Crash-Proof Your Android App: A Complete Guide to Firebase Crashlytics
In the fast-paced world of mobile app development, ensuring the stability and reliability of your Android application is paramount. App crashes can lead to user frustration, negative reviews, and even loss of revenue. This is where Firebase Crashlytics comes to the rescue. Firebase Crashlytics is a robust crash reporting tool offered by Google’s Firebase platform, specifically designed to help developers identify, track, and fix app crashes efficiently. In this blog post, we’ll dive deep into how Firebase Crashlytics works, how to integrate it into your Android app, and how to utilize its powerful features for tracking and reporting crashes effectively. You can hire Android developers for your projects to ensure greater success.
Table of Contents
1. What is Firebase Crashlytics?
Firebase Crashlytics is a comprehensive crash reporting tool that provides real-time insights into your Android app’s stability. It helps you identify and prioritize the most critical issues by monitoring your app for crashes and exceptions. Firebase Crashlytics not only tracks crashes but also provides detailed crash reports, making it easier for developers to understand the root cause of issues and take prompt action.
2. Key Features of Firebase Crashlytics
- Real-time Crash Reporting: Firebase Crashlytics offers real-time crash reporting, ensuring that you are immediately notified when a crash occurs in your app. This enables you to address issues quickly and minimize their impact on users.
- Detailed Crash Reports: Each crash report generated by Firebase Crashlytics includes valuable information such as stack traces, device information, and user data. This data is crucial for diagnosing the problem accurately.
- Prioritization: Crashlytics automatically ranks crashes based on their severity and impact, helping you prioritize which issues to tackle first. This feature ensures that you focus your efforts on the most critical problems affecting your users.
- Custom Logging: You can add custom logs to your crash reports to provide additional context and information about the app’s state leading up to the crash. This can be immensely helpful in understanding the root cause of issues.
- Integration with Firebase: Firebase Crashlytics seamlessly integrates with other Firebase services, allowing you to combine crash data with other analytics and user engagement data for a holistic view of your app’s performance.
3. Setting up Firebase Crashlytics in your Android App
Integrating Firebase Crashlytics into your Android app is a straightforward process. Follow these steps to get started:
Step 1: Create a Firebase Project
If you haven’t already, create a Firebase project on the Firebase Console. Once your project is set up, add your Android app to it.
Step 2: Add Firebase to your Android App
To integrate Firebase Crashlytics into your Android app, you’ll need to add the Firebase SDK to your project. You can do this by adding the following dependencies to your app-level build.gradle file:
```gradle implementation 'com.google.firebase:firebase-core:YOUR_FIREBASE_VERSION' implementation 'com.google.firebase:firebase-crashlytics:YOUR_FIREBASE_VERSION' ```
Make sure to replace `YOUR_FIREBASE_VERSION` with the appropriate version number.
Step 3: Initialize Firebase in your App
In your app’s `Application` class, initialize Firebase by adding the following code:
```java import com.google.firebase.FirebaseApp; import com.google.firebase.analytics.FirebaseAnalytics; import com.google.firebase.crashlytics.FirebaseCrashlytics; public class MyApplication extends Application { @Override public void onCreate() { super.onCreate(); // Initialize Firebase FirebaseApp.initializeApp(this); // Enable Firebase Crashlytics FirebaseCrashlytics.getInstance().setCrashlyticsCollectionEnabled(true); } } ```
Step 4: Test Crash Reporting
To verify that Firebase Crashlytics is working correctly, you can intentionally trigger a crash in your app and then check the Firebase Console for the crash report. Here’s an example of how to do this:
```java public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // Simulate a crash for testing purposes findViewById(R.id.crash_button).setOnClickListener(view -> { throw new RuntimeException("This is a crash for testing"); }); } } ```
After triggering the crash, you should see the crash report in your Firebase project’s Crashlytics dashboard.
4. Analyzing Crash Reports
Firebase Crashlytics provides detailed crash reports that are essential for diagnosing and fixing issues. Let’s explore some key components of a crash report:
- Stack Trace: The stack trace provides information about the sequence of method calls leading up to the crash. It helps you pinpoint the exact location in your code where the issue occurred.
- Device Information: Crash reports include device-specific information, such as the device model, OS version, and screen size. This information can help you identify patterns related to specific devices or OS versions.
- User Data: Firebase Crashlytics can capture user data if you choose to include it in your crash reports. This can be valuable for understanding the impact of crashes on different user segments.
- Custom Logs: You can add custom logs to your crash reports to provide additional context. For example, you can log user actions or specific app events leading up to the crash.
5. Using Firebase Crashlytics to Improve App Stability
Firebase Crashlytics not only helps you identify and diagnose crashes but also enables you to improve your app’s overall stability. Here are some best practices to follow:
- Monitor Crash Trends: Regularly review your crash reports in the Firebase Console to identify recurring issues. Prioritize fixing crashes that impact a large number of users or have a high crash rate.
- React Swiftly: When a critical issue arises, act promptly to release a fix. Firebase Crashlytics provides you with real-time insights, so you can address problems as they occur.
- Utilize Custom Logs: Take advantage of custom logging to capture relevant data leading up to a crash. This can help you recreate the scenario and identify the root cause more efficiently.
- Test Thoroughly: Before releasing updates or new features, thoroughly test your app to catch potential issues early. Firebase Crashlytics can help you catch any regressions in your code.
Conclusion
Firebase Crashlytics is an indispensable tool for Android app developers who prioritize stability and user experience. By integrating Crashlytics into your app, you gain access to real-time crash reporting, detailed crash reports, and valuable insights that can help you identify and resolve issues efficiently. Remember to monitor crash trends, react swiftly to critical issues, and use custom logs to enhance your debugging process. With Firebase Crashlytics, you can ensure that your Android app maintains a high level of stability, keeping your users happy and engaged.
To learn more about Firebase Crashlytics, visit the official Firebase Crashlytics documentation.
By following best practices and utilizing the power of Firebase Crashlytics, you can create a more robust and reliable Android app that delights users and keeps them coming back for more.
You can check out our other blog posts to learn more about Android. We bring you a complete guide titled Elevating Your Skills: Essential Tips and Tricks for Android Development Mastery along with the Demystifying the Android App Lifecycle: An In-depth Exploration of Activity Execution Flow and Android Security: Best Practices to Protect User Data which will help you understand and gain more insight into the Android applications.
Table of Contents
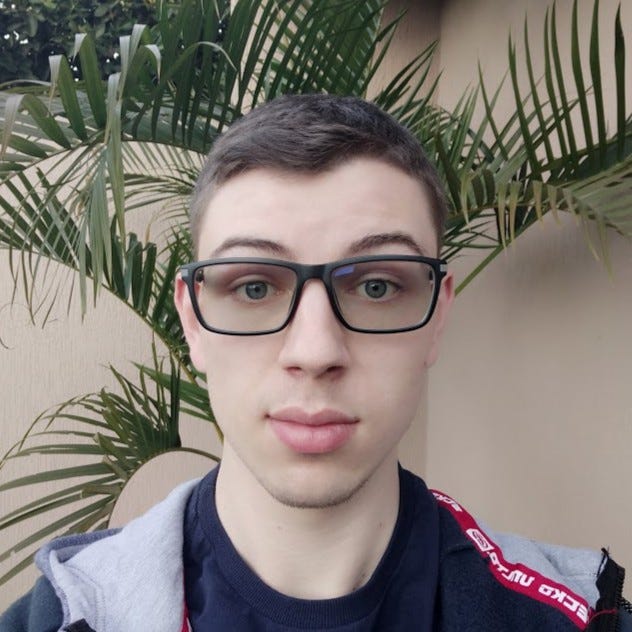
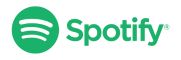