The Android Keystore Chronicles: Protecting Your App’s Valuables
In today’s digital age, data security is paramount. As mobile app developers, it’s our responsibility to protect the sensitive information entrusted to our applications. Android, being the most popular mobile operating system, offers robust tools and frameworks to ensure the security of user data. One of the essential tools in Android’s arsenal is the Android Keystore, which plays a crucial role in safeguarding sensitive data. In this blog post, we will explore what the Android Keystore is, how it works, and provide practical examples of using it to secure user data in your Android applications. You can hire Android developers for your projects to ensure greater success.
Table of Contents
1. Understanding Android Keystore
The Android Keystore is a system service provided by the Android operating system that allows developers to store and manage cryptographic keys securely. It provides a secure storage area for keys and ensures that these keys cannot be extracted from the device, even if it’s rooted. Android Keystore is essential for various security-related tasks, such as encryption, decryption, digital signatures, and more.
2. Key Characteristics of Android Keystore
- Hardware-backed: Android Keystore leverages the hardware-based security features of modern Android devices, making it extremely difficult for malicious actors to access or extract keys.
- Key Generation: It offers APIs to generate and store cryptographic keys within the secure hardware enclave.
- Key Usage: Developers can specify the intended usage of keys, such as encryption, decryption, or digital signing, ensuring that keys are only used for their designated purpose.
- Biometric Authentication: Android Keystore can be configured to require biometric authentication (e.g., fingerprint or face recognition) before granting access to keys, adding an additional layer of security.
Now that we have a basic understanding of Android Keystore, let’s delve into some practical examples of how it can be used to secure user data.
Example 1: Encrypting User Data
Imagine you have a mobile app that stores sensitive user data, such as passwords or personal information. You want to ensure that this data is securely encrypted and can only be decrypted when needed. Android Keystore is the perfect tool for this task.
Here’s a simplified example of how to encrypt and decrypt user data using Android Keystore:
```java // Generate a new encryption key in the Android Keystore KeyGenerator keyGenerator = KeyGenerator.getInstance(KeyProperties.KEY_ALGORITHM_AES, "AndroidKeyStore"); KeyGenParameterSpec keyGenSpec = new KeyGenParameterSpec.Builder("my_key_alias", KeyProperties.PURPOSE_ENCRYPT | KeyProperties.PURPOSE_DECRYPT) .setBlockModes(KeyProperties.BLOCK_MODE_CBC) .setEncryptionPaddings(KeyProperties.ENCRYPTION_PADDING_PKCS7) .setUserAuthenticationRequired(true) // Requires user authentication to use the key .build(); keyGenerator.init(keyGenSpec); SecretKey secretKey = keyGenerator.generateKey(); // Encrypt user data Cipher cipher = Cipher.getInstance("AES/CBC/PKCS7Padding"); cipher.init(Cipher.ENCRYPT_MODE, secretKey); byte[] encryptedData = cipher.doFinal(userData); // Decrypt user data cipher.init(Cipher.DECRYPT_MODE, secretKey); byte[] decryptedData = cipher.doFinal(encryptedData); ```
In this example, we generate an encryption key in the Android Keystore, use it to encrypt user data, and then decrypt the data when needed. The key is protected by requiring user authentication, ensuring that only authorized users can access it.
Example 2: Securing API Keys
Many Android apps rely on external APIs for various functionalities. API keys are often used to authenticate and access these APIs. Storing API keys securely is crucial to prevent unauthorized access. Android Keystore can help in this scenario as well.
Here’s an example of how to securely store an API key using Android Keystore:
```java // Storing an API key String apiKey = "your_api_key"; KeyStore keyStore = KeyStore.getInstance("AndroidKeyStore"); keyStore.load(null); KeyStore.SecretKeyEntry keyEntry = (KeyStore.SecretKeyEntry) keyStore.getEntry("api_key_alias", null); SecretKey secretKey = keyEntry.getSecretKey(); // Retrieving the API key Cipher cipher = Cipher.getInstance(KeyProperties.KEY_ALGORITHM_AES + "/" + KeyProperties.BLOCK_MODE_CBC + "/" + KeyProperties.ENCRYPTION_PADDING_PKCS7); cipher.init(Cipher.DECRYPT_MODE, secretKey); byte[] decryptedApiKey = cipher.doFinal(apiKey.getBytes()); String retrievedApiKey = new String(decryptedApiKey); ```
In this example, we store the API key in the Android Keystore and retrieve it when needed by decrypting it using the stored key. This ensures that the API key remains secure even if the device is compromised.
Example 3: Digital Signatures
Digital signatures are essential for verifying the authenticity and integrity of data. Android Keystore can be used to generate and manage keys for digital signatures.
Here’s a simplified example of how to generate a digital signature using Android Keystore:
```java // Generate a key pair for digital signatures KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance(KeyProperties.KEY_ALGORITHM_RSA, "AndroidKeyStore"); KeyGenParameterSpec keyGenSpec = new KeyGenParameterSpec.Builder("signature_key_alias", KeyProperties.PURPOSE_SIGN) .setDigests(KeyProperties.DIGEST_SHA256) .setUserAuthenticationRequired(true) // Requires user authentication to use the key .build(); keyPairGenerator.initialize(keyGenSpec); KeyPair keyPair = keyPairGenerator.generateKeyPair(); // Create a digital signature Signature signature = Signature.getInstance("SHA256withRSA"); signature.initSign(keyPair.getPrivate()); signature.update(dataToSign); byte[] digitalSignature = signature.sign(); ```
In this example, we generate a key pair for digital signatures in the Android Keystore, create a digital signature for some data, and ensure that user authentication is required to use the key for signing.
Conclusion
Securing user data is a critical aspect of Android app development. The Android Keystore provides a robust and hardware-backed solution for managing cryptographic keys and ensuring the security of sensitive information. In this blog post, we explored the basics of Android Keystore and provided practical examples of how it can be used to encrypt user data, secure API keys, and generate digital signatures.
By implementing these techniques and leveraging the power of Android Keystore, you can enhance the security of your Android applications, protect user data from unauthorized access, and build trust with your users.
For further reading and in-depth documentation on Android Keystore, be sure to check out the official Android Developers website Android Keystore Documentation and this in-depth tutorial on Using the Android Keystore.
You can check out our other blog posts to learn more about Android. We bring you a complete guide titled Elevating Your Skills: Essential Tips and Tricks for Android Development Mastery along with the Demystifying the Android App Lifecycle: An In-depth Exploration of Activity Execution Flow and Android Security: Best Practices to Protect User Data which will help you understand and gain more insight into the Android applications.
Table of Contents
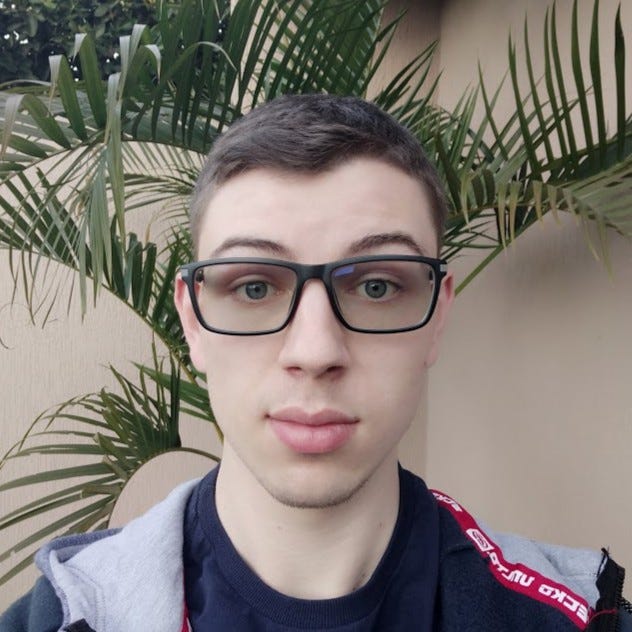
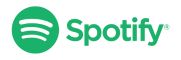