Exploring Angular Change Detection: Optimizing Performance
Angular is a popular JavaScript framework that empowers developers to build dynamic and interactive web applications. One of the core features that enables this dynamism is the change detection mechanism. However, understanding and optimizing Angular’s change detection process is crucial for building high-performance applications. In this blog post, we’ll delve deep into Angular’s change detection, explore strategies to optimize its performance, and provide code samples to illustrate these concepts.
Table of Contents
1. Understanding Angular Change Detection
Before we dive into optimization techniques, it’s essential to grasp the fundamentals of how Angular handles change detection.
1.1. What is Change Detection?
Change detection is the process by which Angular keeps track of changes in application state and updates the DOM to reflect those changes. When data in your Angular application changes, Angular needs to decide when and how to update the view to reflect those changes. This process is known as change detection.
1.1.1. Zones in Angular
Angular employs a concept called “zones” to manage change detection. Zones are execution contexts that allow Angular to intercept and track asynchronous operations, such as HTTP requests, timers, and event handlers. They play a significant role in making Angular applications reactive and responsive.
1.1.2. How Change Detection Works
Angular uses a tree of components to organize the application’s user interface. When an event or data change occurs, Angular starts from the root component and traverses down the component tree to check for changes. This process is called a “digest cycle” or “tick.”
During the digest cycle, Angular checks for changes in component properties, input bindings, and event bindings. If any changes are detected, Angular updates the DOM to reflect those changes. This process continues until no more changes are found or a specified maximum number of digest cycles is reached.
1.2. Change Detection Strategies
Now that we have a basic understanding of how Angular’s change detection works, let’s explore some strategies to optimize its performance.
1.2.1. OnPush Change Detection
Angular offers an “OnPush” change detection strategy that can significantly boost performance in your application. By default, Angular uses the “Default” change detection strategy, which checks for changes in all components during each digest cycle. However, with the “OnPush” strategy, change detection is triggered only when:
- The component’s input properties change.
- The component emits an event.
- An observable subscribed to within the component emits a new value.
To use the “OnPush” strategy, you need to set the changeDetection property in your component’s metadata:
typescript @Component({ selector: 'app-example', templateUrl: './example.component.html', changeDetection: ChangeDetectionStrategy.OnPush }) export class ExampleComponent { // ... }
This strategy reduces the number of unnecessary checks, resulting in improved performance for components that don’t frequently change.
1.2.2. Immutable Data
Immutable data is data that cannot be changed after it’s created. Using immutable data structures in your Angular application can help optimize change detection. When Angular compares objects for changes, it relies on reference checks. If an object’s reference hasn’t changed, Angular assumes that the object hasn’t changed either.
Popular libraries like Immutable.js and Immer can help you work with immutable data in your Angular application.
1.2.3. Async Pipe
The Async Pipe is a powerful tool for handling asynchronous data in Angular applications. It automatically subscribes to an observable and updates the view whenever the observable emits a new value. By using the Async Pipe, you can simplify your code and reduce the manual handling of subscriptions and unsubscribing, which can lead to memory leaks if not managed correctly.
Here’s an example of how to use the Async Pipe in a component’s template:
html <div>{{ data$ | async }}</div>
1.2.4. ChangeDetectionRef
The ChangeDetectorRef is a built-in Angular service that allows you to manually trigger change detection for a component or its children. While this approach should be used sparingly, it can be useful in situations where you need fine-grained control over when change detection occurs.
typescript import { ChangeDetectorRef } from '@angular/core'; @Component({ selector: 'app-example', templateUrl: './example.component.html' }) export class ExampleComponent { constructor(private cdr: ChangeDetectorRef) {} // Manually trigger change detection detectChanges() { this.cdr.detectChanges(); } }
1.2.5. Memoization
Memoization is a technique used to optimize function calls by caching the results of expensive operations. In an Angular context, you can apply memoization to methods that calculate or transform data. By storing the results of these methods and returning cached results when the inputs haven’t changed, you can reduce the computational overhead of change detection.
Here’s a simple example of memoization in an Angular component:
typescript export class ExampleComponent { private cachedResult: any = null; calculateExpensiveValue(input: any): any { if (this.cachedResult !== null && input === this.cachedResult.input) { return this.cachedResult.value; } // Perform expensive calculation const result = /* ... */ // Cache the result this.cachedResult = { input, value: result }; return result; } }
1.3. Detaching Change Detection
In some cases, you may want to temporarily disable change detection for a component or a section of your application. This can be useful when you know that certain parts of the DOM won’t change and don’t need to be checked during change detection.
You can use the ChangeDetectorRef service to detach and reattach change detection as needed:
typescript import { ChangeDetectorRef } from '@angular/core'; @Component({ selector: 'app-example', templateUrl: './example.component.html' }) export class ExampleComponent { constructor(private cdr: ChangeDetectorRef) {} // Detach change detection detachChangeDetection() { this.cdr.detach(); } // Reattach change detection reattachChangeDetection() { this.cdr.reattach(); } }
1.4. TrackBy Function
When rendering lists in Angular templates using *ngFor, Angular needs to track changes in the list items. By default, Angular uses object identity to track items, which can lead to unnecessary DOM updates if the list items have the same content but different object references.
To optimize this, you can provide a trackBy function to *ngFor, which tells Angular how to track changes based on a unique identifier. This can improve performance when working with large lists.
html <ul> <li *ngFor="let item of items; trackBy: trackByFn">{{ item.name }}</li> </ul> In your component, define the trackByFn function: typescript Copy code trackByFn(index: number, item: any): any { return item.id; // Use a unique identifier }
By providing a trackBy function, you ensure that Angular only updates the DOM for items that have actually changed.
2. Change Detection in Production
While optimizing change detection is crucial during development, it’s equally important to consider how your Angular application performs in a production environment. Here are some additional strategies for production:
2.1. Ahead-of-Time (AOT) Compilation
Angular applications can be compiled in two modes: Just-In-Time (JIT) and Ahead-of-Time (AOT). AOT compilation occurs before the application is served to the client, resulting in smaller bundle sizes and faster startup times. AOT-compiled applications also benefit from improved error checking during build time.
To enable AOT compilation, use the –aot flag when building your Angular application:
bash ng build --aot
2.2. Lazy Loading
Lazy loading is a technique that loads only the necessary parts of your application when they are required. This reduces the initial load time and improves overall performance, especially in large applications.
Angular provides built-in support for lazy loading modules. You can configure your routes to load modules on-demand using the loadChildren property:
typescript const routes: Routes = [ { path: 'lazy', loadChildren: () => import('./lazy.module').then(m => m.LazyModule) } ];
2.3. Tree Shaking
Tree shaking is a process that eliminates unused code from your application’s bundle. This reduces the bundle size and improves loading times. To enable tree shaking in your Angular application, ensure that you’re using Angular CLI with production optimization settings:
bash ng build --prod
2.4. Production Build Profiling
Profiling your production build can help you identify performance bottlenecks and optimize your application further. Tools like Google Chrome’s DevTools and Webpack Bundle Analyzer can provide insights into your application’s bundle size and loading times.
3. Server-Side Rendering (SSR)
Server-Side Rendering (SSR) can dramatically improve the initial load time and SEO performance of your Angular application. SSR pre-renders the initial HTML on the server and sends it to the client, reducing the client’s workload and improving the perceived performance.
Angular Universal is a framework for building server-side-rendered Angular applications.
Conclusion
Optimizing performance in Angular applications involves a deep understanding of how change detection works and implementing strategies to minimize unnecessary updates. By using the “OnPush” change detection strategy, working with immutable data, and utilizing tools like the Async Pipe and memoization, you can significantly enhance the performance of your Angular applications.
In production, consider AOT compilation, lazy loading, tree shaking, production build profiling, and server-side rendering to further improve the user experience.
Angular provides a powerful platform for building robust web applications, and by mastering its change detection system, you can ensure your applications are both performant and responsive, providing a top-notch user experience.
Start implementing these optimization techniques in your Angular projects, and watch your application’s performance soar to new heights!
Table of Contents
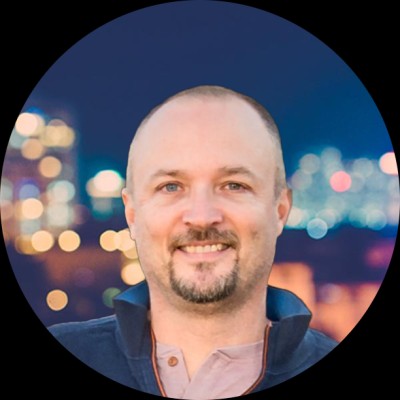
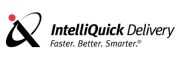