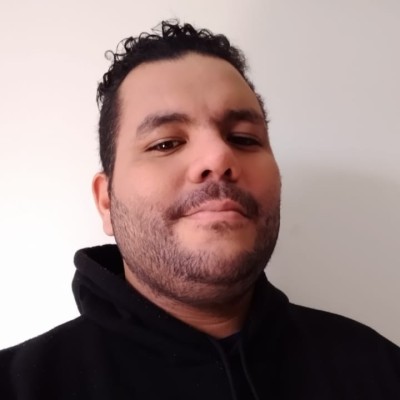
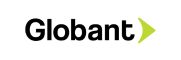