From Fragments to Flow: Building Single Activity Apps on Android
In the world of Android app development, there’s a powerful tool that has been gaining popularity over the years: the Android Navigation Component. This component simplifies the way you handle navigation within your app, making it easier to create a seamless user experience. One of the exciting approaches is to build a single-activity app using the Navigation Component, and in this blog post, we will explore how to do just that. You can hire Android developers for your projects to ensure greater success.
Table of Contents
1. What is a Single Activity App?
Traditionally, Android apps are built with multiple activities, each representing a different screen or functionality. However, as apps have become more complex, managing multiple activities can be challenging. This is where the concept of a single activity app comes in.
A single activity app, as the name suggests, relies on only one main activity to host all the different screens or fragments. This architectural pattern offers several advantages, including improved performance, reduced memory consumption, and simplified navigation. To implement a single activity app effectively, the Android Navigation Component plays a crucial role.
2. Setting Up the Android Navigation Component
Before we dive into creating a single activity app, let’s make sure we have the Android Navigation Component set up in our project. You can do this by adding the following dependencies to your app-level `build.gradle` file:
```gradle dependencies { implementation "androidx.navigation:navigation-fragment-ktx:2.4.0" implementation "androidx.navigation:navigation-ui-ktx:2.4.0" } ```
Once the dependencies are in place, we need to define a navigation graph. A navigation graph is an XML file that represents the different screens and the navigation between them. You can create a navigation graph by right-clicking on the `res` directory, selecting “New,” and then “Android Resource File.” Choose “Navigation” as the resource type and give it a name, like `nav_graph.xml`.
3. Building the Main Activity
Now that we have the Android Navigation Component set up, let’s create our main activity. This activity will serve as the container for all the fragments in our single activity app.
```kotlin class MainActivity : AppCompatActivity() { private lateinit var navController: NavController override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // Initialize the NavController navController = findNavController(R.id.nav_host_fragment) // Set up the ActionBar with the Navigation Component setSupportActionBar(findViewById(R.id.toolbar)) setupActionBarWithNavController(navController) } // Handle Up button navigation override fun onSupportNavigateUp(): Boolean { return navController.navigateUp() || super.onSupportNavigateUp() } } ```
In this code, we create our `MainActivity` class and initialize the `NavController` using the `findNavController` method. We also set up the ActionBar to work with the Navigation Component, enabling the back arrow for navigation.
4. Creating Fragments
With the main activity set up, we can now create fragments to represent the different screens of our app. Let’s create a couple of example fragments, `HomeFragment` and `ProfileFragment`.
```kotlin class HomeFragment : Fragment(R.layout.fragment_home) { // Fragment logic goes here } class ProfileFragment : Fragment(R.layout.fragment_profile) { // Fragment logic goes here } ```
In these fragments, we specify the layout resource associated with each fragment. You can add your fragment logic to these classes as needed.
5. Defining Navigation Destinations
Next, we need to define our navigation destinations in the `nav_graph.xml` file. Open this file, and you can start adding fragments as destinations.
```xml <fragment android:id="@+id/homeFragment" android:name="com.example.app.HomeFragment" android:label="Home" tools:layout="@layout/fragment_home" /> <fragment android:id="@+id/profileFragment" android:name="com.example.app.ProfileFragment" android:label="Profile" tools:layout="@layout/fragment_profile" /> ```
These `<fragment>` elements represent the `HomeFragment` and `ProfileFragment`. You can specify their IDs, class names, and labels. The `tools:layout` attribute is used for design-time previews and should reference the fragment’s layout XML file.
6. Setting Up Navigation Actions
Now that we have defined our destinations, we need to set up navigation actions to define how the user can move between these fragments. In the `nav_graph.xml` file, you can add `<action>` elements to specify navigation actions.
```xml <action android:id="@+id/action_home_to_profile" app:destination="@id/profileFragment" /> <action android:id="@+id/action_profile_to_home" app:destination="@id/homeFragment" /> ```
These actions define transitions from one fragment to another. For example, the first action (`action_home_to_profile`) takes the user from the HomeFragment to the ProfileFragment.
7. Navigating Between Fragments
With the navigation destinations and actions in place, we can now navigate between fragments in our app. To do this, we use the `NavController` that we initialized in the `MainActivity`.
```kotlin // Navigating from HomeFragment to ProfileFragment view.findViewById<Button>(R.id.button).setOnClickListener { findNavController().navigate(R.id.action_home_to_profile) } ```
In this example, we use the `navigate` method to perform the navigation action defined in the `nav_graph.xml` file. You can trigger these actions in response to user interactions, such as button clicks or menu selections.
8. Handling Back Navigation
One of the benefits of using the Navigation Component in a single activity app is that it simplifies back navigation. The `onSupportNavigateUp` method we added to the `MainActivity` handles the Up button in the ActionBar, automatically navigating back to the previous fragment in the stack.
```kotlin // Handle Up button navigation override fun onSupportNavigateUp(): Boolean { return navController.navigateUp() || super.onSupportNavigateUp() } ```
This makes it easy to maintain a consistent and user-friendly navigation experience.
9. External Resources for Further Learning
If you want to dive deeper into Android Navigation Component and building single activity apps, here are some external resources that can provide more in-depth information:
Conclusion
Building a single activity app using the Android Navigation Component can greatly simplify the navigation and organization of your Android applications. With a single activity as the central hub and fragments representing different screens, you can create a more efficient and user-friendly app architecture. By defining navigation destinations and actions, you can effortlessly move between fragments, and the built-in back navigation handling ensures a smooth user experience. Take advantage of this powerful tool in your next Android project and enjoy the benefits of simplified navigation and improved app performance.
You can check out our other blog posts to learn more about Android. We bring you a complete guide titled Elevating Your Skills: Essential Tips and Tricks for Android Development Mastery along with the Demystifying the Android App Lifecycle: An In-depth Exploration of Activity Execution Flow and Android Security: Best Practices to Protect User Data which will help you understand and gain more insight into the Android applications.
Table of Contents
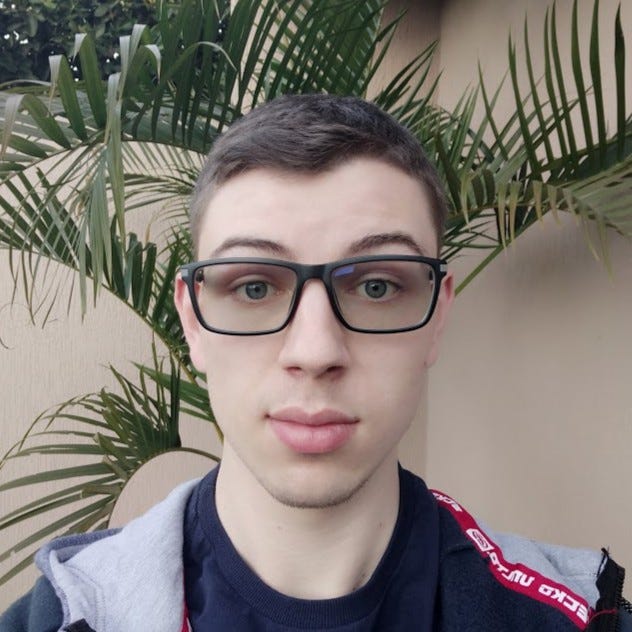
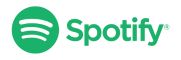