Monetization Made Simple: In-App Purchases with Google Play Billing Library
In-app purchases have become a crucial revenue stream for mobile app developers, allowing them to offer premium features, digital goods, and subscriptions to users. If you’re developing an Android app and want to monetize it effectively, integrating in-app purchases is a must. Google Play Billing Library is the go-to tool for implementing in-app purchases seamlessly within your Android application. In this blog post, we will explore the Google Play Billing Library, understand its key features, and provide examples of how to integrate it into your Android app. You can hire Android developers for your projects to ensure greater success.
Table of Contents
1. Understanding Google Play Billing Library
Google Play Billing Library is a powerful tool provided by Google to facilitate the implementation of in-app purchases in Android applications. It streamlines the process of selling digital goods and subscriptions, making it easier for developers to monetize their apps.
Key Features of Google Play Billing Library:
- Simplified Purchase Flow: The library provides a straightforward and consistent purchase flow, allowing users to make purchases with ease.
- Local Caching: Google Play Billing Library caches the purchase data, reducing the need for repeated network calls and improving performance.
- Security: It ensures secure transactions by handling cryptographic operations and verification processes, preventing unauthorized access to premium content.
- Subscription Management: The library offers robust subscription management features, including handling renewals, upgrades, and cancellations.
Now, let’s dive into the implementation of Google Play Billing Library with examples.
2. Prerequisites
Before you start implementing in-app purchases, you need to set up your development environment and configure your app in the Google Play Console. Additionally, ensure that you have the latest version of Google Play Billing Library in your project’s build.gradle file.
```groovy implementation 'com.android.billingclient:billing:4.0.0' ```
3. Setting up Google Play Billing Client
To start using Google Play Billing Library, you need to create an instance of the BillingClient and establish a connection to the Google Play Store. Here’s a code snippet to set up the BillingClient:
```java import com.android.billingclient.api.BillingClient; import com.android.billingclient.api.BillingClientStateListener; import com.android.billingclient.api.PurchasesUpdatedListener; // ... private BillingClient billingClient; billingClient = BillingClient.newBuilder(context) .setListener(purchasesUpdatedListener) .build(); billingClient.startConnection(new BillingClientStateListener() { @Override public void onBillingSetupFinished(BillingResult billingResult) { if (billingResult.getResponseCode() == BillingClient.BillingResponseCode.OK) { // BillingClient is ready } } @Override public void onBillingServiceDisconnected() { // Handle billing service disconnect } }); ```
4. Implement PurchasesUpdatedListener
The PurchasesUpdatedListener handles the result of purchase operations and is a crucial component of your in-app purchase implementation. Here’s an example of how to implement it:
```java PurchasesUpdatedListener purchasesUpdatedListener = new PurchasesUpdatedListener() { @Override public void onPurchasesUpdated(BillingResult billingResult, List<Purchase> purchases) { if (billingResult.getResponseCode() == BillingClient.BillingResponseCode.OK && purchases != null) { for (Purchase purchase : purchases) { // Handle the purchase } } else if (billingResult.getResponseCode() == BillingClient.BillingResponseCode.USER_CANCELED) { // Handle user cancellation } else { // Handle other errors } } }; ```
5. Querying Available SkuDetails
Before displaying in-app purchase options to users, you need to retrieve the SkuDetails of the products or subscriptions you want to sell. Here’s an example of how to query SkuDetails:
```java List<String> skuList = new ArrayList<>(); skuList.add("premium_upgrade"); skuList.add("monthly_subscription"); SkuDetailsParams params = SkuDetailsParams.newBuilder() .setSkusList(skuList) .setType(BillingClient.SkuType.INAPP) // For one-time purchases // .setType(BillingClient.SkuType.SUBS) // For subscriptions .build(); billingClient.querySkuDetailsAsync(params, new SkuDetailsResponseListener() { @Override public void onSkuDetailsResponse(BillingResult billingResult, List<SkuDetails> skuDetailsList) { if (billingResult.getResponseCode() == BillingClient.BillingResponseCode.OK && skuDetailsList != null) { // Display available products/subscriptions to the user } } }); ```
6. Launching the Purchase Flow
When the user selects a product or subscription, you can launch the purchase flow. Here’s how to do it:
```java String skuId = "premium_upgrade"; BillingFlowParams billingFlowParams = BillingFlowParams.newBuilder() .setSku(skuId) .setType(BillingClient.SkuType.INAPP) // For one-time purchases // .setType(BillingClient.SkuType.SUBS) // For subscriptions .build(); int responseCode = billingClient.launchBillingFlow(activity, billingFlowParams); ```
7. Handling Purchases
Once a purchase is successful, it’s essential to handle it correctly. Here’s an example of how to acknowledge and consume purchases:
```java for (Purchase purchase : purchases) { if (purchase.getPurchaseState() == Purchase.PurchaseState.PURCHASED) { // Acknowledge the purchase (for one-time purchases) AcknowledgePurchaseParams acknowledgePurchaseParams = AcknowledgePurchaseParams.newBuilder() .setPurchaseToken(purchase.getPurchaseToken()) .build(); billingClient.acknowledgePurchase(acknowledgePurchaseParams, new AcknowledgePurchaseResponseListener() { @Override public void onAcknowledgePurchaseResponse(BillingResult billingResult) { if (billingResult.getResponseCode() == BillingClient.BillingResponseCode.OK) { // Purchase acknowledged successfully } } }); // Consume the purchase (for consumable products) ConsumeParams consumeParams = ConsumeParams.newBuilder() .setPurchaseToken(purchase.getPurchaseToken()) .build(); billingClient.consumeAsync(consumeParams, new ConsumeResponseListener() { @Override public void onConsumeResponse(BillingResult billingResult, String purchaseToken) { if (billingResult.getResponseCode() == BillingClient.BillingResponseCode.OK) { // Purchase consumed successfully } } }); } } ```
Conclusion
Incorporating in-app purchases into your Android app using Google Play Billing Library can be a profitable endeavor. By following the steps outlined in this post, you can implement a secure and user-friendly in-app purchase system that offers premium content or subscriptions to your users.
External Resources:
3.Android Developers Blog – Monetize your app
By leveraging the capabilities of Google Play Billing Library, you can effectively monetize your Android app.
You can check out our other blog posts to learn more about Android. We bring you a complete guide titled Elevating Your Skills: Essential Tips and Tricks for Android Development Mastery along with the Demystifying the Android App Lifecycle: An In-depth Exploration of Activity Execution Flow and Android Security: Best Practices to Protect User Data which will help you understand and gain more insight into the Android applications.
Table of Contents
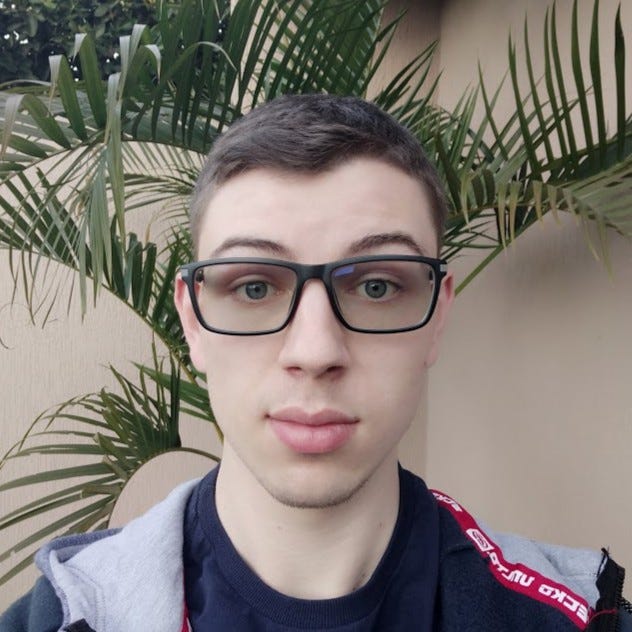
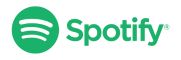