Exploring Angular Custom Directives: Extending Component Functionality
Angular, a powerful front-end framework, provides developers with a wide range of tools and features to build dynamic and interactive web applications. One such feature that often proves invaluable in enhancing the functionality of Angular components is custom directives. In this blog post, we’ll explore Angular custom directives, their significance, and how they can be used to extend the capabilities of your components.
Table of Contents
1. Understanding Directives in Angular
Before diving into custom directives, let’s grasp the concept of directives in Angular itself. Directives are a category of Angular components that empower you to create, manipulate, and interact with the DOM (Document Object Model). They are used to extend the functionality of HTML elements, allowing you to attach custom behavior to elements or even create entirely new elements.
Angular provides several built-in directives like ngIf, ngFor, and ngStyle. These directives are widely used to handle common tasks, such as conditional rendering, iterating over lists, and dynamically applying styles to elements. While these built-in directives are incredibly useful, there are situations where custom directives are necessary to meet specific project requirements.
2. Why Custom Directives?
Custom directives offer a way to encapsulate and reuse code that deals with DOM manipulation or event handling, making your application more maintainable and readable. They can help in achieving the following benefits:
2.1. Reusability
Custom directives allow you to package a specific set of behaviors or functionalities into a reusable component. Once defined, you can apply the same behavior to multiple elements across your application, reducing code duplication and improving consistency.
2.2. Separation of Concerns
Custom directives promote the separation of concerns by isolating DOM manipulation logic from your component’s business logic. This separation makes your codebase cleaner and more maintainable, as each component focuses on its specific responsibilities.
2.3. Readability
By encapsulating complex DOM interactions within custom directives, your component code becomes cleaner and more readable. This makes it easier for other developers to understand and work with your code.
2.4. Testing
Custom directives can be tested independently, ensuring that the behavior you’ve encapsulated in them functions correctly. This makes it easier to identify and fix issues in your application.
2.5. Adaptability
Custom directives are versatile and can be applied to a wide range of scenarios. They provide a flexible way to add functionality to elements without modifying the underlying component.
Now that we understand the benefits of custom directives, let’s explore how to create and use them in Angular.
3. Creating Custom Directives in Angular
Creating a custom directive in Angular involves several steps, including the following:
3.1. Directive Definition
To define a custom directive, you must use the @Directive decorator. This decorator tells Angular that a class is a directive and provides metadata about the directive’s selector and other properties. Here’s an example:
typescript import { Directive, ElementRef } from '@angular/core'; @Directive({ selector: '[appHighlight]' }) export class HighlightDirective { constructor(private el: ElementRef) { el.nativeElement.style.backgroundColor = 'yellow'; } }
In this example, we’ve created a HighlightDirective that changes the background color of an element to yellow. The @Directive decorator specifies that this directive can be applied using the [appHighlight] selector.
3.2. Directive Logic
The logic of your custom directive resides within its class. In our example, the logic is found in the constructor, where we use the ElementRef to access the DOM element and change its background color to yellow.
3.3. Applying the Directive
To use the custom directive in your templates, simply include its selector as an attribute on the HTML element you want to apply the directive to. Here’s how you can use our appHighlight directive:
html <div appHighlight> Highlight me! </div>
When this directive is applied to the div element, it will change the background color to yellow.
3.4. Module Declaration
Don’t forget to declare your custom directive in an Angular module’s declarations array. This step is essential for Angular to recognize and use your custom directive within the module.
typescript @NgModule({ declarations: [ // ... HighlightDirective ], // ... }) export class AppModule { }
With these steps completed, your custom directive is ready to be used in your Angular application.
4. Use Cases for Custom Directives
Custom directives can be applied in various scenarios to enhance your Angular application’s functionality. Let’s explore some common use cases where custom directives shine:
4.1. Input Validation
You can create custom directives to enforce input validation rules on form fields. For instance, you can create a directive that ensures a password input meets specific complexity requirements or restricts input to certain characters.
4.2. Highlighting and Animation
Custom directives are excellent for creating animations or highlighting elements on user interaction. For example, you can create a directive that adds a shimmer effect to an element when the user hovers over it.
4.3. Lazy Loading
Custom directives can be used to lazy load content or images when they become visible in the viewport. This is particularly useful for optimizing performance on pages with large amounts of content.
4.4. Responsive Design
You can create custom directives to conditionally show or hide elements based on screen size or other conditions. This helps in building responsive web applications that adapt to different devices and screen sizes.
4.5. Access Control
Custom directives can enforce access control rules in your application. For example, you can create a directive that hides or disables certain UI elements based on the user’s permissions.
4.6. Third-Party Integration
When integrating third-party libraries or widgets into your Angular application, custom directives can provide an abstraction layer that simplifies the integration process and keeps your codebase clean.
5. Advanced Custom Directives
Custom directives can be as simple or as complex as your application requires. While we’ve covered the basics, there are more advanced concepts and techniques you can explore:
5.1. Input and Output Properties
Custom directives can have input and output properties, allowing them to communicate with parent components and other directives. Input properties enable you to pass data into the directive, while output properties allow the directive to emit events.
typescript @Input() appCustomInput: string; @Output() appCustomOutput: EventEmitter<string> = new EventEmitter();
5.2. Host Listeners and Host Bindings
You can use @HostListener and @HostBinding decorators to listen for events on the host element or bind to its properties. This is especially useful when your directive needs to respond to user interactions or update the host element’s appearance.
typescript @HostListener('mouseenter') onMouseEnter() { // Handle mouse enter event } @HostBinding('style.backgroundColor') backgroundColor: string;
5.3. Directive Lifecycle Hooks
Custom directives can implement lifecycle hooks, just like Angular components. These hooks allow you to execute code at various points in the directive’s lifecycle, such as ngOnInit for initialization or ngOnChanges for reacting to input changes.
typescript ngOnInit() { // Initialize the directive } ngOnChanges(changes: SimpleChanges) { // React to input changes }
6. Real-World Example: Creating a Tooltip Directive
To demonstrate the power of custom directives, let’s create a simple tooltip directive that displays a tooltip when the user hovers over an element. This example will illustrate how custom directives can encapsulate complex behavior in a reusable package.
6.1. Directive Definition
Let’s start by defining our tooltip directive:
typescript import { Directive, Input, ElementRef, Renderer2, HostListener } from '@angular/core'; @Directive({ selector: '[appTooltip]' }) export class TooltipDirective { @Input() appTooltip: string; private tooltipElement: HTMLElement; constructor(private el: ElementRef, private renderer: Renderer2) { this.tooltipElement = this.renderer.createElement('div'); this.renderer.addClass(this.tooltipElement, 'tooltip'); } @HostListener('mouseenter') onMouseEnter() { this.tooltipElement.textContent = this.appTooltip; this.renderer.appendChild(this.el.nativeElement, this.tooltipElement); } @HostListener('mouseleave') onMouseLeave() { this.renderer.removeChild(this.el.nativeElement, this.tooltipElement); } }
In this directive, we create a TooltipDirective with a selector [appTooltip]. It listens for mouseenter and mouseleave events to show and hide the tooltip. We also define an input property appTooltip to set the tooltip content.
6.2. Styling
You can style the tooltip using CSS. Here’s a simple example:
css .tooltip { position: absolute; background-color: #333; color: #fff; padding: 5px; border-radius: 4px; z-index: 1; top: 100%; left: 50%; transform: translateX(-50%); display: none; } :host(:hover) .tooltip { display: block; }
6.3. Using the Tooltip Directive
Now, let’s see how we can use our appTooltip directive in our templates:
html <button [appTooltip]="'Click me!'">Hover me</button>
When the user hovers over the button, a tooltip with the text “Click me!” will be displayed.
Conclusion
Angular custom directives are a powerful tool for extending the functionality of your components and enhancing your application’s overall user experience. They promote code reusability, maintainability, and readability while providing a flexible way to encapsulate complex behaviors.
As you continue to explore Angular custom directives, you’ll discover endless possibilities for creating interactive and dynamic web applications. Whether you’re building simple tooltips or complex UI components, custom directives are a valuable addition to your Angular development toolkit.
So, the next time you encounter a scenario in your Angular project where you need to enhance an element’s behavior or appearance, consider creating a custom directive to simplify your code and make it more maintainable. With custom directives, you’ll be well-equipped to tackle a wide range of challenges in your Angular applications. Happy coding!
Table of Contents
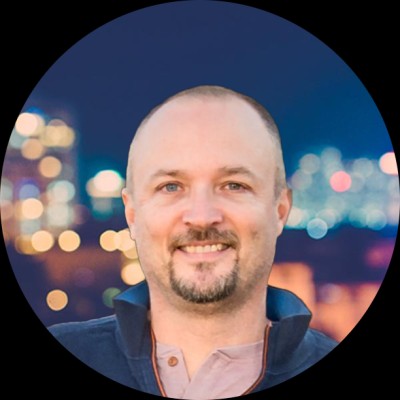
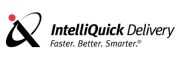