Exploring Angular Directives: Building Custom UI Components
Angular, a powerful JavaScript framework, offers a multitude of tools and features to simplify web development. One of its standout features is directives. Directives are a way to extend HTML by creating custom, reusable elements or attributes that can add functionality and interactivity to your web applications. In this blog, we will delve deep into the world of Angular directives, with a focus on building custom UI components. By the end of this journey, you will have the knowledge and skills to create your own custom directives and enhance your web development projects.
Table of Contents
1. Understanding Directives
Before we dive into building custom UI components, let’s establish a fundamental understanding of what Angular directives are and how they work.
1.1. What Are Angular Directives?
Angular directives are special markers on DOM elements (such as attributes, element names, comments, or CSS classes) that Angular uses to extend the functionality of HTML. They provide a way to manipulate the DOM, add behavior to elements, and interact with data in your Angular application.
Angular comes with a set of built-in directives, such as ngIf, ngFor, and ngModel, which are widely used for common tasks like conditionally rendering elements, iterating over collections, and binding data to form controls. However, you can also create your own custom directives to tailor your application’s behavior to your specific needs.
1.2. Types of Directives
Angular directives are categorized into four main types:
- Component Directives: These are the most common type of directives in Angular. They represent components with templates and logic that can be used as custom HTML elements. Component directives are an essential part of building custom UI components, and we’ll explore them in detail.
- Attribute Directives: Attribute directives modify the appearance or behavior of an element. They are typically used as HTML attributes and often serve as decorators for existing elements. An example is Angular’s ngStyle directive, which allows you to set inline styles based on conditions.
- Structural Directives: Structural directives modify the structure of the DOM by adding or removing elements. ngIf and ngFor are prime examples. They enable you to conditionally include or repeat elements in your templates.
- Custom Directives: As the name suggests, custom directives are created by developers to add unique functionality to their applications. These can be attribute directives, structural directives, or even component directives, depending on the desired behavior.
2. Building Custom UI Components
Now that we have a grasp of what directives are, let’s focus on building custom UI components using Angular’s component directives. Custom UI components are a powerful way to encapsulate and reuse complex parts of your application’s user interface. Whether it’s a custom date picker, a dynamic chart, or a specialized input field, creating custom UI components with Angular is a breeze.
2.1. Setting Up Your Angular Environment
Before we start creating custom UI components, ensure that you have Node.js and Angular CLI installed on your machine. If you haven’t already, you can install Angular CLI globally using the following command:
bash npm install -g @angular/cli
Once Angular CLI is installed, you can create a new Angular project using the ng new command:
bash ng new custom-components-app
Navigate to your project folder:
bash cd custom-components-app
Now, you’re ready to start building custom UI components!
3. Creating Your First Custom UI Component
Let’s create a simple custom UI component called app-button. This component will represent a customizable button with different colors and text.
Step 1: Generate a New Component
To generate a new component, use the following Angular CLI command:
bash ng generate component button
This command will create a folder named button in your project directory with the necessary files for the component.
Step 2: Define the Component Logic
Open the button.component.ts file in the button folder. This is where you’ll define the logic for your custom button component.
typescript import { Component, Input } from '@angular/core'; @Component({ selector: 'app-button', templateUrl: './button.component.html', styleUrls: ['./button.component.css'] }) export class ButtonComponent { @Input() text: string = 'Click me'; @Input() color: string = 'primary'; }
In this code, we’ve defined a component called ButtonComponent. It has two input properties: text and color. The text property will determine the text displayed on the button, while the color property will determine the button’s color.
Step 3: Create the Component Template
Next, open the button.component.html file in the same folder and define the template for your custom button component:
html <button [ngClass]="color">{{ text }}</button>
In this template, we’re using the ngClass directive to dynamically apply a CSS class based on the color input property. This allows us to style the button with different colors.
Step 4: Styling the Component
You can add some basic styles to your button component by modifying the button.component.css file:
css .primary { background-color: #007bff; color: white; border: none; } .secondary { background-color: #6c757d; color: white; border: none; } .success { background-color: #28a745; color: white; border: none; }
In this example, we’ve defined styles for three different colors: primary, secondary, and success.
Step 5: Using the Custom Button Component
Now that you’ve created your custom button component, you can use it in your application’s templates. Open the app.component.html file and add the following code:
html <app-button text="Primary Button" color="primary"></app-button> <app-button text="Secondary Button" color="secondary"></app-button> <app-button text="Success Button" color="success"></app-button>
Here, we’re using the app-button component and passing different values for the text and color inputs. This will render three custom buttons with varying styles.
Step 6: Serve Your Application
To see your custom button component in action, serve your Angular application using the following command:
bash ng serve
Visit http://localhost:4200 in your web browser to view the application. You’ll see the custom buttons you created displayed on the page with their respective styles.
4. Adding Functionality to Custom UI Components
While creating custom UI components is a great start, you can enhance them by adding interactivity and functionality. Let’s expand on our app-button component by making it clickable and emitting an event when clicked.
Step 7: Adding Click Functionality
In the button.component.ts file, modify the component logic as follows:
typescript import { Component, Input, Output, EventEmitter } from '@angular/core'; @Component({ selector: 'app-button', templateUrl: './button.component.html', styleUrls: ['./button.component.css'] }) export class ButtonComponent { @Input() text: string = 'Click me'; @Input() color: string = 'primary'; @Output() buttonClick = new EventEmitter<void>(); onClick() { this.buttonClick.emit(); } }
In this updated code, we’ve added an @Output property named buttonClick, which is an EventEmitter. We also added an onClick method that emits the buttonClick event when the button is clicked.
Step 8: Emitting the Click Event
In the button.component.html file, update the button element to include a click event handler:
html <button [ngClass]="color" (click)="onClick()">{{ text }}</button>
Now, when the button is clicked, it will trigger the onClick method, which emits the buttonClick event.
Step 9: Handling the Click Event
In your app.component.html file, you can now handle the click event emitted by the custom button component:
html <app-button text="Primary Button" color="primary" (buttonClick)="onPrimaryButtonClick()"></app-button> <app-button text="Secondary Button" color="secondary" (buttonClick)="onSecondaryButtonClick()"></app-button> <app-button text="Success Button" color="success" (buttonClick)="onSuccessButtonClick()"></app-button>
In this code, we’ve added (buttonClick) event bindings to each custom button component and provided corresponding event handler methods, such as onPrimaryButtonClick, onSecondaryButtonClick, and onSuccessButtonClick, in your app.component.ts file.
Step 10: Implement Event Handlers
Finally, implement the event handler methods in your app.component.ts file to handle the click events:
typescript import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { onPrimaryButtonClick() { alert('Primary button clicked!'); } onSecondaryButtonClick() { alert('Secondary button clicked!'); } onSuccessButtonClick() { alert('Success button clicked!'); } }
Now, when you click each custom button, you’ll see an alert with the corresponding message.
Conclusion
In this blog, we’ve explored the world of Angular directives with a focus on building custom UI components. We began by understanding the basics of directives, including the various types and their roles in Angular applications.
We then dived into the practical aspect of building custom UI components using Angular’s component directives. You learned how to set up your Angular environment, create a custom button component, style it, and add functionality to it. By the end of this journey, you should feel confident in creating your own custom UI components to enhance the interactivity and user experience of your web applications.
Angular directives, including custom UI components, open up a world of possibilities for web developers. They allow you to create reusable, encapsulated pieces of functionality that can be easily integrated into your projects, making your code more modular and maintainable. As you continue to explore Angular, consider extending your knowledge by creating more complex custom UI components and integrating them into real-world applications. Happy coding!
Table of Contents
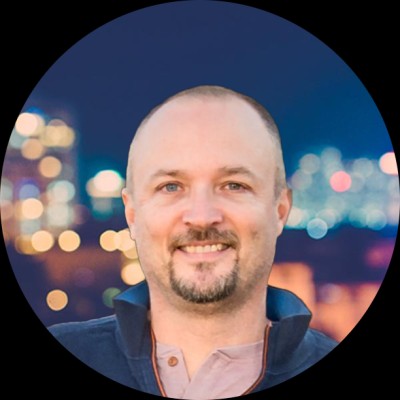
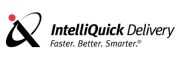