Say Goodbye to Manual Testing: Embrace Espresso for Android
In today’s fast-paced world of mobile app development, ensuring the reliability and quality of your Android application is essential. One of the critical aspects of this process is User Interface (UI) testing. Manual testing can be time-consuming and error-prone, especially as your app grows in complexity. That’s where automated UI testing tools like Espresso come to the rescue. You can hire Android developers for your projects to ensure greater success. In this blog post, we will explore Espresso, a widely-used framework for Android UI testing, and provide examples to demonstrate its capabilities.
Table of Contents
1. What is Espresso?
Espresso is a testing framework that allows developers to write automated UI tests for Android applications. It was developed by Google and is part of the Android Testing Support Library. Espresso’s primary goal is to make UI testing efficient, reliable, and developer-friendly. With Espresso, you can simulate user interactions and assert expected behaviors within your app’s user interface.
2. Why Use Espresso for UI Testing?
There are several reasons why Espresso is a popular choice for UI testing in Android applications:
- Simplicity: Espresso offers a straightforward and intuitive API for writing UI tests, making it accessible even to developers with minimal testing experience.
- Speed: Espresso is optimized for speed. It runs UI tests efficiently, reducing the overall testing time, and provides quick feedback on any issues.
- Integration: Espresso seamlessly integrates with Android Studio and Gradle, making it easy to set up and run tests as part of your development workflow.
- Realistic User Interaction: Espresso allows you to simulate real user interactions like clicking buttons, entering text, and scrolling, providing comprehensive test coverage.
3. Getting Started with Espresso
Before we dive into examples, you’ll need to set up Espresso in your Android project. To do this, add the following dependencies to your app’s build.gradle file:
```gradle androidTestImplementation 'androidx.test.espresso:espresso-core:<version>' androidTestImplementation 'androidx.test.espresso:espresso-contrib:<version>' ```
Replace `<version>` with the desired Espresso version.
Example 1: Testing Button Clicks
Suppose you have a simple login screen in your app with a “Login” button. You want to ensure that clicking this button triggers the correct action. Here’s how you can write an Espresso test for this scenario:
```java @Test public void testLoginButton() { // Find the "Login" button by its text onView(withText("Login")).perform(click()); // Add assertions to verify the expected behavior onView(withId(R.id.loggedInTextView)) .check(matches(isDisplayed())); } ```
In this example, `onView` is used to locate the “Login” button, and `perform(click())` simulates a button click. Then, `onView(withId(R.id.loggedInTextView))` is used to verify that the text view displaying the “logged in” message is displayed as expected.
Example 2: Testing EditText Input
Let’s say your app has a registration screen with multiple input fields. You want to verify that users can enter their information correctly. Here’s how to test EditText input using Espresso:
```java @Test public void testEditTextInput() { // Find the EditText field by its ID and type text into it onView(withId(R.id.usernameEditText)) .perform(typeText("john.doe@example.com"), closeSoftKeyboard()); // Verify that the EditText field contains the entered text onView(withId(R.id.usernameEditText)) .check(matches(withText("john.doe@example.com"))); } ```
In this example, `perform(typeText(…))` is used to type text into the EditText field, and `closeSoftKeyboard()` closes the keyboard after input. The test then checks that the entered text is displayed correctly.
Example 3: Testing RecyclerView
If your app contains a RecyclerView displaying a list of items, you can use Espresso to verify the content of the list:
```java @Test public void testRecyclerViewContent() { // Find the RecyclerView by its ID onView(withId(R.id.recyclerView)) .perform(RecyclerViewActions.scrollToPosition(3)); // Verify that an item at position 3 contains the expected text onView(withText("Item 3")) .check(matches(isDisplayed())); } ```
In this example, `perform(RecyclerViewActions.scrollToPosition(…))` scrolls the RecyclerView to a specific position, and `onView(withText(…))` checks if the expected text is displayed.
Conclusion
Espresso is a powerful tool for automating UI tests in Android applications. It simplifies the process of writing and running tests, allowing developers to catch UI-related issues early in the development cycle. By integrating Espresso into your testing strategy, you can enhance the overall quality and reliability of your Android app.
For more information and advanced topics on Espresso, refer to the official documentation
Incorporating automated UI testing into your Android development process can save time, reduce errors, and lead to a better user experience for your app’s users. So, start using Espresso today and take your Android UI testing to the next level!
To learn more about Android UI testing and stay updated on the latest developments in Android app development, be sure to check out the following external resources:
- Official Espresso Documentation– Dive deeper into Espresso by exploring the official documentation provided by Google.
- Android Testing Codelabs – Explore hands-on codelabs to sharpen your skills in Android testing, including Espresso.
- Android Developers Blog – Stay up-to-date with the latest news, best practices, and insights from the Android development team.
You can check out our other blog posts to learn more about Android. We bring you a complete guide titled Elevating Your Skills: Essential Tips and Tricks for Android Development Mastery along with the Demystifying the Android App Lifecycle: An In-depth Exploration of Activity Execution Flow and Android Security: Best Practices to Protect User Data which will help you understand and gain more insight into the Android applications.
Table of Contents
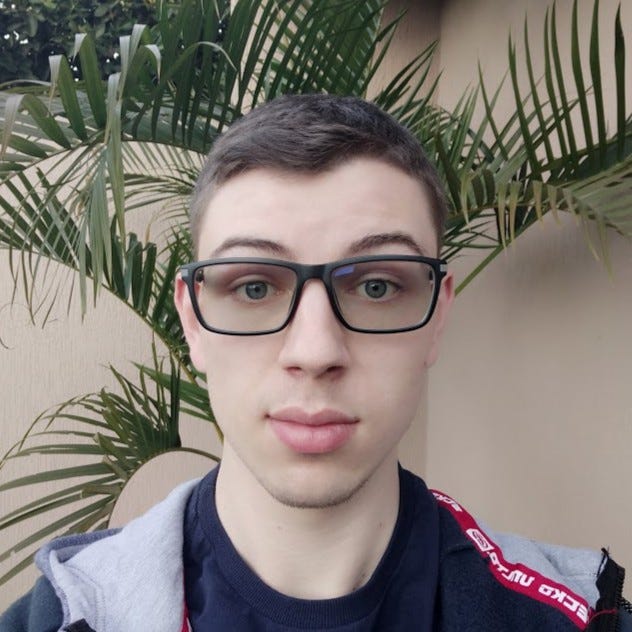
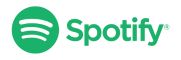