Building Progressive Web Apps with Angular: Offline Capabilities
In today’s digital age, users expect web applications to be not only responsive and fast but also available even when there’s no internet connection. Progressive Web Apps (PWAs) have emerged as a solution to meet these expectations, offering offline capabilities that provide a seamless user experience. In this blog, we will explore how to build PWAs with Angular and enhance them with offline capabilities.
Table of Contents
1. What Are Progressive Web Apps (PWAs)?
Progressive Web Apps (PWAs) are web applications that leverage modern web technologies to deliver a native app-like experience on the web. They combine the best of both worlds: the reach of the web and the performance of native apps. PWAs can be accessed through a web browser but offer features typically associated with native applications, such as offline access, push notifications, and the ability to install on a user’s device.
2. Advantages of PWAs
Before diving into the technical aspects of building PWAs with Angular, let’s briefly explore the advantages they offer:
2.1. Cross-Platform Compatibility
PWAs work on various platforms and devices, including desktops, smartphones, and tablets. This cross-platform compatibility allows you to reach a wider audience with a single codebase.
2.2. Offline Access
One of the key features of PWAs is the ability to work offline. Users can still use the app and access cached content even when there is no internet connection. This ensures a consistent user experience regardless of connectivity.
2.3. Improved Performance
PWAs are designed to be fast and responsive, providing a smooth user experience. They load quickly, respond to user interactions promptly, and offer a fluid navigation experience.
2.4. Installation-Free
PWAs can be added to the user’s home screen without the need for an app store. This frictionless installation process encourages users to engage with your app.
2.5. Push Notifications
PWAs support push notifications, allowing you to re-engage users and send updates or promotions directly to their devices.
Now that we understand the benefits of PWAs, let’s move on to building one with Angular and adding offline capabilities.
3. Building a PWA with Angular
Angular is a popular JavaScript framework for building web applications. It provides a robust foundation for creating PWAs, thanks to its comprehensive set of tools and features. Here’s how you can start building a PWA with Angular:
3.1. Set Up an Angular Project
If you haven’t already, you’ll need to set up an Angular project. You can do this using the Angular CLI (Command Line Interface), which simplifies project creation and management. Open your terminal and run the following commands:
bash npm install -g @angular/cli ng new my-pwa cd my-pwa
This will create a new Angular project named “my-pwa.”
3.2. Configure the Angular Service Worker
To enable offline capabilities in your PWA, you’ll need to configure the Angular service worker. The service worker is a script that runs in the background and intercepts network requests, allowing you to cache assets and data for offline use.
Angular CLI provides built-in support for adding a service worker to your project. Run the following command to generate and set up the service worker:
bash ng add @angular/pwa
This command will add the necessary files and configuration for the service worker.
3.3. Define the App Manifest
The app manifest is a JSON file that provides metadata about your PWA, such as its name, icons, and display mode. Create a file named manifest.webmanifest in the src folder of your project and add the following content:
json { "name": "My PWA", "short_name": "PWA", "start_url": "/", "display": "standalone", "background_color": "#ffffff", "theme_color": "#000000", "icons": [ { "src": "assets/icons/icon-192x192.png", "sizes": "192x192", "type": "image/png" }, { "src": "assets/icons/icon-512x512.png", "sizes": "512x512", "type": "image/png" } ] }
This manifest file provides essential information about your PWA and how it should behave when installed on a user’s device.
3.4. Add Offline Support
Now that you have set up the service worker and defined the app manifest, it’s time to add offline support to your Angular app. This involves caching the app’s assets and data so that they can be accessed even when the user is offline.
In your Angular project, open the ngsw-config.json file, which is generated by the Angular CLI when you added the service worker. This file allows you to configure caching strategies for different types of assets. Here’s an example configuration that caches the app shell and API requests:
json { "index": "/index.html", "assetGroups": [ { "name": "app", "installMode": "prefetch", "resources": { "files": ["/favicon.ico", "/index.html", "/*.css", "/*.js"] } }, { "name": "api", "installMode": "lazy", "updateMode": "prefetch", "resources": { "patterns": ["/api/**"] } } ] }
In this example, the “app” asset group includes essential files like index.html, CSS, and JavaScript files, while the “api” group caches API requests. You can customize this configuration to suit your app’s specific needs.
3.5. Test Offline Functionality
To test the offline functionality of your PWA, you can simulate an offline environment using your browser’s developer tools. Open the Chrome DevTools, go to the “Application” tab, and check the “Offline” option. This will disconnect your app from the network, allowing you to see how it behaves in an offline state.
Try accessing different parts of your app to ensure that the cached assets and data are used when there’s no internet connection. This is a crucial step in verifying that your PWA works as expected offline.
4. Handling Data Synchronization
While caching assets and static data is a significant step toward building an offline-capable PWA, many web applications also require data synchronization with a server when the connection is re-established. Here’s how you can handle data synchronization in your Angular PWA:
4.1. Use a Service Worker for Background Sync
Service workers can be used to perform background sync operations when the app is online. For example, you can queue user actions or data updates and then synchronize them with the server when the connection is available again.
To implement background sync, you can create a custom service worker script and use the Background Sync API. Here’s a simplified example:
javascript // custom-service-worker.js self.addEventListener('sync', (event) => { if (event.tag === 'sync-data') { event.waitUntil(syncDataWithServer()); } }); function syncDataWithServer() { // Perform data synchronization with the server here }
In your Angular app, register this custom service worker in the ngsw-config.json file:
json { "index": "/index.html", "serviceWorker": "./custom-service-worker.js", // ... }
Now, when the app is online, the service worker will listen for sync events and trigger the synchronization process.
4.2. Handle Failed Synchronization
Synchronization with the server may fail due to various reasons, such as server unavailability or network issues. It’s essential to handle these cases gracefully and provide feedback to the user.
In your custom service worker, you can capture synchronization errors and store them locally. When the user goes online again, you can attempt to re-synchronize the failed data. Additionally, you can notify the user about the failed synchronization and offer options for retrying the operation.
5. Providing a Seamless User Experience
To provide a seamless user experience in your Angular PWA, consider implementing the following strategies:
5.1. Graceful Degradation
Design your app to gracefully degrade when offline. Instead of displaying error messages, provide users with cached content or placeholder data when network requests fail.
5.2. Offline UI
Create a user-friendly offline UI that informs users of their offline status and offers guidance on what they can still do in the app. For example, you can display a message like “You are offline. Some features may be limited.”
5.3. Background Sync Indicator
Include an indicator or notification in your app to inform users when background synchronization is in progress. This helps users understand that their data is being synchronized in the background.
5.4. Offline-First Design
Adopt an “offline-first” design approach, where the app’s core functionality is available offline, and online features are considered enhancements. This ensures that users can always use essential app features.
6. Testing and Debugging
Testing and debugging are crucial aspects of building offline-capable PWAs. Here are some tools and techniques you can use:
6.1. Browser DevTools
As mentioned earlier, browser developer tools allow you to simulate offline conditions and test how your PWA behaves in such scenarios. Use the “Service Workers” tab to inspect service worker registration and caching.
6.2. Lighthouse Audits
Google’s Lighthouse tool can perform audits on your PWA to evaluate its offline capabilities, performance, and accessibility. It provides actionable recommendations for improvement.
6.3. Workbox DevTools
If you’re using Workbox, a library for service worker management, it offers DevTools extensions that simplify debugging and monitoring of your service worker.
Conclusion
Building Progressive Web Apps (PWAs) with Angular and adding offline capabilities is a powerful way to provide a seamless user experience. By following the steps outlined in this guide, you can create web applications that work reliably even when there’s no internet connection.
Remember that offline support is just one aspect of building a great PWA. Ensuring performance, responsiveness, and user engagement are equally important. PWAs have the potential to reach a broader audience and keep users engaged, making them a valuable addition to your web development toolkit.
Incorporate offline capabilities into your Angular PWAs, test thoroughly, and continuously improve the user experience to deliver web applications that users will love, whether online or offline.
Table of Contents
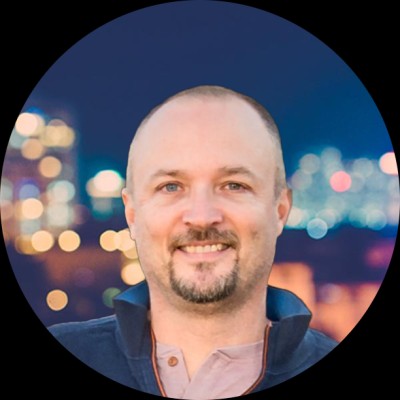
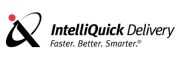