Data Binding in Android: The Key to Smooth and Efficient UIs
In the realm of Android development, keeping the user interface (UI) in sync with the application’s data model can be a cumbersome task. However, with the introduction of Android Data Binding, this process has been greatly simplified. This powerful library enables developers to create more robust, testable, and maintainable applications by seamlessly binding UI components in layouts to data sources. In this blog post, we will delve into how Android Data Binding eases UI updates and explore its benefits through examples. You can hire Android developers for your projects to ensure greater success.
Table of Contents
1. What is Android Data Binding?
Android Data Binding is a support library that allows developers to bind UI components in their XML layouts to data sources in their Android application, using a declarative format rather than programmatically. Introduced in Android Studio 1.3, this library has been a game-changer for many developers. It reduces boilerplate code, enhances readability, and improves performance by minimizing unnecessary UI updates.
2. Key Features:
- Declarative Binding: Allows you to write expressive layout files with less code.
- Automatic UI Updates: UI components automatically update when the bound data changes.
- Bidirectional Binding: Enables two-way data binding, allowing UI elements to both receive and send data changes.
- Event Handling: Simplifies the process of handling user actions.
3. Setting Up Android Data Binding
To use data binding, ensure that your Android Studio is updated and include the data binding library in your `build.gradle` file:
```gradle android { ... dataBinding { enabled = true } } ```
For a comprehensive guide on setting up, refer to the official Android Developers Guide.
4. Implementing Data Binding: A Step-by-Step Example
Let’s dive into an example to illustrate how data binding works:
Step 1: Define a Data Model
First, create a simple data model. For instance, a `User` class:
```java public class User { public final ObservableField<String> name = new ObservableField<>(); public final ObservableField<String> email = new ObservableField<>(); } ```
Step 2: Update the XML Layout
In your XML layout, wrap the layout with a `<layout>` tag and define a variable for your data model:
```xml <layout xmlns:android="http://schemas.android.com/apk/res/android"> <data> <variable name="user" type="com.example.User"/> </data> ... </layout> ```
Step 3: Binding Data to UI Components
Bind the UI components to the data model properties:
```xml <TextView android:text="@{user.name}" android:layout_width="wrap_content" android:layout_height="wrap_content"/> ```
Step 4: Set the Data Model in Your Activity or Fragment
In your `Activity` or `Fragment`, set the data model for the binding:
```java User user = new User(); ActivityMainBinding binding = DataBindingUtil.setContentView(this, R.layout.activity_main); binding.setUser(user); ```
5. Advantages of Using Android Data Binding
- Reduced Boilerplate Code: Data Binding reduces the need to find views and manually update their content.
- Improved Performance: It minimizes unnecessary UI updates, which is crucial for smooth user experiences.
- Enhanced Readability and Maintenance: The separation of layout and logic makes it easier to understand and maintain the code.
- Better Testability: It facilitates easier unit testing of view models and data models.
6. Advanced Data Binding Techniques
6.1 Bidirectional Binding
Android Data Binding also supports bidirectional data binding, allowing UI elements to modify the data and vice versa. This is particularly useful for forms and user inputs.
6.2 Binding Adapters
Custom binding adapters let you add custom logic when binding data to UI components. This opens up a wide array of possibilities for customizing UI behavior and appearance.
For a deeper dive into advanced techniques, explore the [Android Developers Documentation](https://developer.android.com/topic/libraries/data-binding/advanced-binding).
7. Conclusion
Android Data Binding stands as a powerful tool in the arsenal of Android developers, streamlining the process of synchronizing UI and data. It not only enhances the efficiency of code but also contributes to more readable, maintainable, and testable codebases.
You can check out our other blog posts to learn more about Android. We bring you a complete guide titled Elevating Your Skills: Essential Tips and Tricks for Android Development Mastery along with the Demystifying the Android App Lifecycle: An In-depth Exploration of Activity Execution Flow and Android Security: Best Practices to Protect User Data which will help you understand and gain more insight into the Android applications.
To further explore and master Android Data Binding, consider visiting the following resources:
- Android Data Binding Guide – Official guide for beginners.
- Advanced Data Binding – Deep dive into advanced features.
3.Data Binding CodeLab – Hands-on practice through a CodeLab.
Embrace the power of Android Data Binding and elevate your Android development to new heights!
Table of Contents
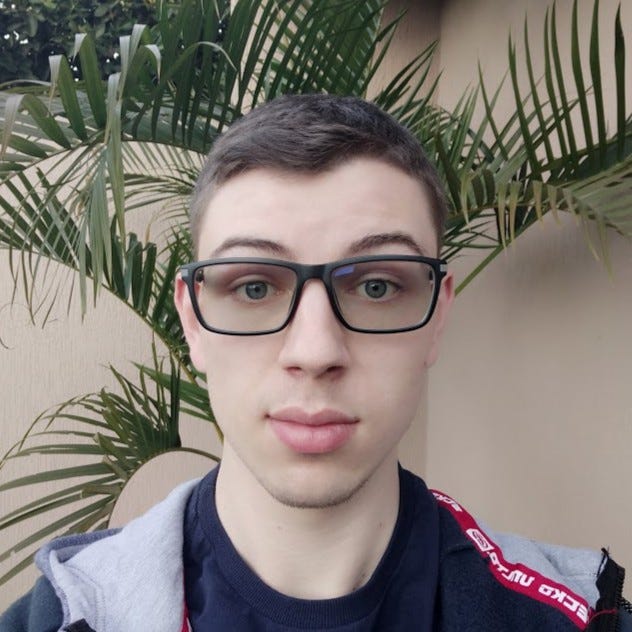
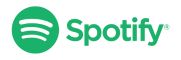