Elevate User Engagement: How to Implement Real-Time Features in Android
In the world of mobile app development, real-time features have become essential for creating engaging and interactive applications. Whether it’s a messaging app, a collaborative tool, or a live-tracking system, the ability to update data in real-time is a game-changer. One powerful tool for achieving this functionality in Android apps is Firebase Realtime Database. You can hire Android developers for your projects to ensure greater success. In this blog post, we’ll explore the concept of real-time data and dive into how to use Firebase Realtime Database to build real-time apps. We’ll also provide examples to illustrate its capabilities.
Table of Contents
1. Understanding Real-Time Data
Before we get into Firebase Realtime Database, let’s understand what real-time data means. Real-time data is information that is updated and made available to users immediately as it changes. In the context of mobile apps, real-time data ensures that users always see the latest information without needing to refresh or manually update the app.
2. Examples of Real-Time Apps
- Chat Applications: Messaging apps like WhatsApp and Slack use real-time data to deliver messages instantly to users in their conversations.
- Collaborative Tools: Tools like Google Docs or Figma enable real-time collaboration, where multiple users can simultaneously edit a document or design in real-time.
- Live Tracking: Apps like Uber and Lyft provide real-time tracking of drivers and their locations.
Now, let’s explore how Firebase Realtime Database can help you achieve real-time functionality in your Android apps.
3. Introducing Firebase Realtime Database
Firebase Realtime Database is a cloud-hosted NoSQL database provided by Google as a part of the Firebase suite. It allows you to store and synchronize data in real-time across all connected clients, making it a perfect choice for building real-time Android apps. Here are some key features of Firebase Realtime Database:
- Real-Time Sync: Any change made to the database is instantly synchronized across all connected devices. This ensures that users always have the latest data.
- No Backend Development Required: Firebase Realtime Database eliminates the need for server-side code or complex backend infrastructure. Developers can focus on the client-side code, making development faster and more efficient.
- Offline Support: Firebase Realtime Database provides offline support, which means your app can continue to work even when the device is not connected to the internet. Changes made offline will be synchronized when the connection is reestablished.
Now, let’s dive into some examples to demonstrate how to use Firebase Realtime Database in Android apps.
Example 1: Real-Time Chat Application
Imagine you’re building a chat application, and you want to ensure that messages are delivered instantly to all users. Firebase Realtime Database makes this a breeze.
- Initialize Firebase: First, initialize Firebase in your Android project. Follow the [official Firebase documentation](https://firebase.google.com/docs/android/setup) for detailed instructions.
- Create a Chat Room: In your database, create a “Chat Rooms” node where each chat room has a unique identifier. Under each chat room, store messages with a timestamp.
- Listen for Changes: Use Firebase’s real-time listeners to observe changes to the chat room. Whenever a new message is added, update the chat interface in real-time.
Example Code:
```java DatabaseReference chatRoomRef = FirebaseDatabase.getInstance().getReference("ChatRooms/roomId"); chatRoomRef.addChildEventListener(new ChildEventListener() { @Override public void onChildAdded(@NonNull DataSnapshot snapshot, @Nullable String previousChildName) { // Handle new message added in real-time } // ... }); ```
Example 2: Real-Time Collaboration Tool
Suppose you’re building a collaborative drawing app where multiple users can draw on a shared canvas simultaneously. Firebase Realtime Database can help you achieve real-time collaboration effortlessly.
- Initialize Firebase: Follow the same initialization steps as in the chat app example.
- Shared Canvas Data: Store the drawing data (lines, shapes, etc.) in your database under a specific node.
- Real-Time Drawing: Use Firebase’s real-time listeners to observe changes to the drawing data. As users draw on the canvas, update the canvas in real-time for all connected users.
Example Code:
```java DatabaseReference canvasRef = FirebaseDatabase.getInstance().getReference("SharedCanvas/canvasId"); canvasRef.addValueEventListener(new ValueEventListener() { @Override public void onDataChange(@NonNull DataSnapshot snapshot) { // Update the canvas with real-time changes } // ... }); ```
Example 3: Real-Time Location Tracking
Suppose you’re developing a food delivery app and need to track the location of delivery drivers in real-time. Firebase Realtime Database can help you achieve this as well.
- Initialize Firebase: Initialize Firebase in your project as before.
- Driver Locations: Store the location data (latitude and longitude) of each driver in your database.
- Real-Time Tracking: Use Firebase’s real-time listeners to observe changes in driver locations. Display the live location of drivers on the map for users.
Example Code:
```java DatabaseReference driverLocationRef = FirebaseDatabase.getInstance().getReference("DriverLocations/driverId"); driverLocationRef.addValueEventListener(new ValueEventListener() { @Override public void onDataChange(@NonNull DataSnapshot snapshot) { // Update the driver's location on the map in real-time } // ... }); ```
Conclusion
Firebase Realtime Database is a powerful tool for building real-time Android apps. Whether you’re creating a chat application, a collaborative tool, or a location-tracking system, Firebase makes it easy to implement real-time features without the need for complex backend infrastructure. By following the examples provided and referring to the [Firebase documentation](https://firebase.google.com/docs/database), you can start building your own real-time Android apps today.
Incorporating real-time functionality can significantly enhance the user experience of your Android apps, making them more engaging and interactive. Firebase Realtime Database empowers developers to bring these features to life with ease.
To learn more about Firebase Realtime Database and explore its capabilities further, check out the official Firebase documentation and the Firebase Console.
You can check out our other blog posts to learn more about Android. We bring you a complete guide titled Elevating Your Skills: Essential Tips and Tricks for Android Development Mastery along with the Demystifying the Android App Lifecycle: An In-depth Exploration of Activity Execution Flow and Android Security: Best Practices to Protect User Data which will help you understand and gain more insight into the Android applications.
Table of Contents
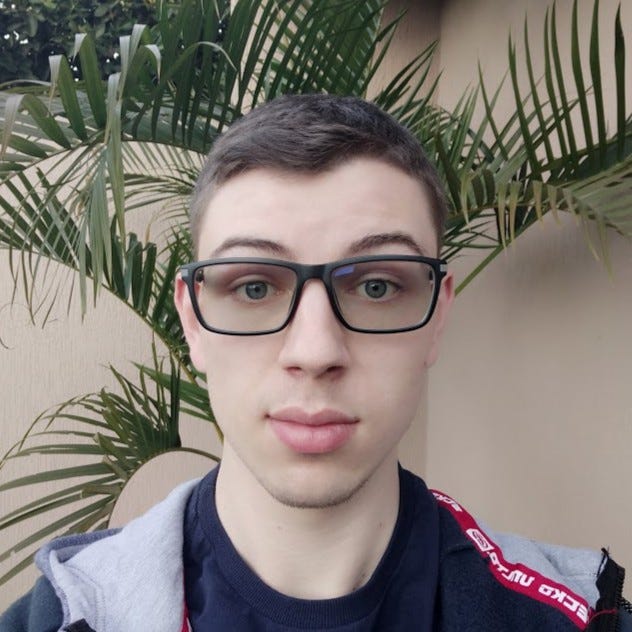
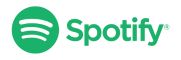