Angular Performance Optimization: Best Practices and Tools
In the fast-paced world of web development, performance optimization is crucial to providing a seamless user experience. When it comes to building web applications with Angular, a popular JavaScript framework, optimizing performance becomes even more significant. In this blog post, we’ll delve into Angular performance optimization, exploring best practices and tools that can help you boost the speed and responsiveness of your Angular applications.
Table of Contents
1. Why Angular Performance Matters
Before we dive into optimization techniques, let’s understand why Angular performance is so important. Users have high expectations for web applications; they demand speedy, responsive, and visually appealing experiences. Slow or unoptimized Angular applications can lead to increased bounce rates, lower user engagement, and a negative impact on your brand’s reputation.
Angular, like any other web framework, generates HTML, CSS, and JavaScript that the browser must interpret and render. If your application is inefficient, it can result in longer load times and decreased responsiveness. Users may abandon your app if they have to wait too long for it to load or interact sluggishly.
Optimizing your Angular application is essential for the following reasons:
- Better User Experience: Faster load times and smoother interactions make for happier users.
- SEO Benefits: Search engines favor faster websites, leading to improved search rankings.
- Lower Bounce Rates: Users are more likely to stay and explore when your app performs well.
- Cost Savings: Optimized apps consume fewer server resources and reduce hosting costs.
- Competitive Advantage: Outperforming competitors in terms of speed can set your app apart.
Now that we understand the importance of Angular performance, let’s explore some best practices and tools to achieve it.
2. Best Practices for Angular Performance Optimization
2.1. Lazy Loading Modules
Lazy loading modules is a fundamental technique for Angular performance optimization. By loading modules only when they are needed, you can reduce the initial load time of your application. Angular’s built-in lazy loading feature allows you to split your application into smaller, manageable chunks that load on-demand.
Here’s how to set up lazy loading in your Angular application:
typescript const routes: Routes = [ { path: 'home', loadChildren: () => import('./home/home.module').then(m => m.HomeModule) }, { path: 'about', loadChildren: () => import('./about/about.module').then(m => m.AboutModule) }, // ... ];
By using the loadChildren property in your route configuration, you can specify which modules to load lazily. This reduces the initial bundle size, resulting in faster load times.
2.2. Ahead-of-Time (AOT) Compilation
Angular applications can be compiled in two ways: Just-in-Time (JIT) and Ahead-of-Time (AOT). AOT compilation is the recommended approach for production applications. It compiles your Angular code during the build process, resulting in smaller and faster-loading JavaScript bundles.
To enable AOT compilation, use the –aot flag when building your Angular app:
bash ng build --aot
AOT compilation eliminates the need for the Angular compiler to run in the browser, reducing startup time and improving overall performance.
2.3. Tree Shaking
Tree shaking is a technique used to eliminate unused code from your application bundles. Angular CLI uses the underlying tool, Terser, to perform tree shaking during the build process automatically.
However, it’s essential to ensure that your code is written in a way that enables effective tree shaking. Avoid importing entire modules when you only need specific components or functions. Instead, import only what you use:
typescript // Bad: Importing the entire module import * as moment from 'moment'; // Good: Importing only what you need import { format } from 'date-fns';
Effective tree shaking can significantly reduce the size of your bundles, resulting in faster load times.
2.4. Change Detection Optimization
Angular’s change detection mechanism is powerful but can also be a performance bottleneck if not used carefully. You can optimize change detection by following these tips:
2.4.1. OnPush Change Detection Strategy
By default, Angular uses the default change detection strategy, which checks all components for changes on every tick. However, you can change the change detection strategy for specific components to OnPush. This strategy tells Angular to only check for changes when the component’s input properties change or when you explicitly trigger change detection.
typescript @Component({ selector: 'app-example', templateUrl: './example.component.html', changeDetection: ChangeDetectionStrategy.OnPush, }) export class ExampleComponent { // ... }
Using the OnPush strategy can significantly reduce the number of change detection cycles, improving performance.
2.4.2. Immutable Data and Pure Pipes
Using immutable data structures and pure pipes helps Angular’s change detection system understand when data has changed. When your application uses immutable data and pure pipes, Angular can skip change detection for components that haven’t been affected by the change.
Ensure that your data structures are immutable and use pure pipes for filtering and formatting data.
2.5. Production Builds and Minification
When building your Angular application for production, use the –prod flag along with optimization flags to enable minification, ahead-of-time compilation, and other production-specific optimizations:
bash ng build --prod
Production builds are optimized for performance, resulting in smaller bundle sizes and faster load times. These builds also remove debugging code and enable Angular’s production mode, which disables certain runtime checks.
2.6. Lazy-Load Images and Assets
Lazy-loading images and assets can significantly improve the initial load time of your Angular application. Instead of loading all images and assets when the page loads, load them on-demand as the user scrolls or interacts with your application.
You can achieve lazy loading of images using libraries like ng-lazyload-image or by implementing your custom lazy loading logic.
2.7. Web Workers
Web Workers allow you to run JavaScript code in the background, separate from the main application thread. This can be used for tasks like data fetching, image processing, and other CPU-intensive operations. By offloading work to Web Workers, you can keep the main thread responsive, resulting in a smoother user experience.
Angular provides a way to use Web Workers with the @angular/service-worker package, which can be integrated into your application to handle background tasks.
3. Tools for Angular Performance Profiling
In addition to best practices, there are several tools available to help you profile and optimize the performance of your Angular application. Let’s explore some of the most useful ones:
3.1. Angular CLI
Angular CLI provides various commands and flags to analyze and optimize your application’s performance. You can use the following commands:
- ng build –prod: Builds a production-ready bundle with optimizations.
- ng serve –prod: Runs the development server with production settings for better performance analysis.
- ng lint: Checks your code for linting issues that can impact performance.
- ng test: Runs unit tests to ensure your application functions correctly and efficiently.
3.2. Lighthouse
Lighthouse is an open-source tool from Google that audits web applications for performance, accessibility, SEO, and more. You can run Lighthouse directly from the Chrome DevTools or as a standalone CLI tool. It provides a detailed report with actionable recommendations to improve your application’s performance.
3.3. Webpack Bundle Analyzer
Webpack Bundle Analyzer is a useful tool for visualizing the size of your application bundles. It generates a visual representation of your bundle’s contents, making it easy to identify large dependencies or unnecessary code. By analyzing your bundles, you can pinpoint areas for optimization and reduce bundle size.
To use Webpack Bundle Analyzer, you can add it as a plugin to your Angular project and run it during the build process.
3.4. Augury
Augury is a Chrome DevTools extension specifically designed for debugging and profiling Angular applications. It provides insights into your component tree, change detection, and routing, helping you identify performance bottlenecks and memory leaks. Augury is a valuable tool for both development and debugging in Angular projects.
3.5. Chrome DevTools
Chrome DevTools is a powerful set of web development tools built into the Chrome browser. It includes a range of features for profiling and debugging web applications. You can use the Performance tab to record and analyze performance data, inspect network requests, and diagnose rendering issues. Chrome DevTools is indispensable for identifying and resolving performance problems in your Angular app.
Conclusion
Angular performance optimization is an ongoing process that requires a combination of best practices and tools. By implementing lazy loading, AOT compilation, tree shaking, and other optimization techniques, you can create faster and more responsive Angular applications. Additionally, using performance profiling tools like Lighthouse, Webpack Bundle Analyzer, and Augury can help you identify and address performance issues effectively.
Remember that optimizing for performance is not a one-time task; it’s a continuous effort to ensure your Angular application delivers the best possible user experience. Stay up to date with the latest Angular updates and best practices, and regularly audit and profile your app to keep it running at peak performance. Your users will thank you with increased engagement and loyalty.
Are you ready to supercharge your Angular application’s performance? Start implementing these best practices and using the recommended tools today, and watch your app soar to new heights of speed and responsiveness.
Table of Contents
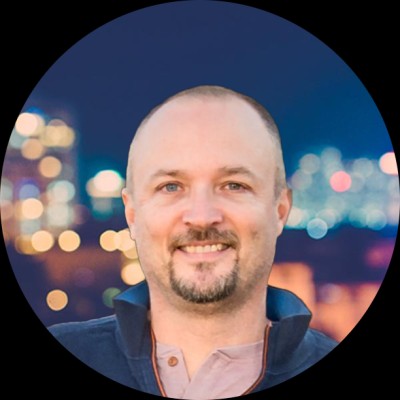
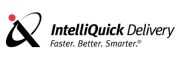